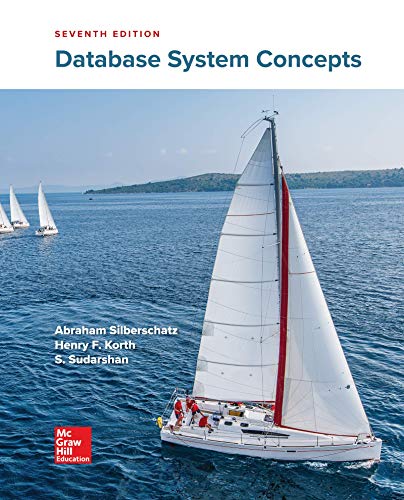
Need help solving this issue.
ShoppingList Class
import java.util.Scanner;
import java.util.LinkedList;
public class ShoppingList {
public static void main (String[] args) {
Scanner scnr = new Scanner(System.in);
// TODO: Declare a LinkedList called shoppingList of type ListItem
LinkedList<String> shoppingList = new LinkedList<String>();
String item;
// TODO: Scan inputs (items) and add them to the shoppingList LinkedList
// Read inputs until a -1 is input
item = scnr.next();
while (!item.equals("-1")) {
shoppingList.add(item);
item = scnr.next();
}
// TODO: Print the shoppingList LinkedList using the printNodeData() method
for (int i = 0; i < shoppingList.size(); i ++) {
ListItem list = new ListItem();
list.listItem();
list.printNodeData();
}
}
}
ListItem Class
public class ListItem {
private String item;
public ListItem() {
item = "";
}
public ListItem(String itemInit) {
this.item = itemInit;
}
// Print this node
public void printNodeData() {
System.out.println(this.item);
}
}
ListItem Class
public class ListItem {
private String item;
public ListItem() {
item = "";
}
public ListItem(String itemInit) {
this.item = itemInit;
}
// Print this node
public void printNodeData() {
System.out.println(this.item);
}
}
I am able to add the items to the shopping list but I have been stuck on trying to print the list using the printNodeData method that is in the ListItem class.
Current Error Mesage on Run
ShoppingList.java:29: error: cannot find symbol
list.listItem();
^
symbol: method listItem()
location: variable list of type ListItem
1 error

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- import java.util.*; public class Main{ public static void main(String[] args) { Main m = new Main(); m.go(); } private void go() { List<Stadium> parks = new ArrayList<Stadium>(); parks.add(new Stadium("PNC Park", "Pittsburgh", 38362, true)); parks.add(new Stadium("Dodgers Stadium", "Los Angeles", 56000, true)); parks.add(new Stadium("Citizens Bank Park", "Philadelphia", 43035, false)); parks.add(new Stadium("Coors Field", "Denver", 50398, true)); parks.add(new Stadium("Yankee Stadium", "New York", 54251, false)); parks.add(new Stadium("AT&T Park", "San Francisco", 41915, true)); parks.add(new Stadium("Citi Field", "New York", 41922, false)); parks.add(new Stadium("Angels Stadium", "Los Angeles", 45050, true)); Collections.sort(parks, Stadium.ByKidZoneCityName.getInstance()); for (Stadium s : parks) System.out.println(s); }}…arrow_forwardJAVA plese Implement the indexOf method in the LinkedIntegerList class public int indexOf(int value); /** * Returns whether the given value exists in the list. * @param value - value to be searched. * @return true if specified value is present in the list, false otherwise. */ } public static void main(String[] args) { // TODO Auto-generated method stub SimpleIntegerListADT myList = null; System.out.println(myList); for(int i=2; i<8; i+=2) { myList.add(i); } System.out.println(myList.indexOf(44));arrow_forwardRedesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forward
- Consider the following class map, class map { public: map(ifstream &fin); void print(int,int,int,int); bool isLegal(int i, int j); void setMap(int i, int j, int n); int getMap(int i, int j) const; int getReverseMapI(int n) const; int getReverseMapJ(int n) const; void mapToGraph(graph &g); bool findPathRecursive(graph &g, stack<int> &moves); bool findPathNonRecursive1(graph &g, stack<int> &moves); bool findPathNonRecursive2(graph &g, queue<int> &moves); bool findShortestPath1(graph &g, stack<int> &bestMoves); bool findShortestPath2(graph &, vector<int> &bestMoves); void map::printPath(stack<int> &s); int numRows(){return rows;}; int numCols(){return cols;}; private: int rows; // number of latitudes/rows in the map int cols; // number of longitudes/columns in the map matrix<bool> value; matrix<int> mapping; // Mapping from latitude and longitude co-ordinates (i,j) values to node index…arrow_forwardPROBLEM STATEMENT: Return a sublist of a given list from the range of the two integerinputs from, to. public class FindSublistFromRange{public static ArrayList<Integer> solution(ArrayList<Integer> elms, int from, int to){// ↓↓↓↓ your code goes here ↓↓↓↓return new ArrayList<>();} Can you help me with this question The language is Java Please use the code i provided above to answer this questionarrow_forwardimport java.util.Scanner; public class Playlist { // TODO: Write method to ouptut list of songs public static void main (String[] args) { Scanner scnr = new Scanner(System.in); SongNode headNode; SongNode currNode; SongNode lastNode; String songTitle; int songLength; String songArtist; // Front of nodes list headNode = new SongNode(); lastNode = headNode; // Read user input until -1 entered songTitle = scnr.nextLine(); while (!songTitle.equals("-1")) { songLength = scnr.nextInt(); scnr.nextLine(); songArtist = scnr.nextLine(); currNode = new SongNode(songTitle, songLength, songArtist); lastNode.insertAfter(currNode); lastNode = currNode; songTitle = scnr.nextLine(); } // Print linked list…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
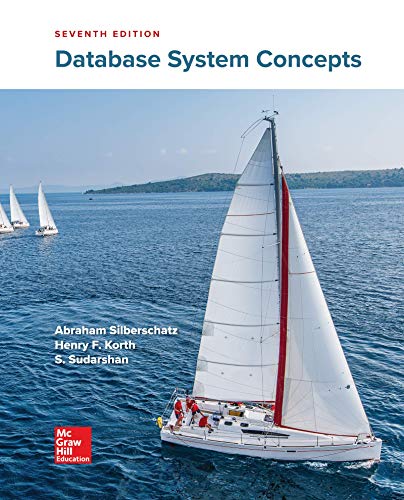
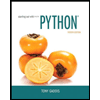
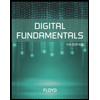
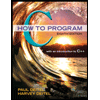
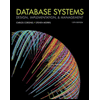
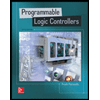