Python help!!! Hello, I need to solve this with no advanced functions or anything in an hour and I have no idea of how to do it. I appreciate your help, thank you very much. *it has to have different functions with no more than 30 lines in each. *no advanced material, as simple as possible because I'm new at this. *please read the instructions carefully
Python help!!! Hello, I need to solve this with no advanced functions or anything in an hour and I have no idea of how to do it. I appreciate your help, thank you very much. *it has to have different functions with no more than 30 lines in each. *no advanced material, as simple as possible because I'm new at this. *please read the instructions carefully
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Python help!!!
Hello, I need to solve this with no advanced functions or anything in an hour and I have no idea of how to do it. I appreciate your help, thank you very much.
*it has to have different functions with no more than 30 lines in each.
*no advanced material, as simple as possible because I'm new at this.
*please read the instructions carefully

Transcribed Image Text:c's contact info:
email: A
phone: B
However, you should replace C, A, and B with the actual name, email, and phone number. If there are no matching contacts, then
print
None
In order to pass the test cases, you'll need to print the matching contacts in sorted order. In order to do so:
• Iterate through all contacts, looking for matches
• Put each matching contact tuple into a separate set of results
• Once you have collected the set of all matches, loop through the matches in this way:
matches = {. . .}
for contact in sorted(matches):
# print each contact
Using that sorted function in the loop will give you the contacts in sorted order. After processing the command, the program
should prompt the user for the next command afterward.
Adding a Contact
When add contact is typed, the program will continue to prompt the user for three additional values: The name, email, and number
of the contact. Once it prompts the user for these three things, the program will add this new contact to the set of contact info
that are stored in the program. Below is an example of what this should look like:
> add contact
nаme: X
email: Y
phone: Z
contact added!
This is what it would look like, but the 0, and 2 values will be custom values entered by the user. Each time an add contact
command is processed, a new contact should be added to the set. You do not need to validate that D, and 2 are any particular
type of input.
Exit
If the user types exit (all lower case) then the program should print an exit message and end. For example:
> exit
Goodbye!

Transcribed Image Text:In this assignment, you will implement a very simple contact organizer application in Python. You should write a program called
contact_manager.py). Using this application, you will be able to request contact information, as well as add new contacts to the
contact manager.
You are NOT allowed to use dictionaries or lists (with some exceptions). The exception is that you MAY use lists when it comes to
reading in content from a file and splitting the lines, but you may not use them for storing the contacts for later access. You may
also use a list when it comes to printing out the contacts, in sorted order Thus, you should use sets and tuples.
Specifically, I recommend storing the contacts in a set of tuples. The set will contain all of the contacts. Each contact will be
represented by a tuple, and each tuple should contain exactly three values: name, email, and phone number. Your program
should not allow a contact with the same name, email, and phone number to appear twice. Using a set can help you maintain this
property of the contacts!
Reading froma file
The contacts should also be able to be loaded from a file called contacts.txt.
contacts. txt) will have a file format that looks like the following:
Bill Jones | bjon@gmail.com | 528-999-8765
Sam Dekker | dekker@apple.com | 123-456-7890
Janet Keller| kel@yahoo.com | 456-2356
James Jamie | jjamie@gmail.com | 435-234-2334
The precise names, emails, and phone numbers may vary. Also, the number of contacts in the file may vary. You should not
assume that the file has any particular number of contacts in it, but you can assume that the file exists. You can assume that the
contacts.txt file is in the same directory as the program you are implementing (Eontact_manager.py)
When contact_manager.py begins, it should read in and save all of the contact names, emails, and phone numbers into the program.
Since you won't know how many contacts are in the file ahead-of-time, you should use a data structure to store the contacts.
You should store the contacts in a set of tuples. Each tuple within the set should have the name, email, and phone number, in that
order. Using a set will automatically guarantee that no duplicate contact name+email+number will be able to be stored.
Command Types
After optionally reading in all of the contacts from the contacts file, your program should repeatedly prompt the user to enter in
commands. Your program should support three main types of commands.
Showing contacts
The user can enter a command of the form show contacts with A B), where A is either name, email, or phone, and B is the name or email
or phone to search for. For instance, the user could type show contacts with name Indiana Jones, where a is name and B is Indiana Jones.
Or, the user could type show contacts with emaii a@gmail.com where A would be email and B would be a@gmail.com.
If the command that a user types begins with show contacts with, then you can assume that the @ and B components exist. You
should loop through the set of contacts and print out all of the ones where the name, email, or phone matches the search string.
Contacts should be reported with the following format:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
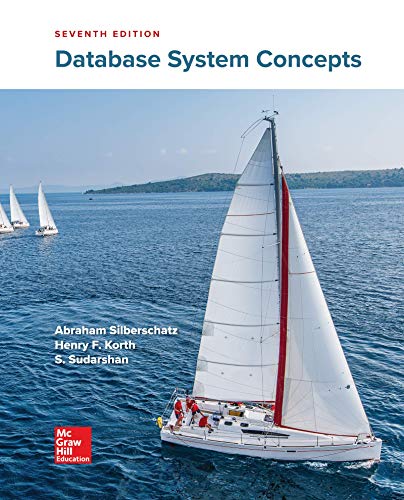
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
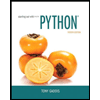
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
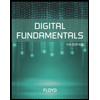
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
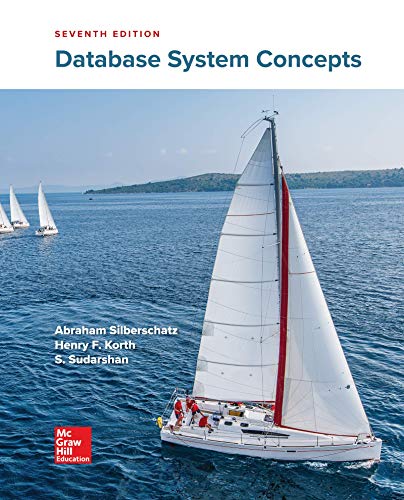
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
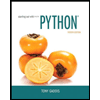
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
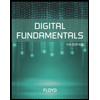
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
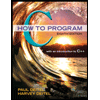
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
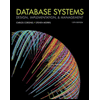
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
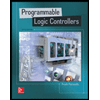
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education