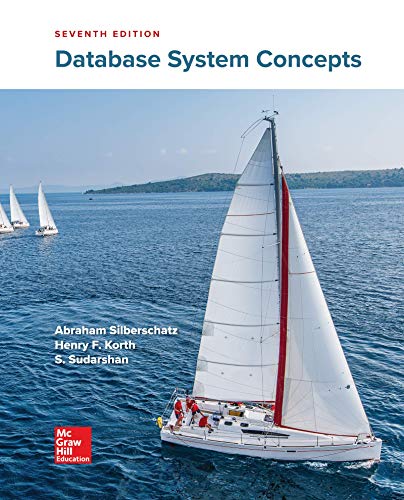
How do I complete the code to get a similar output in the image?
Fibonacci Sequence Coding using Memoization C
- Create the mfib() function to calculate Fibonacci using memoization
- Also create the initMemo() function to clear out memoization table(s) at the beginning of each mfib() run
The output of the program will record the relative amount of time required from the start of each function calculation to the end.
Note the speed difference between fib and mfib!
Turn in your commented program and a screen shot of the execution run of the program.
Do not be alarmed as the non-memoization Fibonacci calls take a long time to calculate, especially the higher values.
Be patient. It should finish.
Given Code:
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
#include <time.h>
// max number of inputs
#define MAX_FIB 200
// result of call to fib function
unsigned long long result;
//loop variable
int i;
// ========================== MEMO DEFINITIONS ==========================
unsigned long long memo[MAX_FIB]; // array to hold computed data values
bool valid[MAX_FIB]; // array to hold validity of data
// make all entries in the memoization table invalid
void initMemo() {
// ***** ADD YOUR INITIALIZATION CODE HERE *****
// ***** ADD YOUR INITIALIZATION CODE HERE *****
// ***** ADD YOUR INITIALIZATION CODE HERE *****
// ***** ADD YOUR INITIALIZATION CODE HERE *****
// ***** ADD YOUR INITIALIZATION CODE HERE *****
return;
}
// ===================== TIME DEFINITIONS ===============
// timer functions found in time.h
// time_t is time type
time_t startTime;
time_t stopTime;
// get current time in seconds from some magic date with
// t = time(NULL);
// or
// time(&t);
// where t is of type time_t
//
// get difference in secs between times (t1-t2) with
// d = difftime(t1, t2);
// where d is of type double
// ========================== NAIVE FIB =========
unsigned long long fib(int n) {
if (n < 1) {
return 0;
} else if (n == 1) {
return 1;
} else {
return fib(n-2) + fib(n-1);
}
}
// ========================== MEMOIZED FIB ==========================
unsigned long long mfib(int n) {
// ***** ADD YOUR MFIB CODE HERE *****
// ***** ADD YOUR MFIB CODE HERE *****
// ***** ADD YOUR MFIB CODE HERE *****
// ***** ADD YOUR MFIB CODE HERE *****
// ***** ADD YOUR MFIB CODE HERE *****
return 0; // replace this return with real computation
}
// ========================== MAIN PROGRAM ==========================
int main() {
for (i = 0; i < 55; i+=5) {
// get start time
time(&startTime);
// call fib
result = fib(i);
// get stop time
time(&stopTime);
printf("fib of %d = %llu\n", i, result);
printf("time taken (sec) = %lf\n\n", difftime(stopTime, startTime));
}
printf("\n\n\n");
for (i = 0; i < 90; i+=5) {
// get start time
time(&startTime);
printf("fib of %d = %llu\n", i, result);
printf("time taken (sec) = %lf\n\n", difftime(stopTime, startTime));
}
printf("\n\n\n");
for (i = 0; i < 90; i+=5) {
// get start time
time(&startTime);
// call mfib
initMemo();
result = mfib(i);
// get stop time
time(&stopTime);
printf("mfib of %d = %llu\n", i, result);
printf("time taken (sec) = %lf\n\n", difftime(stopTime, startTime));
}
return 0;
}
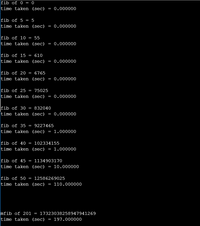

Step by stepSolved in 3 steps with 2 images

- True or false When x-coordinates are selected to create a table of values for a function, x=0 must always be used?arrow_forwardComputer Science 1st year Computer Science Abdulmuttalib T. Rashid Sheet No. 2 Q1: - Design a form and write a code to find the average value of N degrees using For Next Loop. Use InputBox function to read degrees. Q2: - Design a form and write a code to find the largest value of N numbers using Do While Loop. Use InputBox function to read numbers. Q3: - Write VB program using Do Loop While to read 7 marks, if pass in all marks (>=50) print "pass" otherwise print "fail". Use input box to read marks. Q4: - Write VB program using Do Until Loop to find the summation of student's marks, and it's average, assume the student have 8 marks. Use InputBox function to read marks. Q5: - Write VB program to read the degrees of computer science material, and find how many students are success, and how many students are failed. Use Do While Loop. Q6: - Write VB program to calculate the following equation. Use inbut box to read values of x and msgbox function to display the result values of y(x).…arrow_forwardHow do I complete the code to get a similar output in the image? Fibonacci Sequence Coding using Memoization C PROGRAM HELP: - Create the mfib() function to calculate Fibonacci using memoization - Also create the initMemo() function to clear out memoization table(s) at the beginning of each mfib() run The output of the program will record the relative amount of time required from the start of each function calculation to the end. Note the speed difference between fib and mfib! Turn in your commented program and a screen shot of the execution run of the program. Do not be alarmed as the non-memoization Fibonacci calls take a long time to calculate, especially the higher values. Be patient. It should finish. Given Code: #include <stdio.h> #include <stdlib.h> #include <stdbool.h> #include <string.h> #include <time.h> // max number of inputs #define MAX_FIB 200 // result of call to fib function unsigned long long result; //loop variable int i; //…arrow_forward
- program5_1.py Part 1Write a program that displays a table of ten distance equivalents in miles and kilometers. See Example output. You must generate the table by running a function inside a loop in main. Generate a random integer from 10 to 60, inclusive, in each loop cycle. Use this latter value as the miles argument to the function. Repeat: The function prints the table. Print the miles in a column 5 characters wide with 2 decimals and the kilometers in a column 13 characters wide with 5 decimals. Use the column formatting concepts at the end of Chapter 2, not tabs or other methods not in this course. Please include your psudocode to explain your code. Example output MILES KILOMETERS 52.00 83.68568 11.00 17.70274 40.00 64.37360 21.00 33.79614 14.00 22.53076 23.00 37.01482 48.00 77.24832 22.00 35.40548 15.00 24.14010 16.00 25.74944 program5_2.py Part 2Write another program that generates another table with the same columns and decimals…arrow_forwardCreate a string variable containing your full name (with spaces) 2) print the string as shown below 3) Create a blank 5x10 character array 4) Create a function printArray() that will print every row and column of the array 5) Create a function getNextCharacter() that gets passed the name string and returns the next non-blank character. If you have reached the end of the string then continue at the beginning. You may need to use a global variable 6) Create a function fillArray() that will fill the 2 dimensional array with the letters of your name skipping blanks. Use the above getNextCharacter() function to fill the array with only the characters in your last name. 7) Print the entire array. 8) Request a row number to print and use a sentinal loop to make sure the row number is valid. If not then request a row number again 9) Create a function printIter() that prints only the passed row using iteration 10) Create a function printRecur() that prints only the passed row using recursion…arrow_forwardWrite a flowchart and C code for a program that does the following: Declare an array that will store 9 values Use a for() statement to store user entered scores for all 9- holes Create a function and pass the array into the definition of the function to compute the total Use an accumulating total statement to compute the total score Display the overall total to the output screen so that the golfer can see if he won.arrow_forward
- Fibonacci Sequence Coding using Memoization C PROGRAM HELP: - Create the mfib() function to calculate Fibonacci using memoization - Also create the initMemo() function to clear out memoization table(s) at the beginning of each mfib() run The output of the program will record the relative amount of time required from the start of each function calculation to the end. Note the speed difference between fib and mfib! Turn in your commented program and a screen shot of the execution run of the program. Do not be alarmed as the non-memoization Fibonacci calls take a long time to calculate, especially the higher values. Be patient. It should finish. Given Code: //C program #include <stdio.h>#include <stdlib.h>#include <stdbool.h>#include <string.h>#include <time.h> // max number of inputs#define MAX_FIB 200 // result of call to fib functionunsigned long long result; //loop variableint i; // ========================== MEMO DEFINITIONS ==========================…arrow_forward[CelsiusTemperature Table] The formula for converting a temperature from Fahrenheit to Celsius is C = 5/9 * (F - 32) where F is the Fahrenheit temperature and C is the Celsius temperature.Write a function named celsius that accepts two Fahrenheit temperatures (low and high) as arguments. The function will print a table list the Fahrenheit temperatures from low to high and the corresponding Celsuis temperatures. What is the function prototype? (assume only integers used for the temperate) void celsius (int, int); How to implement this function? The function will use a loop to convert the Fahrenheit temperatures to Celsius temperatures and display the values in the console. In the driver program, prompt for the user input for the low and high Fahrenheit temperatures. Call the the function to display the result in the console. Requirements: Use only for-loop for this exercise; Print the output in a table format Include the function prototypes before the main() All functions…arrow_forward# Create a function to find area of the rectangle """ Area of rectangle is calculated by length (l) * breadth (b) The above function should take the value for l , b The function should display the area when function is called """ def area(l,b): # you code will go in herearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
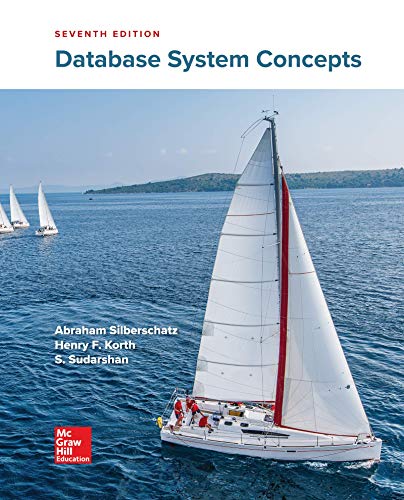
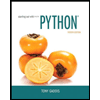
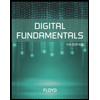
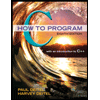
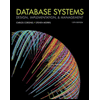
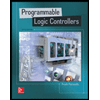