python code write a short Short Description of code and design Part One: Pizza Class: Create a Pizza class which stores information about a single pizza. It contains the following: -Instance variables to store the size of the pizza (small, medium or large), (1)the number of cheese toppings, (2)the number of pepperoni toppings, and the (3) number of mushroom toppings. - Constructor: that takes four arguments and sets all of the corresponding instance variables and that initializes all instance variables to the zero of their type. - Methods to get (accessor) and set (mutator) each instance variable individually. - A method called calcCost() that returns the cost of the pizza. Pizza cost is determined by: Small: $10 + $2 per topping Medium: $12 + $2 per topping Large: $14 + $2 per topping. -A method which returns a string for the pizza size, quantity of each topping, and the pizza cost as calculated by the following example, a large pizza with 1 cheese, 2 pepperoni and 1 mushroom toppings should cost $22 (you can overwrite __str__). Part Two: DeluxePizza Take the Pizza class from the above and modify it in the following way: Rename it to DeluxePizza. Add a stuffedWithCheese (boolean) attribute to the Add a veggieTopping (integer) attribute to the class. This attribute keeps track of the number of vegetables toppings excluding mushrooms. Add a class attribute numberOfPizzas (integer) which will keep track of the number of DeluxePizza objects created (this is not an instance variable). Update the existing constructor such that it increments the numberOfPizzas by one(+ 1) each time it is called and requires 6 arguments instead of 4 to accommodate the two new attributes (stuffedWithCheese and veggieTopping). Modify the constructor to set the new attributes to “zero” and to increment the numberOfPizzas attribute by one. Add an accessor and mutator method for the stuffedWithCheese and the veggieTopping attributes. Add an accessor method for the numberOfPizzas (No mutator attribute.) Modify the calcCost() method to add $2, $4 or $6 dollars for cheese stuffed dough depending on the size of the pizza as well as an extra $3 per vegetable Modify the __str__ method so that when an object of type DeluxePizza is printed all of theattributes are displayed in the following format: Pizza # Pizza size: Cheese filled dough: Number of cheese toppings: Number of pepperoni toppings: Number of mushroom toppings: Number of vegetable toppings: Extra features must be included to spice up the pizzeria! Some sample ideas can include additional menu items (such as ice cream), trio combos, different (multiple) orders, additional users etc.
python code
write a short Short Description of code and design
Part One: Pizza Class:
Create a Pizza class which stores information about a single pizza. It contains the following:
-Instance variables to store the size of the pizza (small, medium or large), (1)the number of cheese toppings, (2)the number of pepperoni toppings, and the (3) number of mushroom toppings.
- Constructor: that takes four arguments and sets all of the corresponding instance variables
and that initializes all instance variables to the zero of their type.
- Methods to get (accessor) and set (mutator) each instance variable individually.
- A method called calcCost() that returns the cost of the pizza. Pizza cost is determined by: Small: $10 + $2 per topping Medium: $12 + $2 per topping Large: $14 + $2 per topping.
-A method which returns a string for the pizza size, quantity of each topping, and the pizza cost as calculated by the following example, a large pizza with 1 cheese, 2 pepperoni and 1 mushroom toppings should cost $22 (you can overwrite __str__).
Part Two: DeluxePizza
Take the Pizza class from the above and modify it in the following way:
- Rename it to DeluxePizza.
- Add a stuffedWithCheese (boolean) attribute to the
- Add a veggieTopping (integer) attribute to the class. This attribute keeps track of the number of vegetables toppings excluding mushrooms.
- Add a class attribute numberOfPizzas (integer) which will keep track of the number of
DeluxePizza objects created (this is not an instance variable).
- Update the existing constructor such that it increments the numberOfPizzas by one(+ 1) each time it is called and requires 6 arguments instead of 4 to accommodate the two new attributes (stuffedWithCheese and veggieTopping).
- Modify the constructor to set the new attributes to “zero” and to increment the
numberOfPizzas attribute by one.
- Add an accessor and mutator method for the stuffedWithCheese and the veggieTopping
attributes.
- Add an accessor method for the numberOfPizzas (No mutator attribute.)
- Modify the calcCost() method to add $2, $4 or $6 dollars for cheese stuffed dough depending on the size of the pizza as well as an extra $3 per vegetable
- Modify the __str__ method so that when an object of type DeluxePizza is printed all of theattributes are displayed in the following format:
Pizza #
Pizza size:
Cheese filled dough:
Number of cheese toppings:
Number of pepperoni toppings:
Number of mushroom toppings:
Number of vegetable toppings:
Extra features must be included to spice up the pizzeria! Some sample ideas can include additional menu items (such as ice cream), trio combos, different (multiple) orders, additional users etc.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

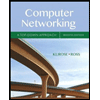
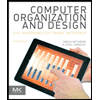
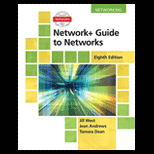
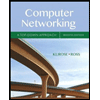
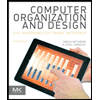
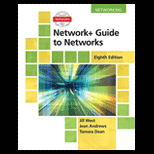
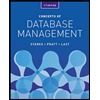
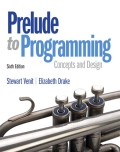
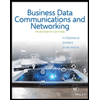