JUST NEED EXECUTION CHARTTT
C++ Language
Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks
Sample Execution Chart Template
//your header files
// constant size of the array
// declare an array of BankAccount objects called accountsArray of size =SIZE
// comments for other declarations with variable names etc and their functionality
// function prototypes
// method to fill array from file, details of input and output values and purpose of function
void fillArray (ifstream &input,BankAccount accountsArray[]);
// method to find the largest account using balance as key
int largest(BankAccount accountsArray[]);
// method to find the smallest account using balance as key
int smallest(BankAccount accountsArray[]);
// method to display all elements of the accounts array
void printArray(BankAccount accountsArray[]);
int main() {
// function calls come here:
// give the function call and a comment about the purpose,
// and input and return value if any
//open file
//fill accounts array
//print array
//find largest
//display the largest
//find smallest
//display the smallest
//find duplicates and display if found
}
CODE:
Maincpp:
#include <iostream>
#include <fstream>
#include "BankAccount.h"
using namespace std;
const int SIZE = 8;
// function declaration for array
void fillArray (ifstream &input,BankAccount accountsArray[]);
int largest(BankAccount accountsArray[]);
int smallest(BankAccount accountsArray[]);
void printArray(BankAccount accountsArray[]);
BankAccount removeDuplicate(BankAccount account1, BankAccount account2);
int main()
{
//open file
ifstream input;
input.exceptions(ifstream::failbit | ifstream::badbit);
//string line;
try{
input.open("BankData.txt"); // provide full path to file
BankAccount accountsArray[SIZE];
//fill accounts array
fillArray(input,accountsArray);
input.close();
//print array
printArray(accountsArray);
//find largest
cout<<"Largest Balance: "<<endl;
cout<<accountsArray[largest(accountsArray)].toString()<<endl;
//find smallest
cout<<"Smallest Balance :"<<endl;
cout<<accountsArray[smallest(accountsArray)].toString()<<endl;
//find duplicates and print if necessary
bool duplicates = false;
for(int i=0;i<SIZE-1;i++)
{
for(int j=i+1;j<SIZE;j++)
{
accountsArray[j] = removeDuplicate(accountsArray[i],accountsArray[j]);
if(accountsArray[j].getAccountName() == "XXXX XXXX")
duplicates = true;
}
}
if(duplicates)
{
cout<<"\nDuplicate Account Found : Reprinting List"<<endl;
printArray(accountsArray);
}
}catch(ifstream::failure &e)
{
cerr<<"Error while opening/reading the input file"<<endl;
}
return 0;
}
void fillArray (ifstream &input,BankAccount accountsArray[]){
string firstName, lastName;
int accountId;
int accountNumber;
double accountBalance;
int count = 0;
string line;
while((!input.eof()) && (count < SIZE))
{
input>>firstName>>lastName>>accountId>>accountNumber>>accountBalance;
BankAccount account(firstName+" "+lastName,accountId,accountNumber,accountBalance);
accountsArray[count] = account;
count++;
}
}
int largest(BankAccount accountsArray[]){
int max = 0;
for(int i=1;i<SIZE;i++)
{
if(accountsArray[i].getAccountBalance() > accountsArray[max].getAccountBalance())
max = i;
}
return max;
}
int smallest(BankAccount accountsArray[]){
int min = 0;
for(int i=1;i<SIZE;i++)
{
if(accountsArray[i].getAccountBalance() < accountsArray[min].getAccountBalance())
min = i;
}
return min;
}
BankAccount removeDuplicate(BankAccount account1, BankAccount account2){
if(account1.equals(account2))
{
return(BankAccount("XXXX XXXX",0,0,0));
}else
return account2;
}
void printArray(BankAccount accountsArray[]){
cout<<" FAVORITE BANK - CUSTOMER DETAILS "<<endl;
cout<<" --------------------------------"<<endl;
for(unsigned int i=0;i<SIZE;i++)
{
cout<<accountsArray[i].toString()<<endl<<endl;
}
}
.h and Bankcaccountcpp are in sc shots
I cant provide more pics because if limitations so I had to merge them please download the pic if its not visible enough. overall I just need an execution chart



This is very simple.
An execution chart is simply a flowchart. Here I have shown the complete execution chart for the given problem statement.
Note: The diagram is not scaled but the concept for the solution is accurate.
Step by step
Solved in 2 steps with 1 images

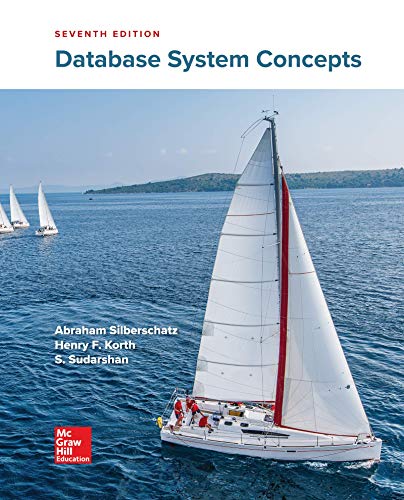
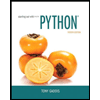
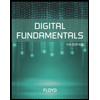
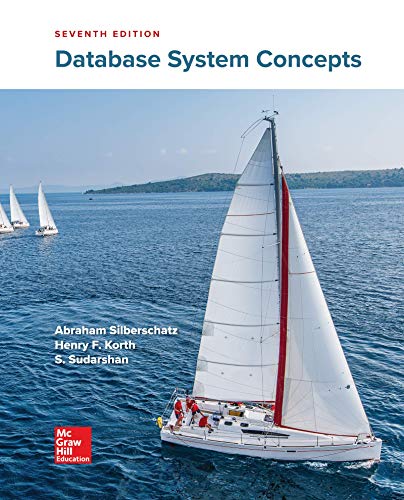
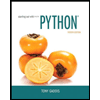
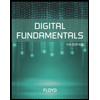
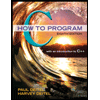
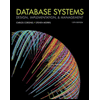
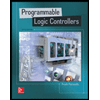