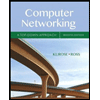
SET-252 – C Programming #2 Homework: 4 – Polymorphism
Step 1 – Parent Class • Create a CAnimal class. • Add the following protected properties: m_strName, m_strType • Add the following public methods: Get/Set Name, Get/Set Type, virtual MakeNoise.
Step 2 – Child Classes • Create three or more child classes based on the CAnimal class (e.g. CDog, CCow, CDragon). • Each child class must re-define the MakeNoise method. • Each child class must have at least one unique property (e.g. Breed, Color, AmountOfGold). • Each child class must have at least one unique method (e.g. Fetch, Graze, BreathFire). • Create at least one instance for each child class and call the set methods in each one to populate/initialize them.
Step 3 – Polymorphism – Part 1 • Create an array of CAnimal* called paclsZoo of at least size 5. • Populate the zoo with pointers to the child class instances created in the previous step. You’ll need to explicit cast the pointers/addresses. • Set at least one cell in the array to 0/null.
Step 4 – Polymorphism – Part 2 • Write a FOR loop that will loop through the zoo and for each non-null cell print the animal name, type and call the MakeNoise method. Make sure that the correct child-specific information is displayed.
Step 5 – Polymorphism – Part 3 • Write a FOR loop that will loop through the zoo and for each non-null cell explicitly cast the pointer into the child class specific type and call the unique child class method. You’ll need to string compare the animal type in order to make the correct cast.
Can someone please help solve it.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 4 images

- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardc++ class runner with constructor and COPY CONSTRUCTORarrow_forwardIf we want our classes to be open to extension, we may have to reorganize our dependencies to depend on __________ instead of concrete classes. (in Java) The Single Responsibility Principle (SRP)The Open-Closed Principle (OCP)The Liskov Substitution Principle (LSP)The Interface Segregation Principle (ISP)The Dependency Inversion Principle (DIP)arrow_forward
- What are the benefits of having a destructor for a class?arrow_forwardProgramming Assignment #5 Educational Objectives: After completing this assignment the student should have the following knowledge, ability, and skills: Define a class hierarchy using inheritance Define virtual member functions in a class hierarchy Implement a class hierarchy using inheritance Implement virtual member functions in a class hierarchy Use initialization lists to call parent class constructors for derived class constructors Operational Objectives: Create (define and implement) a base class Shape classes that will inherit this class called Box, Rectangle, Circle, and Triangle Description: Programming Specifications: You will have a base class of Shape which will have an area and perimeter of type double. You must also create acceptable Gets and Sets for the private data items. All of the other classes will inherit this class. You will have a class Square which will have just one length of type double. You should create acceptable Gets and Sets for the one…arrow_forwardInheritance, Polymorphism, ArrayLists, Throwing Exceptions The UML diagram below shows a set of classes designed to represent a music collection from 1995. The constructors and methods all function in the standard way, except: The equipmentRequired method should return “Record Player” or “CD Player” as appropriate.The getAlbum method of the NinetiesMusicCollection class accepts an index and returns the corresponding Album object. This method throws an IllegalArgumentException if the index is out of range. (This is the only exception you have to throw anywhere in your code.)In the NinetiesMusicCollection constructor, you can assume the ArrayList<Album> object passed as an argument is not null . Don’t worry about privacy leaks.Implement this set of classes in Java. Note the italics on the Class name “Album” and the method name “equipmentRequired” in the Album class.arrow_forward
- Describe the access modifiers that you can use for the members of a classarrow_forwardTrue or False A child class cannot override the parent’s definition of an inherited method.arrow_forward1 using static System.Console; 2 class ShapesDemo 3 { static void Main() { abstract class GeometricFigure Create an application named ShapesDemo that 4 creates several objects 5 that descend from an abstract class called 7 { GeometricFigure . Each 8 public int Height { 10 11 9 GeometricFigure get includes a height , a { 12 13 width , and an area. return height; } 14 15 Provide get and set set { 16 17 accessors for each field height = value; except area ; the area } 18 is computed and is read- } only. Include an abstract 19 public int Width 20 { 21 22 method called get ComputeArea() that { computes the area of the 23 24 return width; GeometricFigure . 25 26 27 28 29 set Next you will create three { width = value; additional classes derived from the } 30 } 31 GeometricFigure class. Name these derived classes: Rectange , 32 public int Area 33 { 34 Square , and Triangle. get 35 { 36 37 return area; } 38 } 39 40 private void CalArea() 41 { 42 43 } 44 area = Height * Width; 45 } 46arrow_forward
- Exercise 1-Account class • Design a class named Account that contains : • A private int data field named id for the account • A private double data field named balance for the account • A privet Date data field named dateCreated that stores the date when the account was created • A no-arg constructor that creates a default account • A constructor that creates an account with the specified id and initial balance • The getters (i.e., accessors) and setters (i.e., mutators) methods for id and balance • The getter method for dateCreated • A method named withdraw that withdraws a specified amount from the account • A method named deposit that deposits a specified amount to the accountarrow_forwardWhy might a class need to implement a destructor?arrow_forwardDesign a Ship class that has the following members:• A field for the name of the ship (a string).• A field for the year that the ship was built (a string).• A constructor and appropriate accessors and mutators.• A toString method that displays the ship's name and the year it was built.Disign a CruiseShip class that extends the Ship class. The CruiseShip class should have thefollowing members:• A field for the maximum number of passengers (an int).• A constructor and appropriate accessors and mutators.• A toString method that overrides the toString method in the base class. The CruiseShipclass's toString method should display only the ship's name and the maximum number ofpassengers.Design a CargoShip class that extends the Ship class. The CargoShip class should have thefollowing members:• A field for the cargo capacity in tonnage (an int).• A constructor and appropriate accessors and mutators.• A toString method that overrides the toString method in the base class. The CargoShipclass's…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
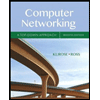
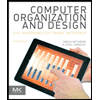
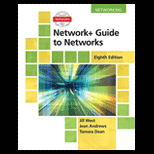
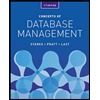
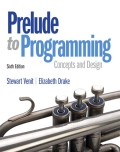
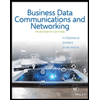