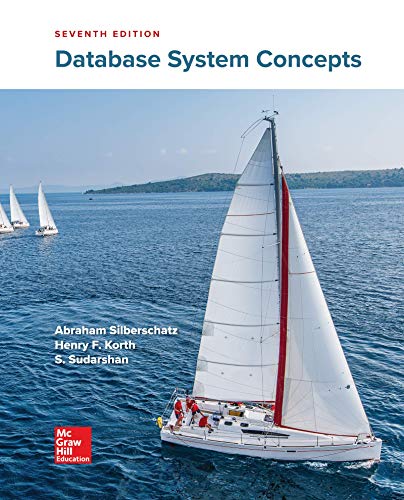
astfoodStats Assignment Description
For this assignment, name your R file fastfoodStats.R
- For all questions you should load tidyverse, openintro, and lm.beta. You should not need to use any other libraries.
suppressPackageStartupMessages(library(tidyverse))
suppressPackageStartupMessages(library(openintro))
suppressPackageStartupMessages(library(lm.beta))
The actual data set is called fastfood.
- Continue to use %>% for the pipe. CodeGrade does not support the new pipe.
- Round all float/dbl values to two decimal places.
- All statistics should be run with variables in the order I state
- E.g., "Run a regression predicting mileage from mpg, make, and type" would be:
lm(mileage ~ mpg + make + type...)
To access the fastfood data, run the following:
fastfood <- openintro::fastfood |
- Create a correlation matrix for the relations between calories, total_fat, sugar, and calcium for all items at Sonic, Subway, and Taco Bell, omitting missing values with na.omit().
- Assign the matrix to Q1. The output should look like this:
calories total_fat sugar calcium calories 1.00 0.81 0.45 0.61 total_fat 0.81 1.00 0.10 0.24 sugar 0.45 0.10 1.00 0.67 calcium 0.61 0.24 0.67 1.00 |

Introduction
Co-relational matrix:
A correlation matrix is a matrix that depicts the linear relation of a number of variables. Pearson's correlation, which quantifies the sequential association between two variables, is displayed by the submissions inside the mixture. Pearson's correlations can vary from -1 to 1, with -1 representing a perfect negative linear relationship, 1 representing a perfect positive linear relationship, and 0 representing no linear relationship. In exploratory data analysis, the correlation matrix is frequently used to identify and visualize relationships between variables.
Step by stepSolved in 3 steps

- Invalid indexes in slicing expressions can produce an ‘out of bound’ exception.True or Flasearrow_forwardDesign a GUI for Book view class for the following Library Information System, which you have worked on 1 with the following details: Library Item Class Design and TestingDesign a class that holds the Library Item Information, with item name, author, publisher. Write appropriate accessor and mutator methods. Also, write a tester program that creates three instances/objects of the Library Items class. Extending Library Item Class (Library and Book Classes): Extend the Library Item class in (1) with a Book class with data attributes for a book’s title, author, publisher and an additional attributes as number of pages, and a Boolean data attribute indicating whether there is both hard copy as well as eBook version of the book. Demonstrate Book Class in a Tester Program with an object of Book class.arrow_forwardCan you help me please: In sorting your data, you will find searching important. You may need to look up a dancer that comes to your studio in a list of other potential clients. If your program is small enough, it may not use searching or sorting. If the program was a file browser, there may not be much searching and sorting but parents and other paths would be stored to peek into a folder. If your program is similar to a calculator and there isn't much searching and sorting, then please note this in your assignment submission. Continue to make your program more robust via algorithms or user interface during this assignment. Do turn in a current UML for this assignment. You should now be writing the code that allows your program to sort, search, and store data. Think about the following points: Does your program store input data? What is it? Sorting algorithm? Searching algorithm? If your program does something alternately, please write this up and submit it. With the…arrow_forward
- TechPreferences Object: let user TechPreferences = { preferredDatabase: "MongoDB", backendFrameworks: ["Express.js", "Django"], frontend Frameworks: ["React", "Angular", "Vue.js"], mobileFrameworks: ["Flutter", "React Native"], preferredIDE: "VS Code", continuousIntegration: true, }; Write a JavaScript function to process the information in "userTechPreferences" and answer the following: Display the person's preferred database. Identify and display any backend frameworks used by the person. Output the frontend frameworks the person is familiar with. Create a list of mobile frameworks the person is interested in. Display the preferred Integrated Development Environment (IDE). Check if the person uses continuous integration and display the corresponding message.arrow_forward/** To change this license header, choose License Headers in Project Properties.* To change this template file, choose Tools | Templates* and open the template in the editor.*/package DataStructures; import ADTs.StackADT;import Exceptions.EmptyCollectionException; /**** @author Qiong* @param <T>*/public class LinkedStack<T> implements StackADT<T> { int count;SinglyLinkedNode<T> top;public LinkedStack(){top = null;count = 0;}public LinkedStack(T data){top = new SinglyLinkedNode(data);count = 1;}@Overridepublic void push(T element) {// TODO implete the push method// The push method will insert a node with holds the given input into the top of the stack } @Overridepublic T pop() throws EmptyCollectionException {if (this.isEmpty()) throw new EmptyCollectionException();SinglyLinkedNode<T> node = top;top = top.getNext();count--;node.setNext(null);return node.getElement();} @Overridepublic T peek() throws EmptyCollectionException {return null;//TODO: Implement…arrow_forwardUsing Java: 1 Description of the Program In this assignment, you are asked to implement a graphical application that displays a list of courses. Briefly, this GUI will take the inputs (i.e., course information), store them in an array list, and display course information in the output area. Figure 1 shows the layout of the CourseDisplay GUI. Figure 1: A screenshot of the CourseDisplay GUI. 2 Details of the program You should have three Java files: 1. CourseDisplayFrame class that extends JFrame class (The main part of your program). 2. CourseDisplayViewer class, which contains the main method where a CourseDisplay object will be created, and the GUI will pop up (Suggested frame width of 450, and frame height of 400). 3. Course class, which is the class that models the Course objects (This file will be given to you). 1 In your CourseDisplayFrame class file, you should have the followings: • Input area: – A text label and a text field (suggested width of 30) for course code; – A text…arrow_forward
- Alert dont submit AI generated answer.arrow_forwardwrite the code to lookup, add and remove phoneentries from the phonebook text file . These are the code we use for the lookup, add and remove in the case statement.(Lookup)# Look someone up in the phone bookgrep $1 phonebook (add)echo "$1 $2" >> phonebooksort -o phonebook phonebook (Remove)grep -v "$1" phonebook > /tmp/phonebookmv /tmp/phonebook phonebook Write a complete Bash script which will accept up to three command-line arguments: the first one is either (lookup, add or remove), the second one is a name enclosed in single quotes and the third one (if doing an add) is a phone number enclosed in single quotes. Using a case statement to match on either lookup, add or remove, the script should then perform the requested operation. Again here, you should first check for null arguments before continuing with the rest of the script or requested operation. In addition, the person may not be in the phonebook for lookup or remove so, you need to account for this.arrow_forwardC++ Assignment Setup Part 1: Working With Process IDs Modify the getProcessID() function in the file named Processes.cpp The function must find and store the process's own process id The function must return the process id to the calling program. Note that the function currently returns a default value of -1.Hint: search for “process id” in the “System Calls” section of the Linux manual. Part 2: Working With Multiple Processes Modify the createNewProcess() function in the file named Processes.cpp as follows: The child process must print the message I am a child process! The child process must then return a string with the message I am bored of my parent, switching programs now The parent process must print the message I just became a parent! The parent process must then wait until the child process terminates, at which point it must return a string with the message My child process just terminated! and then terminate itself. Hint: search for “wait for process” in…arrow_forward
- Skybox class (M Visual Studios): Modify the skybox class appropriately to handle cube mapping. This involves loading six images for the cube map, setting appropriate texture parameters, and ensuring the normals of the cube model that encoded and runs sucessfully Task: The upload_CubeMap_images() function in the Skybox.cpp file needs to be implemented sucessfully according to the instructions.Instructions: Enable cube mapping: Use glEnable(GL_TEXTURE_CUBE_MAP) to enable cube mapping. Generate a texture ID: Use glGenTextures() to generate a texture ID to hold the cube map. This ID is stored in the tex_buffer_ID member variable of the Skybox class. Bind the texture ID: Use glBindTexture() to bind the texture ID as the current buffer. Make sure to bind it as a cube map using GL_TEXTURE_CUBE_MAP. Load six images: Load all six images into the correct "place" in the cube map. You can use the provided file paths (BMP_NEG_X_FILE_PATH, BMP_NEG_Y_FILE_PATH, etc.) to load the images. (Make sure to…arrow_forwardcan you please double-check this code? thank you!arrow_forwarddo it fastarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
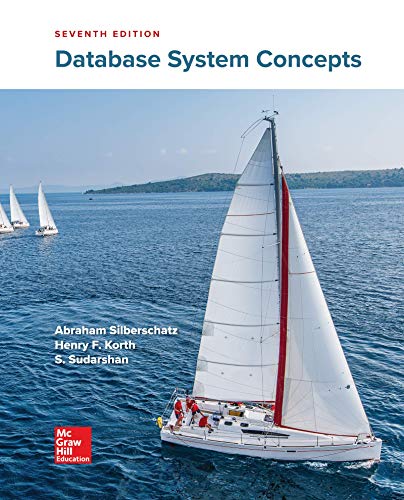
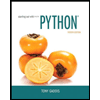
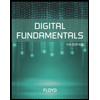
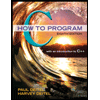
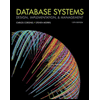
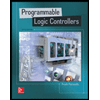