Part 2 - Syntax Errors and Troubleshooting The code below has many syntax (and other) errors. Tasks: Compile the code and fix the errors one at a time. // Lab1a.java // This short class has several bugs for practice. // Authors: Carol Zander, Rob Nash, Clark Olson, you public class Lab1a { public static void main(String[] args) { compareNumbers(); calculateDist(); } publicstatic void compareNumbers() { int firstNum = 5; int secondNum; System.out.println( "Sum is: + firstNum + secondNum ); System.out.println( "Difference is: " + (firstNum - secondNum ); System.out.println( "Product is: " + firstNun * secondNum ); } public static void calculateDistance() { int velocity = 10; //miles-per-hour int time = 2, //hoursint distance = velocity * timeSystem.out.println( "Total distance is: " distanace); } }
FIX THE CODE PROVIDED BELOW
Part 2 - Syntax Errors and Troubleshooting
The code below has many syntax (and other) errors.
Tasks:
Compile the code and fix the errors one at a time.
// Lab1a.java // This short class has several bugs for practice.
// Authors: Carol Zander, Rob Nash, Clark Olson, you
public class Lab1a {
public static void main(String[] args) {
compareNumbers();
calculateDist();
}
publicstatic void compareNumbers() {
int firstNum = 5;
int secondNum;
System.out.println( "Sum is: + firstNum + secondNum );
System.out.println( "Difference is: " + (firstNum - secondNum );
System.out.println( "Product is: " + firstNun * secondNum );
}
public static void calculateDistance() {
int velocity = 10; //miles-per-hour
int time = 2, //hoursint
distance = velocity * timeSystem.out.println( "Total distance is: " distanace);
}
}
Part 3 - Print Statements and Simple Methods
Use "print" and "println" statements, but use method calls to reduce the number of repeated "print"
and "println" statements. Seed code is provided as follows:
// Lab1b.java
// This is a practice lab to output a few verses of
//"99 bottles of beer on the wall"
// Authors: Carol Zander, Clark Olson, you
public class Lab1b {
public static void main (String[] args) {
int numBottles; // number of bottles currently on the wall
// display first verse
numBottles = 5;
System.out.print(numBottles);
onWall();
System.out.print(numBottles);
botBeer();
takeOneDown();
numBottles = 4;
System.out.print(numBottles);
onWall();
System.out.println(); // display blank line between verses
// display second verse
// [this is where you take over]
}
public static void onWall() {
System.out.println(" bottles of beer on the wall");
}
public static void botBeer() {
System.out.println(" bottles of beer");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

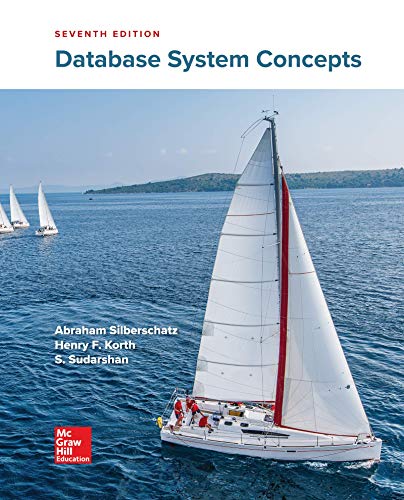
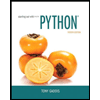
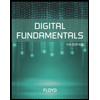
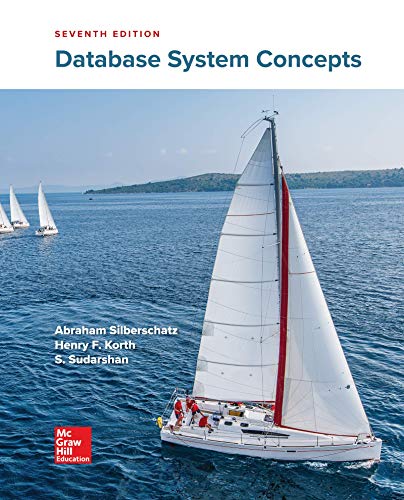
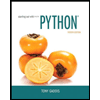
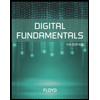
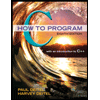
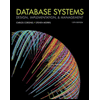
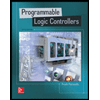