Problem: Write a program that prompts the user to enter 10 names, each with maximum of 30 characters. Output the names that start with letter A and its corresponding length. This is my C++ Codes but it has errors when I run it. Can you fix the errors? Please still use header files iostream and cstring. //Start of program #include #include using namespace std; int main() { //Declare array char name[10]; //Prompt the user to enter 10 names cout << "Enter 10 names: " << endl; //For loop for (int i = 0; i < 10; i++) { //Store each name in the array cin >> name[i]; if (strlen(name) > 30) { i--; } } //Display the names that start with A or a cout << "Names starting with 'A' and their corresponding length: " << endl; //Iterate the value of i from 0 to 9 by using for loop for (int i = 0; i < 10; i++) { //Checking condition if name starts with A or a if (name[i][0] == 'A' || name[i][0] == 'a') { cout << name[i] << ": " << strlen(name) << endl; } } //End of program return 0; }
Problem:
Write a
This is my C++ Codes but it has errors when I run it. Can you fix the errors? Please still use header files iostream and cstring.
//Start of program
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
//Declare array
char name[10];
//Prompt the user to enter 10 names
cout << "Enter 10 names: " << endl;
//For loop
for (int i = 0; i < 10; i++)
{
//Store each name in the array
cin >> name[i];
if (strlen(name) > 30)
{
i--;
}
}
//Display the names that start with A or a
cout << "Names starting with 'A' and their corresponding length: " << endl;
//Iterate the value of i from 0 to 9 by using for loop
for (int i = 0; i < 10; i++)
{
//Checking condition if name starts with A or a
if (name[i][0] == 'A' || name[i][0] == 'a')
{
cout << name[i] << ": " << strlen(name) << endl;
}
}
//End of program
return 0;
}

Step by step
Solved in 4 steps with 1 images

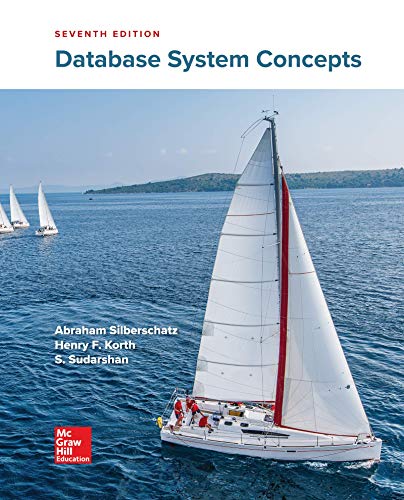
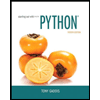
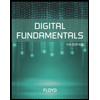
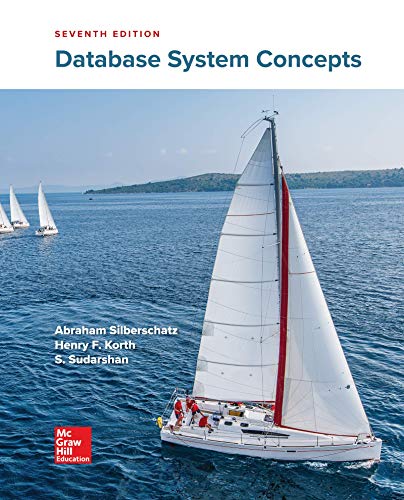
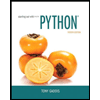
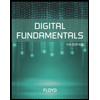
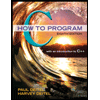
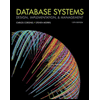
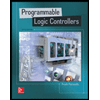