Question
P1
Suppose we want to create a method for the class BinaryTree (file BinaryTree.java) that
counts the number of times an object occurs in the tree.
a. Write the method
public int count1(T anObject)
which calls the private recursive method
private int count1(BinaryNode<T> rootNode, T anObject)
to count the number of occurrences of anObject
b. Write the method
public int count2(T anObject)
that counts the number of occurrences of anObject and that uses one of the iterators of the
binary tree.
Compare the efficiencies of the previous the two methods count1 and count2 using big O
notation. Add your answer as a comment before the function definition
Suppose we want to create a method for the class BinaryTree (file BinaryTree.java) that
counts the number of times an object occurs in the tree.
a. Write the method
public int count1(T anObject)
which calls the private recursive method
private int count1(BinaryNode<T> rootNode, T anObject)
to count the number of occurrences of anObject
b. Write the method
public int count2(T anObject)
that counts the number of occurrences of anObject and that uses one of the iterators of the
binary tree.
Compare the efficiencies of the previous the two methods count1 and count2 using big O
notation. Add your answer as a comment before the function definition
P2
Suppose we want to create a method for the class BinaryTree that decides whether two trees
have the same structure. Two trees t1 and t2 have the same structure if:
- If one has a left child, then both have left children and the left children are isomorphic,
AND
- if one has a right child, then both have right children and the right children are
isomorphic
The header of the method is:
public boolean isIsomorphic(BinaryTree<T> otherTree)
Write this method, using a private recursive method of the same name.
P3
Design an algorithm that produces a binary expression tree from a given infix expression. You
can assume that the infix expression is a string that has only the binary operators +, -, *, /,
parantheses, and one-letter operands. Implement the solution as a construction in
ExpressionTree that takes a string argument:
public ExpressionTree(String infix)
that calls the private method
private ExpressionTree formTree(String expr, int first, int last)
to construct the tree. formTree() builds the tree recursively where first and last are the first and
last index in the string corresponding to the tree you want to construc
isomorphic
The header of the method is:
public boolean isIsomorphic(BinaryTree<T> otherTree)
Write this method, using a private recursive method of the same name.
P3
Design an algorithm that produces a binary expression tree from a given infix expression. You
can assume that the infix expression is a string that has only the binary operators +, -, *, /,
parantheses, and one-letter operands. Implement the solution as a construction in
ExpressionTree that takes a string argument:
public ExpressionTree(String infix)
that calls the private method
private ExpressionTree formTree(String expr, int first, int last)
to construct the tree. formTree() builds the tree recursively where first and last are the first and
last index in the string corresponding to the tree you want to construc
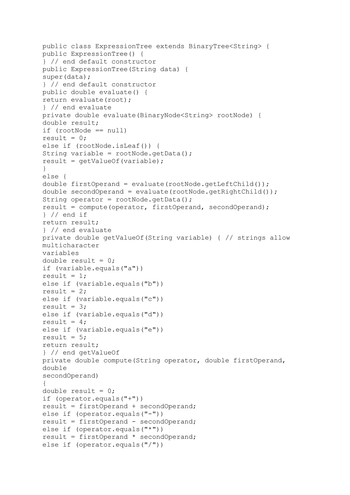
Transcribed Image Text:public class ExpressionTree extends BinaryTree<String> {
public ExpressionTree () {
} // end default constructor
public ExpressionTree (String data) {
super (data);
} // end default constructor
public double evaluate() {
return evaluate (root);
} // end evaluate
private double evaluate (BinaryNode<String> rootNode) {
double result;
null)
if (rootNode
result = 0;
else if (rootNode.isLeaf ()) {
String variable
result = getValueOf (variable);
==
}
else {
double first operand evaluate (rootNode.getLeft Child());
double secondOperand evaluate (rootNode.getRightChild());
String operator = rootNode.getData();
result = compute (operator, firstOperand, secondOperand);
} // end if
return result;
} // end evaluate
private double getValueOf (String variable) { // strings allow
=
=
=
multicharacter
variables
double result =
if (variable.equals("a"))
3;
rootNode.getData();
result = = 1;
else if (variable.equals("b"))
result = 2;
else if (variable.equals("c"))
result
else if (variable.equals("d"))
result =
4;
else if (variable.equals("e"))
result
5;
return result;
} // end getValueOf
private double compute (String operator, double firstOperand,
double
secondOperand)
0;
=
{
double result = 0;
if (operator.equals("+"))
result = firstOperand + secondOperand;
else if (operator.equals("-"))
result = firstOperand
secondOperand;
else if (operator.equals("*"))
result = firstOperand * secondOperand;
else if (operator.equals("/"))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

Knowledge Booster
Similar questions
- Please do not change any of the method signatures in either class. Implement the methods described below. RadixSort.java RadixSort.java contains two different RadixSort implementations each using a different version of Counting Sort that you will implement. As a reminder the pseudocode for CountingSort discussed in class is as follows. countingSort(arr, n, k) 1. let B[1 : n] and C[0 : k] be new arrays 2. for i = 0 to k 3. C[i] = 0 4. for j = 1 to n 5 . C[arr[j]] = C [arr[j]] + 1 6. for i = 1 to k 7. C[i] = C[i] + C[i – 1] 8. for j = n downto 1 9. B[C[arr[j]]] = arr[j] 10 C[arr[j]] = C[arr[j]] – 1 11. return B private static void countingSort2(int[] arr, int k) Now you implement almost the same method, but instead of iterating from the end of arr, you will instead iterate from the beginning. So line 8 becomes for j=1 to n. You should think about why this alternative does not impact the correctness of CountingSort. You don’t need to write this down, just think about it.…arrow_forwardWe have a parking office class for an object-oriented parking management system using java Implement the following methods for our class Add equals and hashCode methods to any class used in a List. Add a method to the Parking Office to return a collection of customer ids (getCustomerIds) Add a method to the Parking Office to return a collection of permit ids (getPermitIds) Add a method to the Parking Office to return the collection of permit ids for a specific customer (getPermitIds(Customer)) The above methods are not included in the parking office class of our class diagram. I have attached class diagrams with definitions of related classes in our system (i.e car, customer, .....)arrow_forwardQuestion 3arrow_forward
- Please answer the question in the screenshot. The language used here is in Java.arrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardGiven the doubly linked list data structure discussed in the lecture, implement a subclass “DuplicateManipuationList” that has a new function for removing duplicates froma list.- Add a new method to your DuplicateManipuationList called removeDuplicates(…). Themethod takes as an argument called ListToRemove that is a singly linked list thatcontains some values. The method should scan the linked list for each member of theListToRemove, and remove all occurrences (not only the duplicates) of that member.Besides updating the DuplicateManipulationList, the removeDuplicates(…) functionshould return a new list that shows the number of duplicates for each deleted member.Note: That with inheritance:- You have a parent class called “Node” that contain [data, *next, *prev] and a sub classcalled “DuplicateManipuationList”arrow_forward
- Complete the below function (using recursion)arrow_forwardCreate a nested class called DoubleNode that allows you to create doubly-linked lists with each node containing a reference to the item before it and the item after it (or null if neither of those items exist). Then implement static methods for the following operations: insert before a given node, insert after a given node, remove a given node, remove from a given node, insert at the beginning, insert at the end, remove from a given node, and remove a given node.arrow_forwardIn this project, you will implement a Set class that represents a general collection of values. For this assignment, a set is generally defined as a list of values that are sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. (in java)Requirements among all implementations there are some requirements that all implementations must maintain. • Your implementation should always reflect the definition of a set. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections. the sort that automatically sorts a list may not be used. Instead, when a successful addition of an element to the Set is done, you can ensure that the elements inside the ArrayList<Integer>…arrow_forward
- public class PokerAnalysis implements PokerAnalyzer { privateList<Card>cards; privateint[]rankCounts; privateint[]suitCounts; /** * The constructor has been partially implemented for you. cards is the * ArrayList where you'll be adding all the cards you're given. In addition, * there are two arrays. You don't necessarily need to use them, but using them * will be extremely helpful. * * The rankCounts array is of the same length as the number of Ranks. At * position i of the array, keep a count of the number of cards whose * rank.ordinal() equals i. Repeat the same with Suits for suitCounts. For * example, if your Cards are (Clubs 4, Clubs 10, Spades 2), your suitCounts * array would be {2, 0, 0, 1}. * * @param cards * the list of cards to be added */ publicPokerAnalysis(List<Card>cards){ this.cards=newArrayList<Card>(); this.rankCounts=newint[Rank.values().length]; this.suitCounts=newint[Suit.values().length];…arrow_forwardWrite program in javaarrow_forwardWrite a Java class DemoList, the class has:a private attribute list1 as an ArrayList of Integer.Zero argument constructor that create and initilaise list1 to an empty list.A method fill(), which take no argument and return no values. The method should allow the user to read the list of integers: user should be prompted, to enter a series of integers one at a time, until the user enters 0. Those values are used to fill list1.A method sortList() that return the sorted list1.A method brint(): that print contents of list1.A method maximum() that returns maximum of list1.A method average that returns average of list1.A method median() that return median of lsit1 as follows:If the number of elements are odd then the median is the middle one in the sorted listIf the number of elements is even, the median is the average of the middle two elements. For example: the median of 1, 2, 2, 3, 5 is 2, then middle element. The median of 1, 2, 2, 3, 4, 5, is 2.5 the average of the two middle values 2…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios