Python code for: The set of rules determining the fate of a cell: X is currently dead: If X has exactly three living neighbors, X becomes alive. In all other cases, X remains dead. X is currently alive: If X has zero or one living neighbor (s), X dies of loneliness. If X has two or three living neighbors, X remains alive. In all other cases, X dies of a shortage of living space. The class LifeGeneration represents one generation in a game of life. In the class LifeGeneration, do the following: Make an initializer method that accepts the state of the board as a 2-dimensional list of booleans (indicating if the cell is alive or not) Make the methods width() and height() that give the size of the board. Make a method is_alive(x,y) that returns if the cell at position x, y is alive. Make a method next_generation() that gives the next generation. Make a method is_all_dead() that returns if the generation is dead. Make a method board() that returns a 2-dimensional list of True, False values indicating if each cell is alive or not. You can add anything else to the class that you need class LifeGeneration: # fill in this class yourself def __init__(self, state): pass def next_generation(self): pass def board(self): pass The class LifeHistory represents a game of life that has been played for a number of generations (such as the whole illustration above). In the class LifeHistory do the following: Make an initializer method that takes an initial LifeGeneration as initial state. Make a method play_step() that adds a new generation to the history Make methods: nr_generations() get_generation(i) Make a method period() which returns the length of the oscillator if the game has become periodic, or None if it has not. Make a method dies_out() which returns True if the last generation is completely dead. Make a method play_out(n) which runs the game for a maximum of n steps, but stops if the game has become periodic or has died out. Add a method all_boards() that returns a list of all generations, where each generation is a 2-dimensional list of True, False values. You can add anything else to the class that you need. class LifeHistory: # fill in this class yourself def __init__(self, initial_gen): pass def nr_generations(self): pass def get_generation(self, i): pass def dies_out(self): pass def period(self): pass def play_out(self, max_steps): pass def all_boards(self): pass
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Python code for:
The set of rules determining the fate of a cell:
- X is currently dead:
- If X has exactly three living neighbors, X becomes alive.
- In all other cases, X remains dead.
- X is currently alive:
- If X has zero or one living neighbor (s), X dies of loneliness.
- If X has two or three living neighbors, X remains alive.
- In all other cases, X dies of a shortage of living space.
The class LifeGeneration represents one generation in a game of life. In the class LifeGeneration, do the following:
- Make an initializer method that accepts the state of the board as a 2-dimensional list of booleans (indicating if the cell is alive or not)
- Make the methods width() and height() that give the size of the board.
- Make a method is_alive(x,y) that returns if the cell at position x, y is alive.
- Make a method next_generation() that gives the next generation.
- Make a method is_all_dead() that returns if the generation is dead.
- Make a method board() that returns a 2-dimensional list of True, False values indicating if each cell is alive or not.
- You can add anything else to the class that you need
class LifeGeneration:
# fill in this class yourself
def __init__(self, state):
pass
def next_generation(self):
pass
def board(self):
pass
The class LifeHistory represents a game of life that has been played for a number of generations (such as the whole illustration above). In the class LifeHistory do the following:
- Make an initializer method that takes an initial LifeGeneration as initial state.
- Make a method play_step() that adds a new generation to the history
- Make methods:
- nr_generations()
- get_generation(i)
- Make a method period() which returns the length of the oscillator if the game has become periodic, or None if it has not.
- Make a method dies_out() which returns True if the last generation is completely dead.
- Make a method play_out(n) which runs the game for a maximum of n steps, but stops if the game has become periodic or has died out.
- Add a method all_boards() that returns a list of all generations, where each generation is a 2-dimensional list of True, False values.
- You can add anything else to the class that you need.
class LifeHistory:
# fill in this class yourself
def __init__(self, initial_gen):
pass
def nr_generations(self):
pass
def get_generation(self, i):
pass
def dies_out(self):
pass
def period(self):
pass
def play_out(self, max_steps):
pass
def all_boards(self):
pass

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

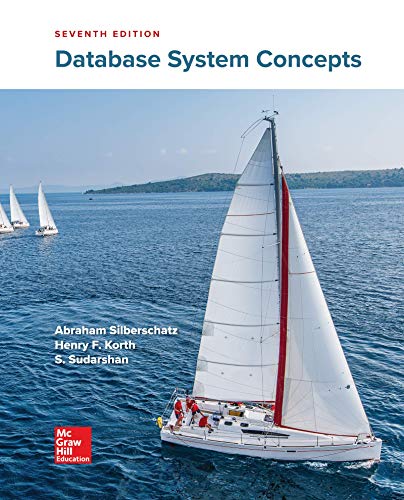
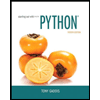
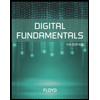
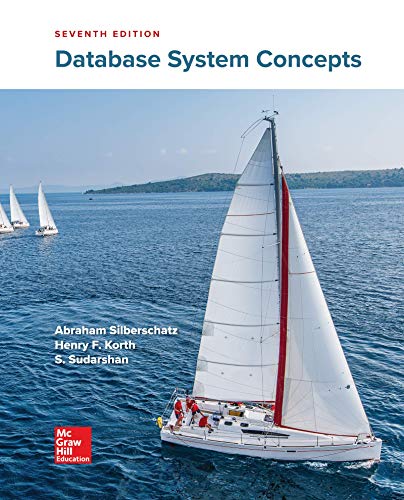
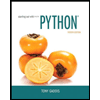
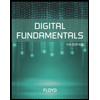
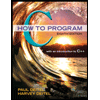
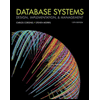
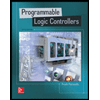