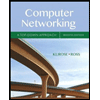
Problem: Implement a part of functionality for the Netflix DVD queue. It's a service that allows a user to create a list of desired movies and then sends DVDs with movies on top (what we called head in our lectures) of this list to the subscriber one at a time. A subscriber should be able to create a list of desired movies and manipulate the order in a movie queue in their account. Your program will implement some of the desired functionality by storing the list of movie titles in a linked list. You are provided with the following files available in the "Downloadable files" section:
- MovieList.h contains a class declaration for the class that represents a list of movies. top refers to the head position of a list and bottom refers to the tail or end position of a list.
- Driver.cpp contains the main function you can use to test your implementation.
*** NOTE: Please do not change any of the function names since our automated test script calls those functions. Changing function names or parameters will break the automated test script.
You will be responsible for providing the MovieList.cpp file, including the implementation of the MovieList member functions (described below). The class is defined in the MovieList.h file.:
- MovieList and ~MovieList: create an empty list and deallocates all the nodes in the list, respectively.
- display(ostream& out) Print movie titles from top to bottom, with positions numbered (put a colon and space between the number and the movie title) one movie per line. Use out << instead of cout <<.
- addToTop(string title) Add a movie to the top (head) of the list
- addToBottom(string title) Add a movie to the bottom (end) of the list
- moveToTop(string title) Move a movie with given title to position 0 (top or head)
- moveToPosition(string title, int position) Move movie with given title to position n (0 is top or head)
- remove(int n) Remove a movie at the given position. Return true if successful, false if there is no movie at position n.
Input/Output:
Use the provided Driver.cpp file to test your code. I recommend trying to implement one or two functions at a time, and testing them, rather than implementing all the functions and then trying to debug them all at once.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- How did you decide to handle the possibility of queue underflow in java? A. Assume as a precondition that it will not occur. B. Provide an isEmpty operation so a client can prevent underflow. C. Ignore it. D. Throw a QueueUnderflowException if it occurs. E. Throw a QueueUnderflowException if it occurs, and provide an isEmpty operation so a client can prevent underflowarrow_forwardConsider the BadReaderWriter.java program attached with this. The program has three threads, namely, one reader thread and two writer threads, and they all access the same list of numbers. The reader thread reads the list and prints it to the terminal. The writer threads append numbers to the list. At any point in time, if either of the writer threads finds that the list contains n elements, then it appends the number n + 1 to the list. Run this program, examine the output, and identify the problems.Fix these problems by implementing the acquireLock() and releaseLock() methods in the code.arrow_forwardDescription: In this project, you are required to make a java code to build a blockchain including multiple blocks. In each block, it contains a list of transactions, previous hash - the digital signature of the previous block, and the hash of the current block which is based on the list of transactions and the previous hash. If anyone would change anything with the previous block, the digital signature of the current block will change. When this changes, the digital signature of the next block will change. Detailed Requirements: 1. Define your block class which contains three private members and four public methods. a. Members: previousHash (int), transactions (String[]), and blockHash (int); b. Methods: i. Block( int previousHash, String[] transactions){} ii. getPreviousHash(){} iii. getTransaction(){} iv. getBlockHash(){} 2. Using ArrayList to design your Blockchain. 3. Your blockchain must contain genesis block and make it to be the first block. 4. You can add a block to the end of…arrow_forward
- You are to use the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. The program has to be in c++, and have to use the already started code below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in…arrow_forwardJava programming homework please helparrow_forwardSuppose a program builds and manipulates a linked list: What two special nodes would the program typically keep track of? Describe two common uses for the null reference in the node of the linked lists.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
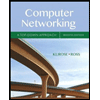
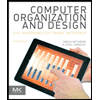
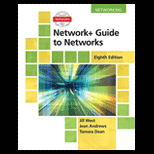
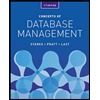
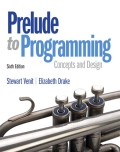
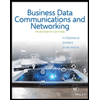