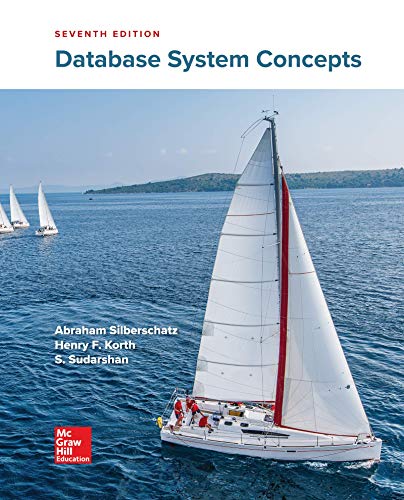
Concept explainers
Programming Assignment #5
Educational Objectives:
After completing this assignment the student should have the following knowledge, ability, and skills:
Define a class hierarchy using inheritance
Define virtual member functions in a class hierarchy
Implement a class hierarchy using inheritance
Implement virtual member functions in a class hierarchy
Use initialization lists to call parent class constructors for derived class constructors
Operational Objectives:
Create (define and implement) a base class Shape classes that will inherit this class called Box, Rectangle, Circle, and Triangle
Description:
Programming Specifications:
You will have a base class of Shape which will have an area and perimeter of type double. You must also create acceptable Gets and Sets for the private data items. All of the other classes will inherit this class.
You will have a class Square which will have just one length of type double. You should create acceptable Gets and Sets for the one data type. This class will inherit the base class
You will have a class Circle which will have just one length of type double which will be the radius. You should create acceptable Gets and Sets for the one data type. This class will inherit the base class
You will have a class Rectangle which will have two lengths of type double. You should create acceptable Gets and Sets for the two data types. This class will inherit the base class
You will have a class Triangle which will have three lengths of type double. You should create acceptable Gets and Sets for the two data types. This class will inherit the base class
You will have one virtual Member Function for all of the classes (Square, Rectangle, Circle, and Triangle) which will be called PrintData() which will print the details of the shape. All will have the area and perimeter but Circle will have a radius, Square will have one side, Rectangle will have two, and the Triangle will have three.
You should have two constructors for each class. A default constructor and one that accepts all of the private data items.
All sizes should be positive double floating point numbers at or above 1.00. There is no maximum size. If a size is entered less than 1.00 it should default to 1.00.
Write your own main routine to test your class. The test case should encompass using both constructors for each class, ensuring default values are properly used, and calling each method at least once.
Deliverables:
Tar file containing:
shape.h, shape.cpp
square.h, square.cpp
rectangle.h, rectangle.cpp
circle.h circle.cpp
triangle.h, triangle.cpp
main.cpp
makefile
Required
Square Perimeter:
4 * side.
Square Area:
side*side
Rectangle Perimeter:
Side1*2 + Side2*2
Rectangle Area:
Side1 * Side2
Circle Area:
Pi * Radius*Radius
Circle Perimeter:
Pi * 2*Radiuis
Triangle Area:
Assume we know three sides (Side1, Side2, Side3) then the area based on Heron’s formula which gives the area in terms of the three sides of the triangle. First calculate s which is (Side1 + Side2 + Side3)/2. Then obtain the square root of the absolute value of following formula: s*(s – Side1)*(s – Side2)*(s – Side3).

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 13 images

- The Point2D should store an x and y coordinate pair, and will be used to build a new class via class composition. A Point2D has a x and a y, while a LineSegment has a start point and an end point (both of which are represented as Point2Ds). class Invariants The start and end points of a line segment should never be null Initialize these to the origin instead. Data A LineSegment has a start point This is a Point2D object All data will be private A LineSegment also has an end point. Also a Point2D object Methods Create getters and setters for your start and end points public Point2D getStartPoint() { public void setStartPoint(Point2D start) { Create a toString() function to build a string composed of the startPoint’s toString() and endPoint’s toString() Should look like “Line start(0,0) and end(1,1)” Create an equals method that determines if two LineSegments are equal public boolean equals(Object other) { if(other == null || !(other instanceof LineSegment)) return…arrow_forwardDefine a Course base class with the following attributes: number - course number title - course title Define a print_info() method in Course that displays the course number and title. Also define a derived class OfferedCourse with the additional attributes: instructor_name - instructor name location - class location class_time - class timearrow_forwardTrue or false?(a) Every object is an instance of the object class.(b) If a class does not extend a superclass explicitly, it extends object by default.arrow_forward
- True or False? Because child classes in generalizations inherit the attributes, operations, and relationships of the parent, you must only define for the child the attributes, operations, or relationships that are distinct from the parent.arrow_forwardTrue or False A child class cannot override the parent’s definition of an inherited method.arrow_forwardWrite UML Class Diagram that illustrates the following program: 1) Person class The person class should include the following data fields; • Name • ID number • birth date • home address • phone number Be sure to include get and set methods for each data field. 2) Course class The course class should contain the following fields: • ID Number • Name College (Business, Engineering, Nursing, etc) • An Array of person objects . 3) University class The University class should contain the data fields for the university, such as: • University name • Federal ID number • Address • Array / ArrayList of course class objects • Array / ArrayList of person class objects Extended Classes: 4 & 5) Extend the Person class into Student class and Employee class 6) Extend the Employee class into Instructor class and Staff class 7 & 8) Extend the Student class into graduate Student and undergrad Student class 9) Registration Class ---> The Registration class creates transactions to link each student with…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
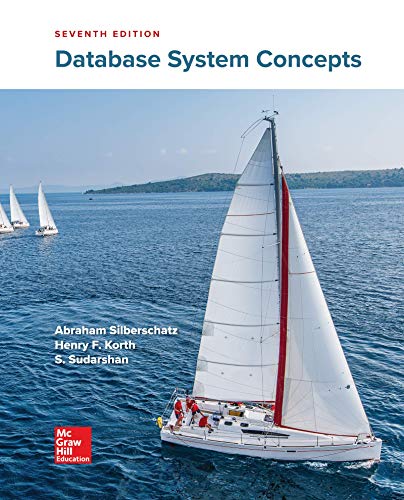
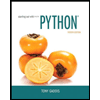
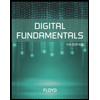
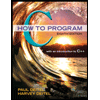
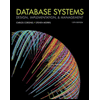
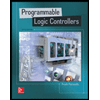