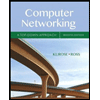
Ruby-related coding: Need help in resolving the red-selected code parts of my practice problem, primarily dealing with case-sentitive, loops, and if-else statements. The ", :pending => true" needs to be removed, used for checking purposes. (Picture reference provided)
Hangperson_game.rb
class HangpersonGame
# add the necessary class methods, attributes, etc. here
# to make the tests in spec/hangperson_game_spec.rb pass.
# Get a word from remote "random word" service
# def initialize()
# end
def initialize(word)
@word = word
@guesses = ""
@wrong_guesses = ""
end
def word
return @word
end
def guesses
return @guesses
end
def wrong_guesses
return @wrong_guesses
end
def guess(letter)
if @word.include?(letter)
@guesses += letter
return true
else
@wrong_guesses += letter
end
end
def word_with_guesses
partial_matches = ""
@word.each_char do |w|
partial_matches += "-"
end
return partial_matches
end
def check_win_or_lose
if word_with_guesses.downcase == @word.downcase
return :win
elsif @wrong_guesses.length >= 7
return :lose
else
return :play
end
end
# You can test it by running $ bundle exec irb -I. -r app.rb
# And then in the irb: irb(main):001:0> HangpersonGame.get_random_word
# => "cooking" <-- some random word
def self.get_random_word
require 'uri'
require 'net/http'
uri = URI('http://randomword.saasbook.info/RandomWord')
Net::HTTP.new('randomword.saasbook.info').start { |http|
return http.post(uri, "").body
}
end
end
Hangperson_game_spec.rb
require 'spec_helper'
require 'hangperson_game'
describe HangpersonGame do
# helper function: make several guesses
def guess_several_letters(game, letters)
letters.chars do |letter|
game.guess(letter)
end
end
describe 'new' do
it "takes a parameter and returns a HangpersonGame object" do
@hangpersonGame = HangpersonGame.new('glorp')
expect(@hangpersonGame).to be_an_instance_of(HangpersonGame)
expect(@hangpersonGame.word).to eq('glorp')
expect(@hangpersonGame.guesses).to eq('')
expect(@hangpersonGame.wrong_guesses).to eq('')
end
end
describe 'guessing' do
context 'correctly' do
before :each do
@game = HangpersonGame.new('garply')
@valid = @game.guess('a')
end
it 'changes correct guess list' do
expect(@game.guesses).to eq('a')
expect(@game.wrong_guesses).to eq('')
end
it 'returns true' do
expect(@valid).not_to be false
end
end
context 'incorrectly' do
before :each do
@game = HangpersonGame.new('garply')
@valid = @game.guess('z')
end
it 'changes wrong guess list' do
expect(@game.guesses).to eq('')
expect(@game.wrong_guesses).to eq('z')
end
it 'returns true' do
expect(@valid).not_to be false
end
end
context 'same letter repeatedly' do
before :each do
@game = HangpersonGame.new('garply')
guess_several_letters(@game, 'aq')
end
it 'does not change correct guess list', :pending => true do
@game.guess('a')
expect(@game.guesses).to eq('a')
end
it 'does not change wrong guess list', :pending => true do
@game.guess('q')
expect(@game.wrong_guesses).to eq('q')
end
it 'returns false', :pending => true do
expect(@game.guess('a')).to be false
expect(@game.guess('q')).to be false
end
it 'is case insensitive', :pending => true do
expect(@game.guess('A')).to be false
expect(@game.guess('Q')).to be false
expect(@game.guesses).not_to include('A')
expect(@game.wrong_guesses).not_to include('Q')
end
end
context 'invalid' do
before :each do
@game = HangpersonGame.new('foobar')
end
it 'throws an error when empty', :pending => true do
expect { @game.guess('') }.to raise_error(ArgumentError)
end
it 'throws an error when not a letter', :pending => true do
expect { @game.guess('%') }.to raise_error(ArgumentError)
end
it 'throws an error when nil', :pending => true do
expect { @game.guess(nil) }.to raise_error(ArgumentError)
end
end
end
describe 'displayed word with guesses' do
before :each do
@game = HangpersonGame.new('banana')
end
# for a given set of guesses, what should the word look like?
@test_cases = {
'bn' => 'b-n-n-',
'def' => '------',
'ban' => 'banana'
}
@test_cases.each_pair do |guesses, displayed|
it "should be '#{displayed}' when guesses are '#{guesses}'" do
guess_several_letters(@game, guesses)
expect(@game.word_with_guesses).to eq(displayed)
end
end
end
describe 'game status' do
before :each do
@game = HangpersonGame.new('dog')
end
it 'should be win when all letters guessed', :pending => true do
guess_several_letters(@game, 'ogd')
expect(@game.check_win_or_lose).to eq(:win)
end
it 'should be lose after 7 incorrect guesses', :pending => true do
guess_several_letters(@game, 'tuvwxyz')
expect(@game.check_win_or_lose).to eq(:lose)
end
it 'should continue play if neither win nor lose', :pending => true do
guess_several_letters(@game, 'do')
expect(@game.check_win_or_lose).to eq(:play)
end
end
end
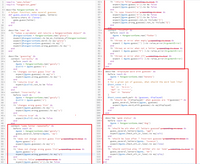

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- C++ Assignment---This is an unfinished code please complete it. Make changes if necessary. Upvote for finished working code. Objectives • To apply knowledge of classes and objects Instructions Implement all of the following, each in a separate Visual Studio project (and/or solution). 1. Define a class called CounterType to implement a simple counter. The class must have a private field (data member) counter of type int. You must also provide methods (member functions), including the following: a. A constructor to set the counter to the value specified by the user b. A constructor to set the counter to 0 c. A method to allow the user to set the counter to a value they specify d. A method to increment the counter by 1 e. A method to decrement the counter by 1 Your code must ensure the value of counter is never negative. If the user calls a method to attempt to make the counter negative, set the counter to 0 and print out an error to the user (e.g., “The counter must not be negative.”) Do…arrow_forwardWhat are the three things you need to do when working with classes that include pointer variables as member variables?arrow_forward!! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forward
- Goal 1: Update the Fractions Class Here we will overload two functions that will used by the Recipe class later: multipliedBy, dividedBy Previously, these functions took a Fraction object as a parameter. Now ADD an implementation that takes an integer as a parameter. The functionality remains the same: Instead of multiplying/dividing the current object with another Fraction, it multiplies/divides with an integer (affecting numerator/denominator of the returned object). For example; a fraction 2/3 when multiplied by 4 becomes 8/3. Similarly, a fraction 2/3 when divided by 4 becomes 1/6. Goal 2: Update the Recipe Class Now add a private member variable of type int to denote the serving size and initialize it to 1. This means all recipes are initially constructed for a single serving. Update the overloaded extraction operator (<<) to include the serving size (sample output below for an example of formatting) . New Member functions to the Recipe Class: 1. Add four member functions…arrow_forwardClasses, Objects, Pointers and Dynamic Memory Program Description: This assignment you will need to create your own string class. For the name of the class, use your initials from your name. The MYString objects will hold a cstring and allow it to be used and changed. We will be changing this class over the next couple programs, to be adding more features to it (and correcting some problems that the program has in this simple version). Your MYString class needs to be written using the .h and .cpp format. Inside the class we will have the following data members: Member Data Description char * str pointer to dynamic memory for storing the string int cap size of the memory that is available to be used(start with 20 char's and then double it whenever this is not enough) int end index of the end of the string (the '\0' char) The class will store the string in dynamic memory that is pointed to with the pointer. When you first create an MYString object you should…arrow_forwardExplain how to use wrapper classes for non-object data types so that you don't end up with a jumbled bag of non-object and object values?arrow_forward
- Implementation does not work, please include some test casesarrow_forwardNeed help with Ruby-related code; I'm tackling a sample practice problems and in the selected areas, I wanted to know how I can properly define and adjust the code to function. The ", :pending => true" parts have been removed/scratched out in order to allow the code to check if it works. (It's for checking purposes, ignore it) Topic: Case-sensitive and If else statement checksarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
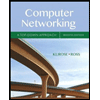
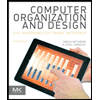
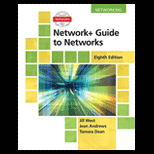
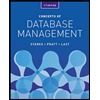
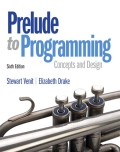
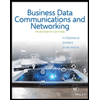