nested loops to achieve numerous repeating actions for each repeating action Practive print() function inside the loop Work with specific `end="?" parameter of print() function Instruction Assume we need to print a grid structure given the height and width.
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Python!!
Learning Objective
- Use nested loops to achieve numerous repeating actions for each repeating action
- Practive print() function inside the loop
- Work with specific `end="?" parameter of print() function
Instruction
Assume we need to print a grid structure given the height and width.
-
Create a function print_my_grid that takes height and width as parameters
1.1 Loop over the height and inside this loop, create another for loop over the width 1.2 In the 2nd loop (the loop over the width), print " * "
-
Input from the user 2 integers height and width
-
Check that both inputs are non zero positive integers. If yes:
3.1 Call print_my_grid with height and width as arguments
3.2 Otherwise, print "Invalid input, please use positive integers"
The code I should complete:
def print_my_grid(height, width)
'''Write your code here'''
pass
if __name__== "__main__":
'''Write your code here'''


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

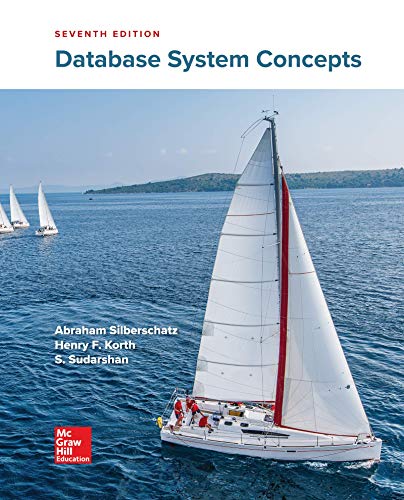
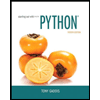
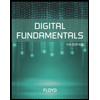
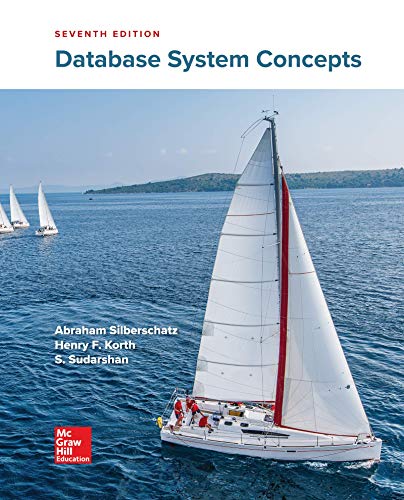
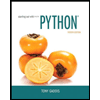
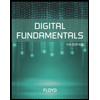
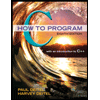
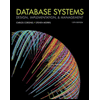
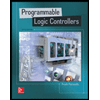