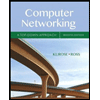
Please provide me java code for below problem:
Assignment Guidelines:
Text messaging is a popular means of communication. Many abbreviations are in common use but are not appropriate for formal communication. Suppose the abbreviations are stored, one to a line, in a text file named abbreviations.txt. For example, the file might contains these lines:
lol
:)
free
iirc
4
u
ttfn
Write a class AbreviationMarker that will read a message from another text file and surround each occurrence of an abbreviation(ignoring case) with <> brackets. Write the marked message to a new text file.
For example, if the message to be scanned is:
Ttfn, how are u today? Iirc, this is your first free day. Hope you are having fun! :)
the new text file should contain:
<Ttfn>, how are <u> today? <Iirc>, this is your first <free> day. Hope you are having fun! <:)>
----jGRASP exec: java AbreviationMarkerDemo
What is the file to read from?
in.txt
What is the file to write to with abbreviations marked
out.dat
What is the abbreviations file to read from?
abbreviations.txt
File processing completed.

Solution:
/*
// create and abbreviations.txt file in this format
for 4
you u
*/
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Write a class FileConverter with a constructor that accepts a String file name as its argument. It should store filename as its attribute (with a accessor getFilename(). The class should have the following methods: • createUpperCaseFile(): Accepts a String parameter targetFilename. It will open up both the file specified in class attribute filename and the method parameter targetFilename. Then it will convert all lines of the class attribute filename to upper case and write it to target file, line by line. • createRowCharCountFile(): Accepts a String parameter targetFilename. It will open up both the file specified in class attribute filename and the method parameter targetFilename. Then it will count the number of chars in each line of the class attribute filename and write it to target file line by line. The class must come with a main() method, which will create an object instance and generate two target files based on the source file, one all upper case, and another row char count.arrow_forwardjava programming I have a Java program with a restaurant/store menu. Can you please edit my program when displaying the physical receipt? I would like the physical receipt to export as text file, not print the console's order receipt. import java.util.*; public class Restaurant2 { publicstaticvoidmain(String[] args) { // Define menu items and pricesString[] menuItems= {"Apple", "Orange", "Pear", "Banana", "Kiwi", "Strawberry", "Grape", "Watermelon", "Cantaloupe", "Mango"};double[] menuPrices= {1.99, 2.99, 3.99, 4.99, 5.99, 6.99, 7.99, 8.99, 9.99, 10.99};StringusersName; // The user's name, as entered by the user.ArrayList<String> arr = new ArrayList<>(); // Define scanner objectScanner input=new Scanner(System.in); // Welcome messageSystem.out.println("Welcome to AppleStoreRecreation, what is your name:");usersName = input.nextLine(); System.out.println("Hello, "+ usersName +", my name is Patrick nice to meet you!, May I Take Your Order?"); // Display menu items and…arrow_forward6. Design a Java class with a main method that does the following. You must include a meaningful comment for main and each method a. Main invokes a method readScores to read input from a file (name of your choice) that contains the name of the bowlers (no more than 25) and their bowling scores (two scores per bowler). The program stops reading values when it has reached the end of the file. For example, the file might contain: Bob 203 176 Jane 210 253 Jack 300 189 Eileen 220 298 • As the method reads the data, it stores the name into a String array and the average of the two bowling scores into an array of double. • The method returns the actual number of bowlers read in b. Main then invokes a method computeOverallAvg to: compute (a) the overall average score of all the bowlers combined, (b) the average score and name of the bowler with the highest average score and (c) the average score and name of the bowler with the lowest average scores. print to the console (a) the overall average…arrow_forward
- Java programming 1. Write the code to print out an individual customer’s information. Your code must read the customer from a file, populate the object, and display the data from the object. Customer customer = new Customer (); 2. Write the signature line for this method 3, Write the line of code to read a line from the file Assume the object has been populated with the proper values. Write the line of code that will print one of the properties in the customer object. Be sure to include a textual description, e.g. “Customer Name: “arrow_forwardCreate a file named Question.py and then create a class on it named Question. A Question object will contain information about a trivia question. It should have the following: A constructor, with the following parameters: 1 string to be the question itself (for example: "When was Cypress College Founded?"). A list of four strings, each a possible answer to the question (only one of them should be correct) An integer named 'answer': the index in the list of the correct answer. Example: if the third answer in the answer list is the correct one, the client should pass 2 to this parameter to signify the correct answer is in index 2. Overload the __str__ method. It should return a string made up of the question and the four possible answers. A ‘guess’ method, with an integer parameter. If the 'answer' attribute matches the int passed to 'guess', return True. Otherwise, False On Main.py, in your main function, create a list and add four Question objects to it. You may give them with…arrow_forward1. Complete the class code below and include a method named "search" that takes two String parameters, a string to search and the other a target string to search for in the first string. The search method will return an integer representing the number of times the search string was found. The string being searched is a super-string formed by joining all lines of a text file, so we might expect to get multiple hits with our method. HINT: walk the String in a loop and use .substring(start, end) to examine a target-sized segment of the input string for equality to your target word. public class WordSearch { public static void main(String[] args) { String fileName String target = "help"; Scanner fileln = new Scanner(new File(fileName)); "story.txt"; %D String story = while(fileln.hasNextLine()) { story += fileln.nextLine(); // Build a super-String } System.out.printIn("(" + target + ") found "+ search(story, target) + " times"); } /l method code here } // end of WordSearch classarrow_forward
- Challenge 3: Encryption.java and Decryption.java Write an Encryption class that encrypts the contents of a binary file. Your encryption program should work like a filter, reading the contents of one file, modifying the data into a code, and then writing the coded content out to a second file. The second file will be a version of the first file, but written in a secret code. Use a simple encryption technique, such as reading one character at a time, and add 10 to the character code of each character before it is written to the second file. Come up with your own encryption method. Also write a Decryption class that reads the file produced by the Encryption program. The decryption file will use the method that you used and restore the data to its original state, and write it to a new file.arrow_forward2. Name the demo file firstname_lastname_PE2 Create a program that simulates a dumpster diving game. Dumpster diving is when you search for loot in large industrial sized trash bins. In the game you will roll a ten-sided dice to determine what the user has received. Use the UML below as a guide for the Die class (Name the class Die.java). Die - sideUp: int = 10 - value: int + Die() + roll(): void + getvalue(): int Below are some suggestions to aid you in designing the game: • Each round of the game is performed as an iteration of a loop that repeats as long as the player wants to "dive" for more items. • At the beginning of each round, the program will ask the user whether or not he or she wants to continue diving. The program simulates the rolling of a ten-sided die Each item that can be caught is represented by a number generated from the die; for example, 1 for "a half-eaten sandwich", 2 for "a left shoe", 3 for "a broken watch", and so on. • Each item the user catches is worth a…arrow_forwardPython3 code: Create a simple text file called mycandy The first line of the file will be Candy Name, Candy Calories, Total Fat Grams Next, add five lines to the file by hand using your favorite five candy bars information. Each line's data will be separated by commas. Create a class called Candy which keeps track of the three pieces of information Read in the file line by line and create a Candy class instance for each candy bar you have, and add that new instance to a list. Once you have the list created, loop through the list and print the candy bar info out to the console Add a line of code to append one more candy bar’s information to your mycandy file. Show what your new text file looks like after the appendarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
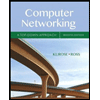
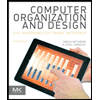
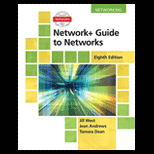
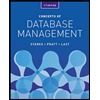
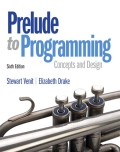
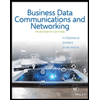