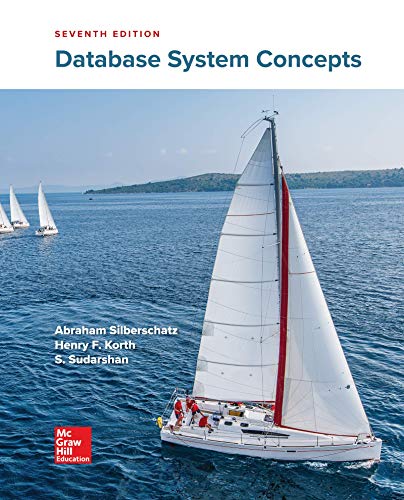
Your task is simple: use machine learning to create a model that predicts which passengers survived the Titanic shipwreck. Implement and train a classification model for the Titanic dataset (the dataset can be found here: https://www.kaggle.com/c/titanic). Please ignore the test set (i.e., test.csv) and consider the given train set (i.e., train.csv) as the dataset. What you need to do: 1. Data cleansing 2. Split the dataset (i.e., train.csv) into a training set (80% samples) and a testing set (20% samples) 3. Train your model (see details below) 4. Report the overall classification accuracies on the training and testing sets 5. Report the precision, recall, and F-measure scores on the testing set 1. Required Model (100 pts): Implement and train a logistic regression as your classification model. • You have to use Sklearn deep learning library. • You may want to refer to this tutorial: https://bit.ly/37anOxi

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Use Python Codehow to filter dataframes using python . For example I have a student grade csv table, first, I need to read the csv using pandas library then I want to visualize my student scores (using matplotlib, seaborn, etc) but I only want to visualize those whose scores are 60 and above. so how do i do the code in python.Exampe of Student grades table (csv) : Student name Score Jojo 90 Lili 65 Bibi 50 Sarah 40 Rajah 100 Albert 80 Cherry 75 Jamie 85 So , based on the table above, what doesn't need to be visualized is Bibi and Sarah . Hope you can help me :)arrow_forwardA list of projects and a list of dependencies, or a list of project pairings where the second project depends on the first project, are provided to you. Before starting a project, all of its dependencies must be built. Find a build order that enables the construction of the projects. Return an error if there is no proper construction order. EXAMPLEInput:projects: a, b, c, d, e, fdependencies: (a, d), (f, b), (b, d), (f, a), (d, c)Output: f, e, a, b, d, carrow_forwardPy .in plsarrow_forward
- Implement the transaction manager class using java Explain your code in few words. I have included other classes that have relation with the parking transaction calssarrow_forwardModify the simulate() method in the Simulator class to work with the revised interface of ParkingLot and the new peek() method in Queue. In addition, implement the getIncomingQueueSize() method of Simulator using the size() method of Queue. The getIncomingQueueSize() is going to be used in the CapacityOptimizer class (next task) to determine the size of the incoming queue after a simulation run. CODE TO MODIFY IN JAVA: public class Simulator { /** * Length of car plate numbers */ public static final int PLATE_NUM_LENGTH = 3; /** * Number of seconds in one hour */ public static final int NUM_SECONDS_IN_1H = 3600; /** * Maximum duration a car can be parked in the lot */ public static final int MAX_PARKING_DURATION = 8 * NUM_SECONDS_IN_1H; /** * Total duration of the simulation in (simulated) seconds */ public static final int SIMULATION_DURATION = 24 * NUM_SECONDS_IN_1H; /** * The probability distribution for a car leaving the lot based on the duration * that the car has been parked in…arrow_forwardYou will be using on RV related review comments to train a Sentence completion model. You could use the code below. The code is written in Jupyter notebook python. please follow the following instructions: 1. Build a working sentence completion model. Training data is provided in train.csv. This model should take partial sentences (e.g., "hey how ar," "hello, please") and provide completion for that sentence. Not every input needs to be completed into a correct sentence (e.g., "asdfauaef" does not need to have a sentence completion). For this model's purposes, a sentence is considered completed if an "end of sentence" character (like a full stop, question mark, etc.) occurs within the next five words OR. We predict up to 5 words from the input. 2. BONUS: If you use word vectors or other techniques from what we shared in class using character encoding and have a working model. 3. The test data (test.csv) will be used to evaluate the model. The test file contains partial sentences. Use…arrow_forward
- Using overload + to add total passengers and total calls and overload < to determine which taxi served most passengers. The company needs a more detailed report to be generated at the end of each shift.The sample screen output with the report is listed below: with private members taxi Id color,model, maker, passenger etc with 6 objects of class. And random selection of passenger and random selection of object. Welcome to CTC Taxi. Do you need a taxi? Y Taxi CTC0001 will pick you up in a few minutes. (Taxi CTC0001 determined that there were 3 passengers) Do you need a taxi? Y Taxi CTC0004 will pick you up in a few minutes. (Taxi CTC0004 determined that there were 5 passengers) …… …… Do you need a taxi? N CTC Taxi served a total of 124 passengers today. CTC0001 CTC00022 CTC0003 CTC0004 CTC0005 CTC0006 19 Calls 25 Calls 10 Calls 12 Calls 22 Calls 13…arrow_forwardMovie Recommendations via Item-Item Collaborative Filtering. You are providedwith real-data (Movie-Lens dataset) of user ratings for different movies. There is a readmefile that describes the data format. In this project, you will implement the item-item collab-orative filtering algorithm that we discussed in the class. The high-level steps are as follows: a) Construct the profile of each item (i.e., movie). At the minimum, you should use theratings given by each user for a given item (i.e., movie). Optionally, you can use other in-formation (e.g., genre information for each movie and tag information given by user for eachmovie) creatively. If you use this additional information, you should explain your method-ology in the submitted report. b) Compute similarity score for all item-item (i.e., movie-movie) pairs. You will employ thecentered cosine similarity metric that we discussed in class. c) Compute the neighborhood set Nfor each item (i.e. movie). You will select the moviesthat…arrow_forwardModify the GeometricObject classto implement the Comparable interface and define a static max method in theGeometricObject class for finding the larger of two GeometricObject objects.Draw the UML diagram and implement the new GeometricObject class. Writea test program that uses the max method to find the larger of two circles, the largerof two rectangles.arrow_forward
- Implement a city database using a BST to store the database records. Each database record contains the name of the city (a string of arbitrary length) and the coordinates of the city expressed as integer x- and y-coordinates. The BST should be organized by city name. Your database should allow records to be inserted, deleted by name or coordinate, and searched by name or coordinate. Another operation that should be supported is to print all records within a given distance of a specified point.Collect running-time statistics for each operation. Which operations can be implemented reasonably efficiently (i.e., in O(log n) time in the average case) using a BST? Can the database system be made more efficient by using one or more additional BSTs to organize the records by location?arrow_forwardYou are given a list of projects and a list of dependencies (which is a list of pairs ofprojects, where the second project is dependent on the first project). All of a project's dependencies must be built before the project is. Find a build order that will allow the projects to be built. If there is no valid build order, return an error.EXAMPLEInput:projects: a, b, c, d, e, fdependencies: (a, d), (f, b), (b, d), (f, a), (d, c)Output: f, e, a, b, d, carrow_forwardYou are given a list of projects and a list of dependencies (which is a list of pairs ofprojects, where the second project is dependent on the first project). All of a project's dependenciesmust be built before the project is. Find a build order that will allow the projects to be built. If thereis no valid build order, return an error.EXAMPLEInput:projects: a, b, c, d, e, fdependencies: (a, d), (f, b), (b, d), (f, a), (d, c)Output: f, e, a, b, d, carrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
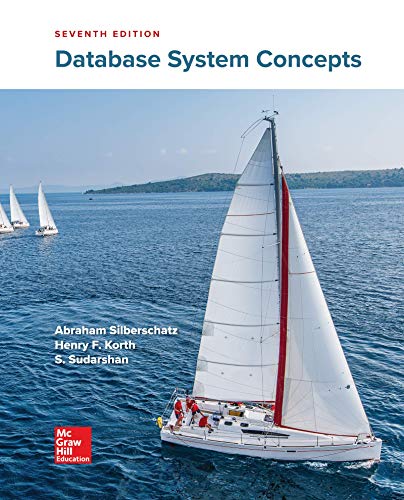
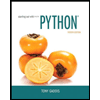
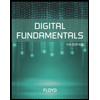
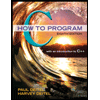
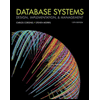
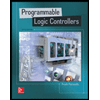