Provide full C++ code for playlist.cpp, playlist.h and main.cpp Update Checkpoint A First, add a member variable name that will store the name of the playlist. Now, make minor changes to your code so that the alternate constructor takes an additional parameter: a string, which represents the name of the playlist. Make another alternate constructor that takes a single parameter: a string, which represents the name of the playlist. This constructor creates an empty playlist with the name provided in the parameter. Update the overloaded << operator so that in addition to the number of songs in the playlist, and total playtime at the beginning, it also shows the following: (see format below) the name of the playlist, the description of each song ends with its uniqueId (see format below). After making those changes and slightly updating main.cpp provided in checkpoint A with these statements: PlayList a("P1", "file1.txt"); PlayList b("P2"); you should get the output that exactly matches: ***Playlist P1 contains 3 songs with a total playtime of 825 seconds*** "Peg" by Steely Dan is 237 seconds long (ID SD123). "All For You" by Janet Jackson is 391 seconds long (ID JJ234). "Black Eagle" by Janet Jackson is 197 seconds long (ID JJ456). Reversing the play list ***Playlist P2 contains 3 songs with a total playtime of 825 seconds*** "Black Eagle" by Janet Jackson is 197 seconds long (ID JJ456). "All For You" by Janet Jackson is 391 seconds long (ID JJ234). "Peg" by Steely Dan is 237 seconds long (ID SD123). Method: IsSongPresent This method has the following prototype: bool IsSongPresent(string uniqueID); //Determines if the song with given uniqueId is present in the playlist and returns accordingly. If the song with that uniqueID is already present in the playlist, it returns true. Otherwise, it returns false. Method: AddSong This method has the following prototype: bool AddSong(string uniqueID, string songName, string artistName, int songLength); //Adds song to the end of the list, if the song (based off the uniqueId) is not already present If the song with that uniqueID is already present in the playlist, it returns false along with the following message: "Song with unique ID insert_uniqueID_here already exists. Skipping ..." Otherwise, it adds the song to the end of the playlist and returns true. Method: RemoveSong This method has the following prototype: bool RemoveSong(string uniqueID); //Removes song with given uniqueId from the playlist, if it exists, and returns true. Otherwise, returns false. If the song with that uniqueID is not present in the playlist, it returns false along with the following message: "Song with unique ID insert_uniqueID_here does not exist." Otherwise, it removes the song from the playlist and returns true. Method: PlayFirstSong This method has the following prototype: bool PlayFirstSong(); //"Plays" first song if list is not empty and then deletes it from the PlayList. Otherwise, gives a message indicating list is empty. If the playlist is empty, it returns false along with the following message: "Sorry, play list is empty!" Otherwise, it plays the song from the playlist (with the description below), deletes it from the playlist, and returns true. "Playing insert_songName_here by insert_artistName_here" Method: Overload operator+ This method has the following prototype: PlayList operator+(const PlayList& other); It creates and returns a new playlist by concatenating the current playlist to other playlist but it does so while ensuring that no song is repeated. In addition, the name of the new playlist is simply the concatenation of the names of the current playlist and the other playlist. Example: if the current playlist is ***Playlist P1 contains 2 songs with a total playtime of 628 seconds*** "Peg" by Steely Dan is 237 seconds long (ID SD123). "All For You" by Janet Jackson is 391 seconds long (ID JJ234). and the other playlist is ***Playlist P2 contains 2 songs with a total playtime of 588 seconds*** "Black Eagle" by Janet Jackson is 197 seconds long (ID JJ456). "All For You" by Janet Jackson is 391 seconds long (ID JJ234). then adding these playlists should give: "Song with unique ID JJ234 already exists. Skipping ..." ***Playlist P1P2 contains 3 songs with a total playtime of 825 seconds*** "Peg" by Steely Dan is 237 seconds long (ID SD123). "All For You" by Janet Jackson is 391 seconds long (ID JJ234). "Black Eagle" by Janet Jackson is 197 seconds long (ID JJ456).
Provide full C++ code for playlist.cpp, playlist.h and main.cpp
Update Checkpoint A
- First, add a member variable name that will store the name of the playlist.
- Now, make minor changes to your code so that the alternate constructor takes an additional parameter: a string, which represents the name of the playlist.
- Make another alternate constructor that takes a single parameter: a string, which represents the name of the playlist. This constructor creates an empty playlist with the name provided in the parameter.
- Update the overloaded << operator so that in addition to the number of songs in the playlist, and total playtime at the beginning, it also shows the following: (see format below)
- the name of the playlist,
- the description of each song ends with its uniqueId (see format below).
After making those changes and slightly updating main.cpp provided in checkpoint A with these statements:
PlayList a("P1", "file1.txt");
PlayList b("P2");
you should get the output that exactly matches:
***Playlist P1 contains 3 songs with a total playtime of 825 seconds***
"Peg" by Steely Dan is 237 seconds long (ID SD123).
"All For You" by Janet Jackson is 391 seconds long (ID JJ234).
"Black Eagle" by Janet Jackson is 197 seconds long (ID JJ456).
Reversing the play list
***Playlist P2 contains 3 songs with a total playtime of 825 seconds***
"Black Eagle" by Janet Jackson is 197 seconds long (ID JJ456).
"All For You" by Janet Jackson is 391 seconds long (ID JJ234).
"Peg" by Steely Dan is 237 seconds long (ID SD123).
Method: IsSongPresent
This method has the following prototype:
bool IsSongPresent(string uniqueID); //Determines if the song with given uniqueId is present in the playlist and returns accordingly.
If the song with that uniqueID is already present in the playlist, it returns true. Otherwise, it returns false.
Method: AddSong
This method has the following prototype:
bool AddSong(string uniqueID, string songName, string artistName, int songLength); //Adds song to the end of the list, if the song (based off the uniqueId) is not already present
If the song with that uniqueID is already present in the playlist, it returns false along with the following message:
"Song with unique ID insert_uniqueID_here already exists. Skipping ..."
Otherwise, it adds the song to the end of the playlist and returns true.
Method: RemoveSong
This method has the following prototype:
bool RemoveSong(string uniqueID); //Removes song with given uniqueId from the playlist, if it exists, and returns true. Otherwise, returns false.
If the song with that uniqueID is not present in the playlist, it returns false along with the following message:
"Song with unique ID insert_uniqueID_here does not exist."
Otherwise, it removes the song from the playlist and returns true.
Method: PlayFirstSong
This method has the following prototype:
bool PlayFirstSong(); //"Plays" first song if list is not empty and then deletes it from the PlayList. Otherwise, gives a message indicating list is empty.
If the playlist is empty, it returns false along with the following message:
"Sorry, play list is empty!"
Otherwise, it plays the song from the playlist (with the description below), deletes it from the playlist, and returns true.
"Playing insert_songName_here by insert_artistName_here"
Method: Overload operator+
This method has the following prototype:
PlayList operator+(const PlayList& other);
It creates and returns a new playlist by concatenating the current playlist to other playlist but it does so while ensuring that no song is repeated. In addition, the name of the new playlist is simply the concatenation of the names of the current playlist and the other playlist.
Example: if the current playlist is
***Playlist P1 contains 2 songs with a total playtime of 628 seconds***
"Peg" by Steely Dan is 237 seconds long (ID SD123).
"All For You" by Janet Jackson is 391 seconds long (ID JJ234).
and the other playlist is
***Playlist P2 contains 2 songs with a total playtime of 588 seconds***
"Black Eagle" by Janet Jackson is 197 seconds long (ID JJ456).
"All For You" by Janet Jackson is 391 seconds long (ID JJ234).
then adding these playlists should give:
"Song with unique ID JJ234 already exists. Skipping ..."
***Playlist P1P2 contains 3 songs with a total playtime of 825 seconds***
"Peg" by Steely Dan is 237 seconds long (ID SD123).
"All For You" by Janet Jackson is 391 seconds long (ID JJ234).
"Black Eagle" by Janet Jackson is 197 seconds long (ID JJ456).

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

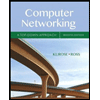
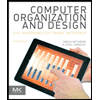
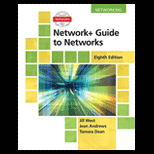
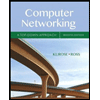
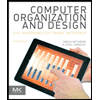
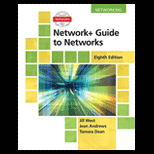
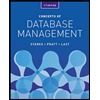
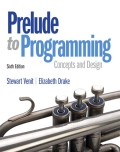
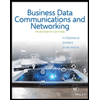