Need help making a java file that combines both linearSearch and binarySearch •Both search methods must use the Comparable interface and the compareTo() method. •Your program must be able to handle different data types, i.e., use generics. •For binarySearch, if you decide to use a midpoint computation formula that is different from the textbook, explain that formula briefly as a comment within your code.
Need help making a java file that combines both linearSearch and binarySearch
•Both search methods must use the Comparable<T> interface and the compareTo() method.
•Your program must be able to handle different data types, i.e., use generics.
•For binarySearch, if you decide to use a midpoint computation formula that is different from
the textbook, explain that formula briefly as a comment within your code.
//code from textbook
//linearSearch
public static <T>
boolean linearSearch(T[] data, int min, int max, T target) {
int index = min;
boolean found = false;
while (!found && index <= max) {
found = data [index].equals(target);
index++;
}
return found;
}
//binarySearch
public static <T extends Comparable<T>>
boolean binarySearch(T[] data, int min, int max, T target) {
boolean found = false;
int midpoint = (min + max)/2;
if (data[midpoint].compareTo(target)==0)
found = true;
else if(data[midpoint].compareTo(target)>0) {
if(min<=midpoint-1)
found = binarySearch(data, min, midpoint-1, target);
}
else if (midpoint+1<=max)
found=binarySearch(data, midpoint+1, max, target);
return found;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

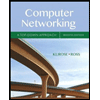
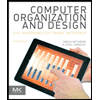
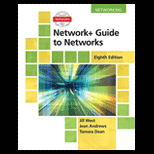
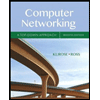
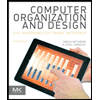
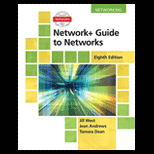
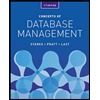
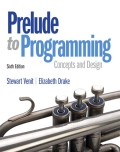
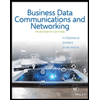