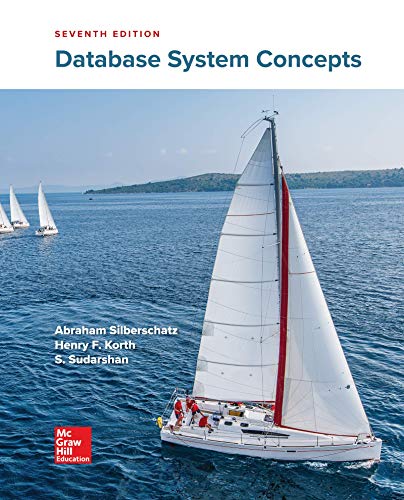
Please note global variables are not allowed to use in this assignment except of global constants.
Find Median. In statistics, the median of a set of values is the value that lies in the middle when the values are arranged in sorted order. If the set has an even number of values, then the median is taken to be the average of the two middle values. Write a C++ program that determines the median of a sorted array. In the main function,
- Declare and initialize two arrays. One array must contain an even number of values, for example {91, 71, 67, 78, 76, 82, 100, 89}. The other array must contain an odd number of values, for example {76, 71, 78, 67, 82}.
- Call a function named sortArray to sort the values in each array in ascending order. should take an array of numbers and an integer indicating the size of the array as the arguments, and sort the array in ascending order. This function does not return any value.
- Call another function named findMedian to find the median of the values in each sorted array. The function should take an array of numbers and an integer indicating the size of the array and return the median of the values in the array.
- At the end, in the main function, the program should display the sorted arrays, number of elements in the arrays, and the medians of the arrays. For example, the outputs could be:
Array numSet1 after sorting is: 67 71 76 78 82 89 91 100
It has 8 values, the median of those values is: 80
Array numSet2 after sorting is: 67 71 76 78 82
It has 5 values, the median of those values is: 76
Note: In this program, you should define two functions: sortArray, and findMedian as required. You may use the sample code in selection sort example to define sortArray function.
Debug and test your program to make sure there is no syntax and logical errors. Generate a testing report that includes at least two testing cases and testing results.

Step by stepSolved in 2 steps

- write a c++ program: Create an array with 11 integers, which will be randomly selected from the range of 0 to 100. Print the items of the array on screen as one line. Develop a function that takes the array as argument and perform these operations: -Find the minimum of array items and replace (swap) with first item of the array. -Find the maximum of array items and replace (swap) with last item of the array. -Find the average of array’s items and assign it to middle location of the array. The average of numbers should be calculated as an integer. (hint: static_cast<int>(float))arrow_forwardPlease write the following in very simple C++ code: Write a function that calculates the average of an array, where the smallest element in the array is dropped. If there are multiple copies of the smallest value, just ignore one copy. You can assume the array has at least two items in it. The function header is as follows. int getAverage (int a[], int size)arrow_forwardUse C++arrow_forward
- Declare 2D array whose size and elements must be according to the desire of user then perform the following functions on it Display a lower triangular matrix. Find the sum of an upper triangular matrix. DO IT IN C++arrow_forwardc++problemarrow_forwardPlease complete this program in C++ Please enusre that there is plenty of comments. PLENTY even if unessessary. Please include screenshots of actual code, screenshots of output, and code in the browser so I can copy and paste. Thank you.arrow_forward
- Write a C++ program : Question:Ask user to give you a list of the numbers and then Sort them, by calling two functions: Asc(Sort in Ascending order), and Desc(Sort in Descending order): Do the above question by using array. First ask user how many numbers are there in the list and then get the numbers and sort them.arrow_forwardC++arrow_forwardC++ program: Write a function that accepts an array/vector as an input and calculate the sum of all the elements in the array. This function must use recursion to achieve this. Show code: Please show all steps.arrow_forward
- Write a C++ program that reads a list of numbers from a file into an array, then uses that array to find the average of all the numbers, the average of the positive and negative numbers (0 is neither positive nor negative!), and the largest number. The point is to be able to store data in an array and do things with it Your program must contain at least the following four functions... read_list() This function will take as input parameters an array of integers and a string filename. It will open that file and read in numbers, storing them in the array, stopping at the end of the file. Remember that there are tricky issues with extra whitespace at the end of the file. You can assume the file contains only integers. The function will return the number of numbers that were read in.Note: In versions of Visual C++ pre-2010, when using the open function with a string argument, you must call the c_str() function on the string variable, such as:my_input_stream.open( filename.c_str() ) 2.…arrow_forwardProgram in C++arrow_forwardIn C++ please ! Write a Program that: Initializes an interger array size of 10 with random intergers in range [10,30]. Use a function named 'initialized_array' to acheive that. Determine if all array elements are distinct from each other or not.Use boolean function named 'distinct_elements' to achieve that function 'distinct elements, if the elements in the array are duplicate print out, elements are duplicate if not print out arrayarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
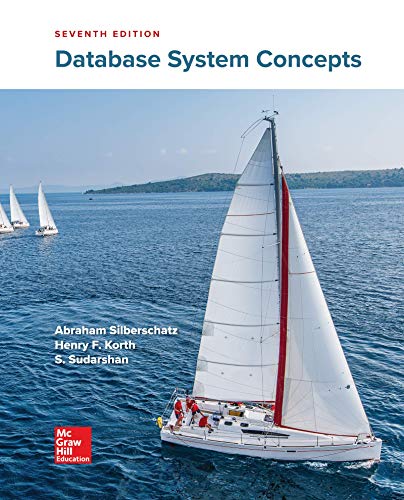
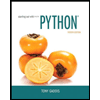
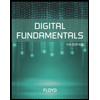
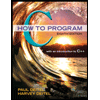
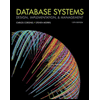
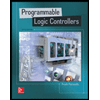