Please help me writing python code based on the pseudo code and solve the problem Pseudo code for the DP global alignment algorithmDP_GLOBAL_ALIGNMENT(x, y) // Input: x, y: two amino acid sequences // Output: x', y': the two input sequences under their best global alignment, with gaps allowed/included n = length(x) m = length(y) d = 8 for k in {A, R, N, D, C, Q, E, G, H, I, L, K, M, F, P, S, T, W, Y, V} for l in {A, R, N, D, C, Q, E, G, H, I, L, K, M, F, P, S, T, W, Y, V} assign values to substitution matrix entries S(k,l) using BLOSUM50 matrix values for i = 0 to n F(i, 0) = - i*d for j = 0 to m F(0, j) = - j*d for i = 1 to n for j = 1 to m F(i, j) <— max value among F(i-1,j-1)+S(x[i], y[j]), F(i-1,j) -d, and F(i, j-1)-d Pointer(i, j) <— pointer to the cell (above-left, left, or above) that gave the max value above traceback from F(n, m), following the pointers, back to F(0,0). Record the aligned sequences x', y', with gaps, during traceback return x', y' PROBLEM Task 1.1 Write the python code for using dynamic programming to solve for the making change problem. You can use the pseudo code in lecture notes as guidance, but please feel free to modify the pseudo code according to your own design (e.g. by having subroutines instead of having all code in one function). Then, implement the algorithm in Python code. Hint: For making an array of arrays, you can check out the array function in the Python library numpy. Task 1.2 Test your code by finding the solutions for making 13, 46, and 63 cents, using the coin set containing 1-cent, 5-cent, 10-cent, and 25-cent coins. Please show me the print statements as well. This is the code that are being made but not working.
Please help me writing python code based on the pseudo code and solve the problem
Pseudo code for the DP global alignment algorithmDP_GLOBAL_ALIGNMENT(x, y)
// Input: x, y: two amino acid sequences
// Output: x', y': the two input sequences under their best global alignment, with gaps allowed/included
n = length(x)
m = length(y)
d = 8
for k in {A, R, N, D, C, Q, E, G, H, I, L, K, M, F, P, S, T, W, Y, V}
for l in {A, R, N, D, C, Q, E, G, H, I, L, K, M, F, P, S, T, W, Y, V}
assign values to substitution matrix entries S(k,l) using BLOSUM50 matrix values
for i = 0 to n
F(i, 0) = - i*d
for j = 0 to m
F(0, j) = - j*d
for i = 1 to n
for j = 1 to m
F(i, j) <— max value among F(i-1,j-1)+S(x[i], y[j]), F(i-1,j) -d, and F(i, j-1)-d
Pointer(i, j) <— pointer to the cell (above-left, left, or above) that gave the max value above
traceback from F(n, m), following the pointers, back to F(0,0). Record the aligned sequences x', y', with gaps, during traceback
return x', y'
PROBLEM
Task 1.1 Write the python code for using dynamic
Task 1.2 Test your code by finding the solutions for making 13, 46, and 63 cents, using the coin set containing 1-cent, 5-cent, 10-cent, and 25-cent coins.
Please show me the print statements as well.
This is the code that are being made but not working.
![#please write your code for Task 1.1 and Task 1.2 in this code cell.
#Task 1.1
import numpy as np.
from decimal import Decimal
def make change(coins, amount):
n = len(coins)
dp_table = np.zeros((n+1, amount+1), dtype=int)
#initialize the first column of the table.
for i in range(n+1):
dp_table[i][0] = 0
#initialize the first row of the table
for j in range(1, amount+1):
dp_table[0][j] = float('inf')
# fill up the table using dynamic programming
for i in range(1, n+1):
for j in range(1, amount+1):
if coins[i-1] <= }}
dp_table[i][j] = min(dp_table[i-1] [j], 1 + dp_table[i][j-coins[i-1]])
dp_table[i][j] = dp_table[i-1][j]
else:
#traceback and record the coins used
i = n
j = amount
coins_used= []
while i > 0 and j > 0:
if dp_table[i][j] == dp_table[i-1] [j]:
| = | − 1
else:
coins used.append(coins[i-1])
J = j - coins[i-1]
coins_used. sort()
return_dp_table[n] [amount], coins_used
#Task 1.2
from decimal import Decimal
coins = [1, 5, 10, 25]
amounts [13, 46, 63]
for amount in amounts:
num_coins, coins_used = make change(coins, amount)
print (f"For amount {amount} cents, {num_coins) coins are needed: {coins_used}")](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F96eb680b-bf96-4b4b-a2da-be1777b93ea0%2Ff1831e83-705f-407c-b4e4-4ff0e95524da%2Fw9d64h_processed.png&w=3840&q=75)
![#Task 1.2
from decimal import Decimal
coins = [1, 5, 10, 25]
amounts [13, 46, 63]
for amount in amounts:
num_coins, coins_used = make change(coins, amount)
print (f"For amount {amount} cents, {num_coins) coins are needed: {coins_used}")
OverflowError
<ipython-input-7-649474284adb> in <module>
6
7 for amount in amounts:
8
9
10
15
16
17
<i python-input-5-d53a254f3dcd> in make change(coins, amount)
# initialize the first row of the table
for j in range(1, amount+1):
00 07
Traceback (most recent call last)
18
num_coins, coins_used = make change(coins, amount)
print (f"For amount {amount} cents, {num_coins) coins are needed: {coins_used}")
dp_table[0][j] = float('inf')
19 # fill up the table using dynamic programming
OverflowError:
cannot convert float infinity to integer
SEARCH STACK OVERFLOW](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F96eb680b-bf96-4b4b-a2da-be1777b93ea0%2Ff1831e83-705f-407c-b4e4-4ff0e95524da%2Fygy25f1_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 2 images

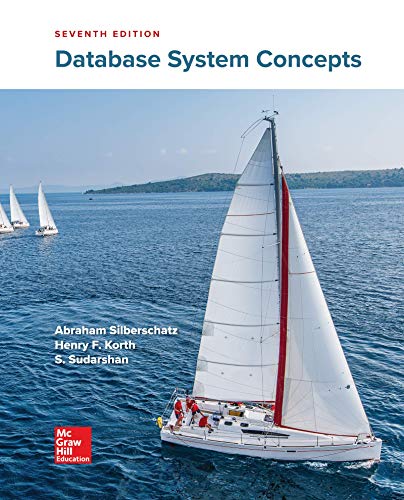
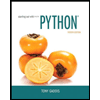
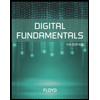
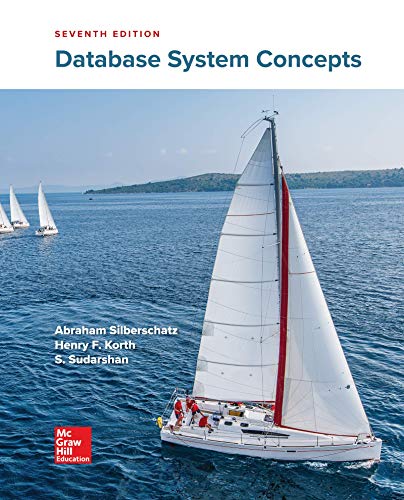
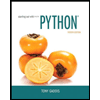
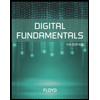
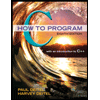
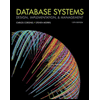
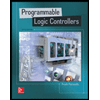