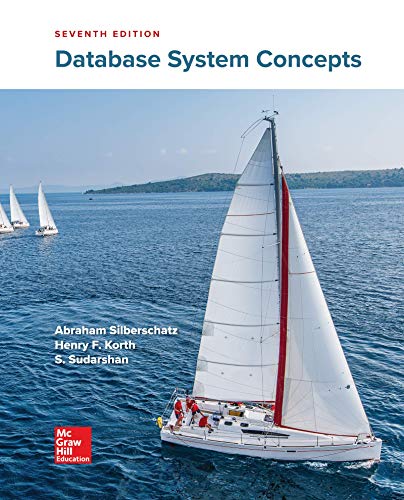
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write in C++
Write a program to declare and populate the four arrays listed below. You will be required to write the entire program, including headers and the main() function.
- distance - an array of 10 integers in increasing order starting from 5 and incrementing by one
- cities - an array of the strings “Boulder”, “NYC”, “LA", “Chicago”, and “Houston”, in that order.
- sequence - an array of the first 100 integers that are divisible by 6 in order 6, 12, 18, 24, … etc.
- letters - an array of all uppercase and lowercase characters in order, A, a, B, b, C, c, … Hint: the ASCII table will be helpful here!
In order to test that you correctly created and populated the arrays, print the values of each of their elements, in order, one element per line. Note: You are required to use a loop for distance, sequence and letters arrays. Use of individual cout or assignment statements for these three arrays will receive a 0.
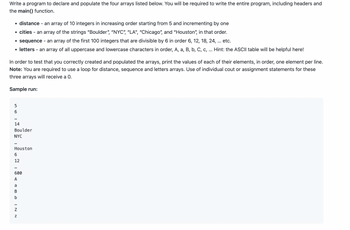
Transcribed Image Text:Write a program to declare and populate the four arrays listed below. You will be required to write the entire program, including headers and the `main()` function.
- **distance** – an array of 10 integers in increasing order starting from 5 and incrementing by one.
- **cities** – an array of the strings "Boulder", "NYC", "LA", "Chicago", and "Houston", in that order.
- **sequence** – an array of the first 100 integers that are divisible by 6 in order 6, 12, 18, 24, ... etc.
- **letters** – an array of all uppercase and lowercase characters in order, A, a, B, b, C, c, ... Hint: the ASCII table will be helpful here!
In order to test that you correctly created and populated the arrays, print the values of each of their elements, in order, one element per line.
**Note**: You are required to use a loop for distance, sequence, and letters arrays. Use of individual `cout` or assignment statements for these three arrays will receive a 0.
**Sample run:**
```
5
6
...
14
Boulder
NYC
...
Houston
6
12
...
600
A
a
B
b
...
Z
z
```
Expert Solution

arrow_forward
Step 1
We need to write a C++ code for the given scenario.
Step by stepSolved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Exercise 2 This exercise is designed to assess student’s skills of writing programs using arrays and loops. Write a C++ program using array and loop that prompts a user to enter 15 Students’ marks in ProgramDesign and Implementation (PDI) Module in an array. The program sorts the students’ marks in descending order using a loop and displays the marks of all students as well as lowest, highest, average marks, and the class result based on the average module marks. The result criteria is given below:Average is less than 40 = Poor ResultAverage is between 40 to 49 = Fair ResultAverage is less than 50 to 59= Good ResultAverage is less than 60 to 69 = Very Good ResultAverage is between 70 to 79 = Excellent ResultAverage is 80 or greater = Outstanding ResultNote: You must demonstrate the use of arrays and loops. Your code must be properly commented Sample Output:Welcome to Result Management SystemEnter PDI Marks of Student 1: 50Enter PDI Marks of Student 2: 35Enter PDI Marks of Student 3:…arrow_forwardUSING C lang: Create a simple Tic Tac Toe game designed for one player (against computer). you will need to create arrays and functions to complete this program. The first screen should ask the user for his name, print a welcome message, and display an empty 3x3 Tic Tac Toe board. Then, the program should ask the user to put the coordinates of his move. once the user enters the coordinates, the program should respond accordingly with a print of the user's move and the computer's move. this should go on until a winning pattern is found. Key steps: Game board is designed, user inputs taken and marked on the board, validate the inputs that no two inputs at the same place and out of range, check the winner/loser and complete the game.arrow_forwardC++ Do the following lab by an array(not vector) for 3 students find the average of a student by asking him how many grades do you have. The answer to this question, determine the size of the array(list of grades) and then ask the user to enter grades(the number of the grades is equal to the size of the array) then calculate the average for each student. Hence, you will calculate 3 averages. to calculate the average, use the array accumulator. Also when you get each grade validate it. validation for grade means that you check whether a grade is between 0 and 100. if the grade is negative or a grade is more than 100 then it is not valid and you have to ask the user to enter a grade again. Find the maximum and minimum grades for each student. Print the sorted list of the grades for each student. example: if grades user entered are: 60 78 98 23 45 then sorted list is:23 45 60 78 98arrow_forward
- Code in C please. Write the code that defines a struct called pilot. The following information must be in the struct (holds information about a attending flight training) - field names all capital -the last name of the person (NAME) -the first letter of the first name (INITIAL) -A double for the longest flight (LONGFLIGHT) -a list of flights for the contest series (use an array of type double size 20) -an integer for the number of flights Declare an instance of entrant and fill only the last name and initial with user input, remember to prompt.arrow_forwardTopic: Array covered in Chapter 7 Do not use any topic not covered in lecture. Write a C++ program to store and process an integer array. The program creates an array (numbers) of integers that can store up to 10 numbers. The program then asks the user to enter up to a maximum of 10 numbers, or enter 999 if there are less than 10 numbers. The program should store the numbers in the array. Then the program goes through the array to display all even (divisible by 2) numbers in the array that are entered by the user. Then the program goes through the array to display all odd (not divisible by 2) numbers in the array that are entered by the user. At the end, the program displays the total sum of all numbers that are entered by the user.arrow_forwardC++ Create a function that will read the following .txt file contents and put them into an array: "apple", "avocado", "apricot","banana", "blackberry", "blueberry", "boysenberry","cherry", "cantaloupe", "cranberry", "coconut", "cucumber", "currant","date", "durian", "dragonfruit","elderberry",arrow_forward
- Summary In this lab, you use what you have learned about searching an array to find an exact match to complete a partially prewritten C++ program. The program uses an array that contains valid names for 10 cities in Michigan. You ask the user to enter a city name; your program then searches the array for that city name. If it is not found, the program should print a message that informs the user the city name is not found in the list of valid cities in Michigan. The file provided for this lab includes the input statements and the necessary variable declarations. You need to use a loop to examine all the items in the array and test for a match. You also need to set a flag if there is a match and then test the flag variable to determine if you should print the the Not a city in Michigan. message. Comments in the code tell you where to write your statements. You can use the previous Mail Order program as a guide. Instructions Ensure the provided code file named MichiganCities.cpp is…arrow_forwardC++ Visual 2019 Write a program that uses a two identical arrays of at least 100 randomly generated integers. It should call a function that uses the bubble sort algorithm to sort one of the arrays in ascending order. The function should keep a count of the number of exchanges it makes. The program then should call a function that uses the selection sort algorithm to sort the other array. It should also keep count of the number of exchanges it makes. Display these values on the screen with appropriate descriptions.arrow_forwardC++arrow_forward
- Problem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forwardSee attached images. Please help - C++arrow_forwarduse c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
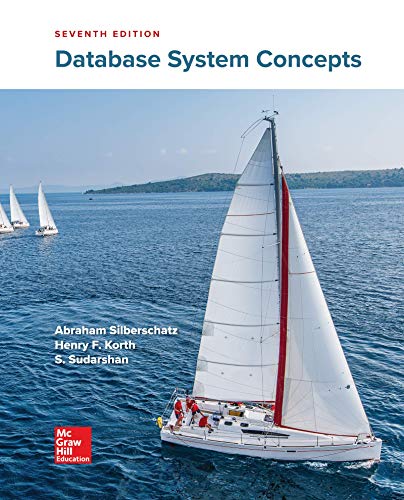
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
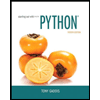
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
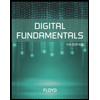
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
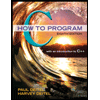
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
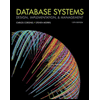
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
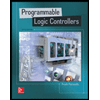
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education