Program - Python This is my program for a horse race class (Problem below code) class Race: def __init__(self,name,time): self.name = name self.time = time self.entries = [] class Entrant: def __init__(self,horsename,jockeyname): self.horsename = horsename self.jockeyname = jockeyname race1 = Race('RACE 1','10:00 AM May 12, 2021') race2 = Race('RACE 2','11:00 AM May 12, 2021') race3 = Race('RACE 3','12:00 PM May 12, 2021') race1.entries.append(Entrant('Misty Spirit','John Valazquez')) race1.entries.append(Entrant('Frankly I’m Kidding','Mike E Smith')) race2.entries.append(Entrant('Rage against the Machine','Russell Baze')) race3.entries.append(Entrant('Secretariat','Bill Shoemaker')) race3.entries.append(Entrant('Man o War','David A Gall')) race3.entries.append(Entrant('Seabiscuit','Angel Cordero Jr')) print(race1.name, race1.time) for entry in race1.entries: print('Horse:',entry.horsename) print('Jockey:',entry.jockeyname) print() print(race2.name, race2.time) for entry in race2.entries: print('Horse:',entry.horsename) print('Jockey:',entry.jockeyname) print() print(race3.name, race3.time) for entry in race3.entries: print('Horse:',entry.horsename) print('Jockey:',entry.jockeyname) print() Problem: Need help to modify program so that Make a race class, which has attributes Race name - a string which is the name of the race Race time - the time of the race Race entrants - a list of the entrants to the race Make an entrant class, which has attributes Horse name - the name of the horse Jockey name - the name of the jockey
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Program - Python
This is my program for a horse race class (Problem below code)
class Race:
def __init__(self,name,time):
self.name = name
self.time = time
self.entries = []
class Entrant:
def __init__(self,horsename,jockeyname):
self.horsename = horsename
self.jockeyname = jockeyname
race1 = Race('RACE 1','10:00 AM May 12, 2021')
race2 = Race('RACE 2','11:00 AM May 12, 2021')
race3 = Race('RACE 3','12:00 PM May 12, 2021')
race1.entries.append(Entrant('Misty Spirit','John Valazquez'))
race1.entries.append(Entrant('Frankly I’m Kidding','Mike E Smith'))
race2.entries.append(Entrant('Rage against the Machine','Russell Baze'))
race3.entries.append(Entrant('Secretariat','Bill Shoemaker'))
race3.entries.append(Entrant('Man o War','David A Gall'))
race3.entries.append(Entrant('Seabiscuit','Angel Cordero Jr'))
print(race1.name, race1.time)
for entry in race1.entries:
print('Horse:',entry.horsename)
print('Jockey:',entry.jockeyname)
print()
print(race2.name, race2.time)
for entry in race2.entries:
print('Horse:',entry.horsename)
print('Jockey:',entry.jockeyname)
print()
print(race3.name, race3.time)
for entry in race3.entries:
print('Horse:',entry.horsename)
print('Jockey:',entry.jockeyname)
print()
Problem: Need help to modify program so that
Make a race class, which has attributes
Race name - a string which is the name of the race
Race time - the time of the race
Race entrants - a list of the entrants to the race
Make an entrant class, which has attributes
Horse name - the name of the horse
Jockey name - the name of the jockey

Step by step
Solved in 2 steps with 2 images

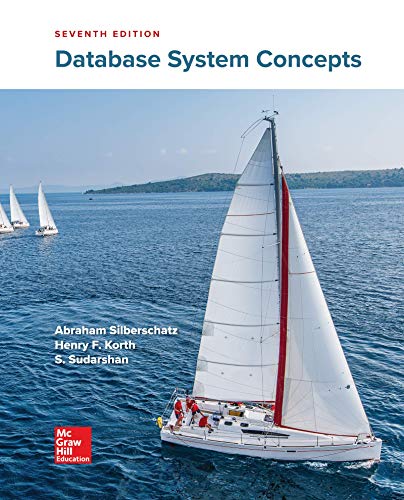
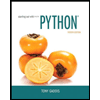
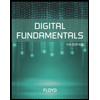
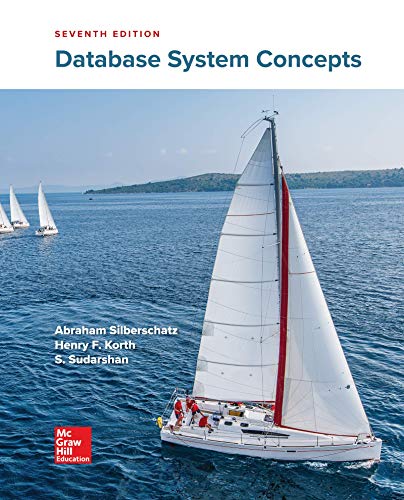
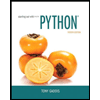
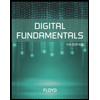
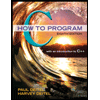
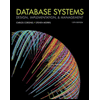
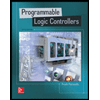