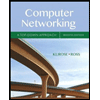
My question is for C programming.
Write a complete program by using four helper functions The whole requested parts should be written in one single program. For all the functions, use the same prototype. Do not hard code any value, all the values must be given by the user in the whole code.
The first function is getting a string and prints the given string, use the same given prototype:
void myname ( char firstlastname[ ]);
when you test the program, you will call the function in the main, the input will be your name ( you will prompt the user) and the functions prints it completely.
The second function which you will add to the program will take 3 integer values and return the average all the 3 integer values, use the same prototype which is given here:
double ave ( int num1, int num2, int num3);
Call the function in the main, ask the user for 3 values.
Test case for 3 given values by the user: 3 5 2
The third function will accept an array of numbers and prints it in the reverse order.
void ptr_r (int arr[ ]);
for testing this part you will call the function in the main with array int test[5];
you will get values from the user, and the function ptr_r, prints that in the reverse order.
User input for the test case is:
100 200 0 -99 22
And after the call it shows:
22 -99 0 200 100
The fourth function will find the max value in the array test[5], which user added before.
int maxi( int arr[ ]);
based on the given values from the user, when you call the function, it returns 200.
At the end of the code, print the number of the characters, that you have added for the string ( your name) with the use of a function of string.h library.
You should get one screenshot which shows all the functions and requested options work.

Step by stepSolved in 2 steps with 1 images

- C++ Need help with the functions While testing I am not getting the count for the vowles and consontants. Please jelp and test each option. Write a function that accepts a C-string as its argument. The function should count the number of vowels appearing in the string and return that number. Write another function that accepts a C-string as its argument. This function should count the number of consonants appearing in the string and return that number.Demonstrate the two functions in a program that performs the following steps:1. The user is asked to enter a string.2. The program displays the following menu: A) Count the number of vowels in the string B) Count the number of consonants in the string C) Count both the vowels and consonants in the string D) Enter another string E) Exit the program3. The program performs the operation selected by the user and repeats until the user selects E, to exit the program. // Sharissa Sullivan //Febuary 2 2023// Chapter 10 Vowels and…arrow_forwardWrite in C++ Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five parameters and inserts the name of a Pokemon right after a specific index. Function specifications Name: insertAfter() Parameters (Your function should accept these parameters IN THIS ORDER): input_strings string: The array containing strings num_elements int: The number of elements that are currently stored in the array arr_size int: The number of elements that can be stored in the array index int: The location to insert a new string. Note that the new string should be inserted after this location. string_to_insert string: The new string to be inserted into the array Return Value: bool: true: If the string is successfully inserted into the array false: If the array is full If the index value exceeds the size of the arrayarrow_forwardWrite a function in JAVA language that accepts three integer parameters and returns true if two or more of them (integers) have the same rightmost digit. The integers are non-negative. Inside the main function test the function for at least two test cases.arrow_forward
- Given a string which is basically a sentence without spaces between words. However, the first letter of every word is in uppercase. You need to print this sentence after the following amendments: (i) Put a single space between these words (ii) Convert the uppercase letters to lowercase. Note: The first character of the string can be both uppercase/lowercase. Write a C++ function that takes a string and then performs the mentioned amendments and returns a string.arrow_forwardIn C++: Write a function which will find the average of all elements of the sequence: {2, 4, 6, 8, 10, 12}. Use call by reference, and return the average as the function's return value.arrow_forwardin c++arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
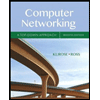
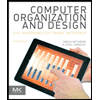
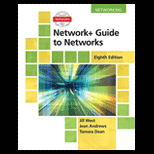
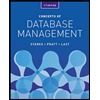
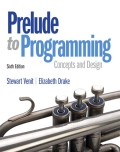
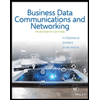