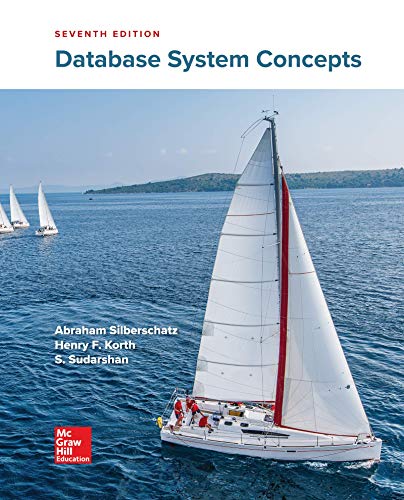
PLEASE ENSURE TO USE THE FRAMEWORK PROVIDED IN THE IMAGES, AND THAT IT WORKS WITH THE TESTER CLASS. PLEASE DONT EDIT THE TEST CLASS.
Simulate a circuit for controlling a hallway light that has switches at both ends of the hallway. Each switch can be up or down, and the light can be on or off. Toggling either switch turns the lamp on or off.
Provide methods
public int getFirstSwitchState() // 0 for down, 1 for up public int getSecondSwitchState()
public int getLampState() // 0 for off, 1 for on
public void toggleFirstSwitch()
public void toggleSecondSwitch()
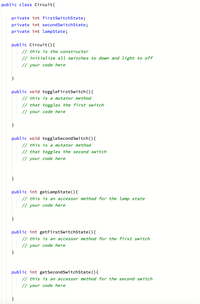
![public class CircuitTester{
public static void main(String[] args){
Circuit myCircuit = new Circuit();
// test initial state
+ myCircuit.getLampState());
+ myCircuit.getFirstSwitchState());
System.out.println("current lamp state:
System.out.println("first switch state:
%3D
System.out.println("second switch state:
+ myCircuit.getSecondSwitchState());
%3D
System.out.println();
// test first switch
System.out.println("Toggle first switch");
myCircuit.toggleFirstSwitch ();
+ myCircuit.getLampState());
+ myCircuit.getFirstSwitchState());
+ myCircuit.getSecondSwitchState ());
System.out.println("current lamp state:
System.out.println("first switch state:
System.out.println("second switch state:
%3D
System.out.println();
// test second switch
System.out.println("Toggle second switch");
myCircuit.toggleSecondSwitch();
System.out.println("current lamp state: "
+ myCircuit.getLampState());
System.out.println("first switch state:
+ myCircuit.getFirstSwitchState());
System.out.println("second switch state:
+ myCircuit. getSecondSwitchState ());
%3D
System.out.println();
// test first switch again
System.out.println("Toggle first switch");
myCircuit.toggleFirstSwitch ();
System.out.println("current lamp state:
%3D
+ myCircuit.getLampState());
+ myCircuit.getFirstSwitchState());
+ myCircuit.getSecondSwitchState ());
System.out.println("first switch state:
System.out.println("second switch state:
System.out.println();
}
}](https://content.bartleby.com/qna-images/question/123fe9bd-f5bf-404f-97ce-90ae622db155/7938ca50-1f73-4074-a60a-3986c9a5f3a9/8l2m4fr_thumbnail.png)

CODE TEXT SO YOU DON'T HAVE TO TYPE :)
public class Circuit {
private int firstSwitchState; // 0 = down, 1 = up
private int secondSwitchState; // 0 = down, 1 = up
private int lampState; // 0 = off, 1 = on
public Circuit() {
firstSwitchState = 0;
secondSwitchState = 0;
lampState = 0;
}
public void toggleFirstSwitch() {
firstSwitchState = (firstSwitchState == 1) ? (0) : (1);
lampState = (lampState == 1) ? (0) : (1);
}
public void toggleSecondSwitch() {
secondSwitchState = (secondSwitchState == 1) ? (0) : (1);
lampState = (lampState == 1) ? (0) : (1);
}
public int getLampState() {
return lampState;
}
public int getFirstSwitchState() {
return firstSwitchState;
}
public int getSecondSwitchState() {
return secondSwitchState;
}
}
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- Create a new project called Family. Add a person class with name, birthDate, and sex private instance variables. Create the public getter and setter methods for each. Create an overloaded constructor that initializes all of the instance variables. Create the default constructor. Override the toString () method to show the Person's info. Create the Person in the main method, and display that person's info. Now add a new instance variable to the Person class of type Person with the identifier spouse. Spouse should be private with setter and getter methods. If you try to instantiate spouse in the contructor, you will get a stack overflow error. Each Person, creates a Person, creates a Person, and so on forever. Try that to see the error and then remove the code from the constructor. Add the following logic: in Person there should be a method to show Spouse. If spouse is null, display or return "Not Married", otherwise call the toString() method. The should also be a get married method.…arrow_forwardPlease the code in Java eclipse. Please add comments for each instructions given in the image. I'll appreciate your help. And send the screenshoot of the output.arrow_forwardWrite a Rectangle class. Its constructor should accept length and width as a parameter. It should have methods that return its length, width, perimeter, and area. (Use Java) Show a demonstration where you create two separate rectangles.arrow_forward
- Create a Point class to hold x and y values for a point. Create methods show(), add() and subtract() to display the Point x and y values, and add and subtract point coordinates. Create another class Shape, which will form the basis of a set of shapes. The Shape class will contain default functions to calculate area and circumference of the shape, and provide the coordinates (Points) of a rectangle that encloses the shape (a bounding box). These will be overloaded by the derived classes; therefore, the default methods for Shape will only print a simple message to standard output. Create a display() function for Shape, which will display the name of the class and all stored information about the class (including area, circumference and bounding box). Build the hierarchy by creating the Shape classes Circle, Rectangle and Triangle. Search the Internet for the rules governing these shapes, if necessary. For these three Shape classes, create default constructors, as well as constructors…arrow_forwardPlease help me with my assignment for computer science as I had all the dialog box pop up for me except for "Whew" what am I doing wrong? I am including with this message the coding block for the assignment. import java.awt.event.*;import javax.swing.*;public class MouseWhisperer extends JFrame implements MouseListener {MouseWhisperer() {super("COME CLOSER");setSize(300,100);setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);addMouseListener(this);setVisible(true);}public void mouseClicked(MouseEvent e) { setTitle("OUCH"); }public void mousePressed(MouseEvent e) { setTitle("LET GO"); }public void mouseReleased(MouseEvent e) { setTitle("WHEW"); }public void mouseEntered(MouseEvent e) { setTitle("I SEE YOU"); }public void mouseExited(MouseEvent e) { setTitle("COME CLOSER"); }public static void main(String[] args) { new MouseWhisperer(); }} Please advice.arrow_forwardDraw the UML diagram for the class. Implement the class. Write a test program that creates an Account object with an account ID of 1122, a balance of $20,000, and an annual interest rate of 4.5%. Use the withdraw method to withdraw $2,500, use the deposit method to deposit $3,000, and print the balance, the monthly interest, and the date when this account was created.arrow_forward
- Write the code for the timeTick method in ClockDisplay that displays hours, minutes, and seconds, or even implement the whole class if you wish.arrow_forwardWrite a modified Button class that creates circular buttons. Call your class CButton and implement the exact same methods that are in the existing Button class. Your constructor should take the center of the button and its radius as normal parameters. Place your class in a module called cbutton . py. Test your class by modifying roller . py to use your buttons.arrow_forwardusing eclipse part 1 Create a different package session5a. Create a class SquareDemo. Create an instance of Square in the main() method with sideLength set to 10. along with output screenshot for this part. Part 2 Create 12 Square instances in SquareDemo class with random size (1-100) and print out: If divisible by 2, print out side length If divisible by 3, print out area If divisible by 6, print out side length and area Otherwise, print out "Side length and area are hidden" along with output screenshot for this part.arrow_forward
- Write a main program that displays a single frame with the title “My First Frame”. Set the size to 800 by 800. Make the frame visible. Create a panel by using the JPanel constructor and add it to the frame. Use Color.RED (a constant in the java.awt package) along with the setBackground() method in JPanel, to set the color of the panel. Add a JButton and a JLabel to the panel before adding the panel to the frame. Display the resultsarrow_forwardPythonarrow_forwardWrite in Java! Write the Widget class. a. A widget has a name and price. b. The default for name should be “widget” c. Write a constructor (not the no-args), all getters and setters. d. Add a toString method. e. Create an object of type Widget.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
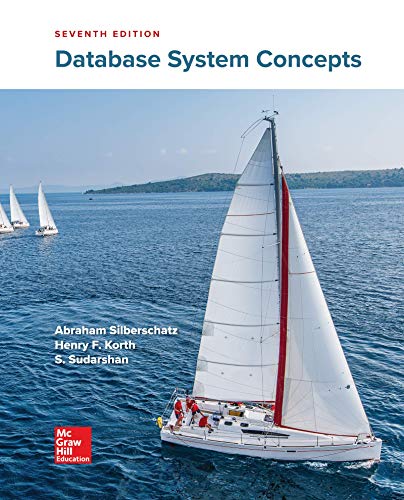
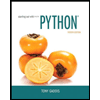
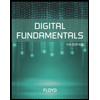
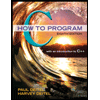
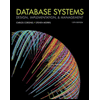
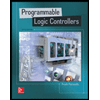