Write a Java code to implement the main functions of a simple perceptron. The target perceptron has three inputs, one output. Instances Sensor 1 Sensor 2 Sensor 3 Alarm Instance 1 Off Off On Fake Instance 2 On On On Real Instance 3 On Off On Real Instance 4 Off On On Fake This perceptron is used to analyze data from sensor inputs and, based on the analysis, it classifies alarms as true or fake. The table above shows four instances from the alarm box records and their alarm true class. These four instances will be used as a dataset to train the perceptron. After training the perceptron, it will be used to predict the alarm class for an unseen instance. Write the Java code for the following two public classes: Forward_Propagation: This class implements the forward propagation flow. The class does the following Defines the inputs and outputs as double arrays in the code double[][] inputs = new double[][]{ {0, 0, 1}, {1, 1, 1}, {1, 0, 1}, {0, 1, 1} }; double[][] outputs = new double[][]{ {0}, {1}, {1}, {0} }; Generates the initial weights and bias using Math.random(). Calculates the outputs of a given input set. Train_Test: This class runs the training loop for 1000 times. Note that the training phase includes calculating the output and then updating the bias and weights accordingly. After completing the training, it will test the following instance to see if the alarm is real or fake and prints the output on the screen Instances Sensor 1 Sensor 2 Sensor 3 Instance 5 Off Off Off After writing the function, create a main program and call the two methods in the main program. Submit a screenshot of the output along with you code. Students can import external library and use a built-in methods to calculate the sigmoid function and its derivative. it should: Calculating the sigmoid and its derivative correctly. Adjust weights correctly. Correct implementation of the Train_Test method Correct prediction of the test instance. code integration and printing out the test results at the terminal.
Write a Java code to implement the main functions of a simple perceptron. The target perceptron has three inputs, one output.
Instances |
Sensor 1 |
Sensor 2 |
Sensor 3 |
Alarm |
Instance 1 |
Off |
Off |
On |
Fake |
Instance 2 |
On |
On |
On |
Real |
Instance 3 |
On |
Off |
On |
Real |
Instance 4 |
Off |
On |
On |
Fake |
This perceptron is used to analyze data from sensor inputs and, based on the analysis, it classifies alarms as true or fake. The table above shows four instances from the alarm box records and their alarm true class. These four instances will be used as a dataset to train the perceptron. After training the perceptron, it will be used to predict the alarm class for an unseen instance.
Write the Java code for the following two public classes:
-
Forward_Propagation:
This class implements the forward propagation flow. The class does the following
-
Defines the inputs and outputs as double arrays in the code
double[][] inputs = new double[][]{
-
{0, 0, 1},
{1, 1, 1},
{1, 0, 1},
{0, 1, 1}
};
double[][] outputs = new double[][]{
-
{0},
{1},
{1},
{0}
};
-
-
Generates the initial weights and bias using Math.random().
-
Calculates the outputs of a given input set.
-
-
Train_Test:
This class runs the training loop for 1000 times. Note that the training phase includes calculating the output and then updating the bias and weights accordingly. After completing the training, it will test the following instance to see if the alarm is real or fake and prints the output on the screen
Instances |
Sensor 1 |
Sensor 2 |
Sensor 3 |
Instance 5 |
Off |
Off |
Off |
After writing the function, create a main program and call the two methods in the main program. Submit a screenshot of the output along with you code. Students can import external library and use a built-in methods to calculate the sigmoid function and its derivative.
it should:
Calculating the sigmoid and its derivative correctly.
Adjust weights correctly.
Correct implementation of the Train_Test method
Correct prediction of the test instance. |
code integration and printing out the test results at the terminal.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

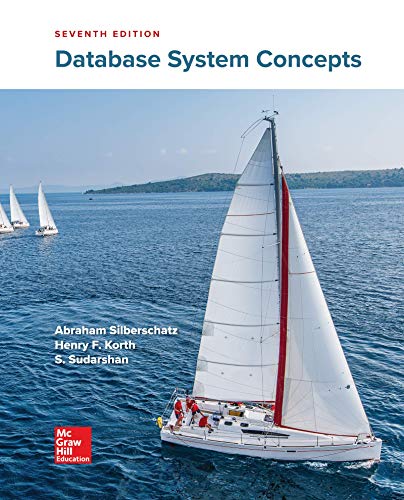
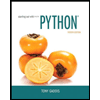
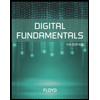
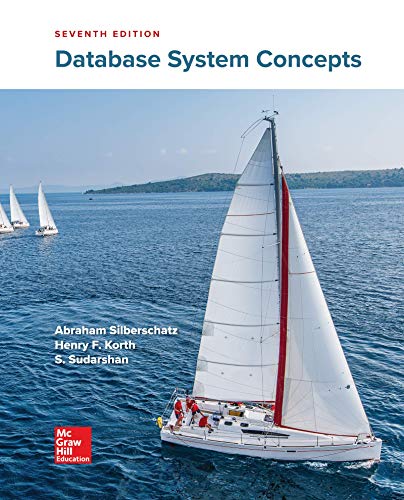
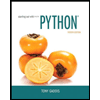
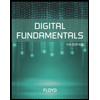
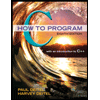
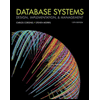
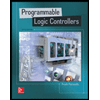