My game over function thrwos an error though. I have attached a screenshot of the error an my code. the game should be over when the head of the snake collides with the rest of the snake. Can you help fixing it?
Hi there,
I am making a snake game in python. My game over function thrwos an error though. I have attached a screenshot of the error an my code. the game should be over when the head of the snake collides with the rest of the snake. Can you help fixing it? also where exactly should i call the game_over function, is it it correct in my code?
thanks you so much in advance
my code:
import snakelib
from snakelib import SnakeUserInterface
import importlib
width = 0 # initialized in play_snake
height = 0 # initialized in play_snake
ui = None # initialized in play_snake
SPEED = 1
direction = "r"
keep_running = True
#WIDTH = 10
#HEIGHT = 10
#SCALE = 0.75
#ui = SnakeUserInterface(WIDTH,HEIGHT,SCALE)
#ui.set_animation_speed(5)
class Coordinate:
def __init__(self,x,y):
self.x = x
self.y = y
def equals(self, coordinate):
if self.x == coordinate.x and self.y == coordinate.y:
return True
def shift(self,x_shift,y_shift):
self.x += x_shift
self.y += y_shift
def copy(self):
return Coordinate(self.x, self.y)
class CoordinateSnake:
def __init__(self):
self.coordinate_row = [] # (0, 2), (0, 1), (0, 0)
def add(self, coordinate):
self.coordinate_row.append(coordinate)
def addFront(self, coordinate):
self.coordinate_row.insert(0, coordinate)
def removeTail(self):
self.coordinate_row.pop(-1)
def contains(self, coordinate):
for el in self.coordinate_row:
if coordinate.equals(el):
return True
return False
head = Coordinate(1,0)
tail = Coordinate(0,0)
body = CoordinateSnake()
body.addFront(tail)
body.addFront(head)
apple = Coordinate(5,5)
def draw():
global head, tail, body, apple, CoordinateSnake
ui.clear()
for coord in body.coordinate_row:
ui.place(coord.x, coord.y, ui.SNAKE)
ui.place(apple.x,apple.y,ui.FOOD)
ui.show()
def generate_apple():
global apple, CoordinateSnake, Coordinate
nrFreeSpots = (width * height) - len(body.coordinate_row)
apple_spot = ui.random(nrFreeSpots)
i = 0
for y in range(height):
for x in range(width):
if not body.contains(Coordinate(x,y)):
#print(i, x, y)
if i == apple_spot:
apple = Coordinate(x,y)
return
i = i + 1
def process_event(event):
if event.name == 'alarm' and event.data == "refresh":
process_alarm()
draw()
elif event.name == "arrow":
process_arrow(event.data)
def process_arrow(data):
global direction
if data == "l" and direction != "r":
direction = "l"
elif data == "r" and direction != "l":
direction = "r"
elif data == 'u' and direction != "d":
direction = "u"
elif data == 'd' and direction != "u":
direction = "d"
def process_alarm():
global head,body,apple
move()
#game_over()
if head.x == width:
head.x = 0
if head.x < 0:
head.x = width - 1
if head.y == height:
head.y = 0
if head.y < 0:
head.y = height -1
game_over() #might be needed here instead. Depends on the tests
CoordinateSnake.addFront(body,head)
if apple.x == head.x and apple.y == head.y:
generate_apple()
else:
CoordinateSnake.removeTail(body)
game_over()
def move():
global direction, head
head = Coordinate.copy(head)
if direction == "r":
Coordinate.shift(head,1,0)
if direction == "l":
Coordinate.shift(head,-1,0)
if direction == "u":
Coordinate.shift(head,0,-1)
if direction == "d":
Coordinate.shift(head,0,1)
#draw()
def game_over():
global head, tail
#tmp_head = body.coordinate_row[0]
#tmp_body = body.coordinate_row[1:]
tmp_head = body.coordinate_row[-1]
tmp_body = body.coordinate_row[:-1]
for l in tmp_body:
if tmp_head.equals(l):
ui.set_game_over()
ui.show
return
def play_snake(init_ui):
global width, height, ui, keep_running
ui = init_ui
width, height = ui.board_size()
generate_apple()
draw()
while keep_running:
event = ui.get_event()
process_event(event)
# make sure you handle the quit event like below,
# or the test might get stuck in an infinite loop
if event.name == "quit":
keep_running = False
if __name__ == "__main__":
# do this if running this module directly
# (not when importing it for the tests)
ui = snakelib.SnakeUserInterface(10, 10)
ui.set_animation_speed(SPEED)
play_snake(ui)


Step by step
Solved in 3 steps with 2 images

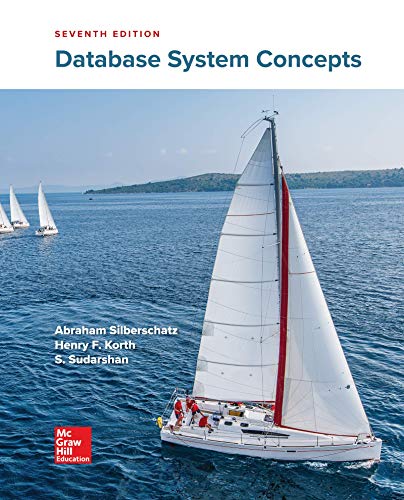
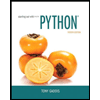
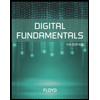
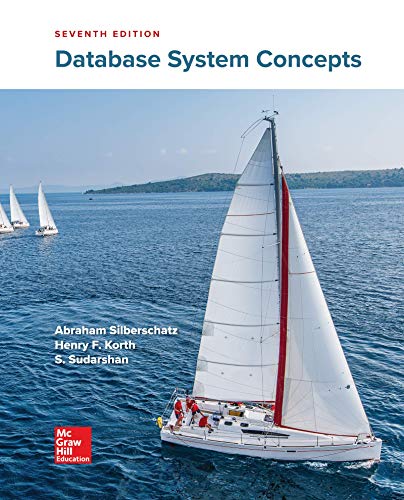
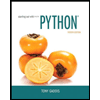
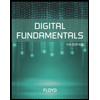
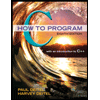
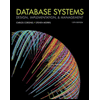
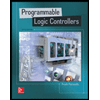