Modify the provided starter code by completing the two methods, evalAS(String e) and evalMD(String e), adding the ability to evaluate arithmetic expressions with + and – or * and / operators and multi-digit integer operands to the program. An example method has been written for you in the starter code that does + and – operations with single digit operands. Use that example to come up with your code to complete the other two methods. Test your program with the math expressions provided in the comments of the starter code. Then try other longer valid expressions you come up with for testing. You can assume that the provided expressions do not have spaces or other characters in them other than valid math operators ( +, - , *, /) and integers. You can also assume that all expressions begin with a positive integer. import java.util.Scanner; public class Lab9{ //if e = "1-2+3+4-5", return 1. //if e = "9", return 9 //convert //'0' -> 0, //'1' -> 1, // //'9' -> 9 //this method assumes a positive number to start public static int evalAS1(String e) { int r = e.charAt(0)-'0'; for (int i = 1; i < e.length(); i+=2) if (e.charAt(i) == '+') r += e.charAt(i+1)-'0'; else r -= e.charAt(i+1)-'0'; return r; } //add a method here for multi-digit numbers using addition and subtraction only //if e = "123-24+3+467-50", return 519 public static int evalAS(String e){ //add your code here } //add a method here for multi-digit numbers using multiply and division only //integer division is being used here so 123/24 = 5 in the example below //if e = "123/24*3*467/50", return 140 public static int evalMD(String e) { //add your code here } public static void main(String[] args) { Scanner input = new Scanner(System.in); int choice; String again; do{ System.out.println("Enter your math expression."); String problem = input.nextLine(); System.out.println("1. My expression contains single digit numbers with addition and subtraction."); System.out.println("2. My expression contains multi-digit numbers with only addition and subtraction."); System.out.println("3. My expression contains multi-digit numbers with only multiplication and division."); System.out.println("4. Exit."); System.out.println("Pick an option from the menu."); choice = input.nextInt(); input.nextLine(); switch(choice){ case 1: System.out.println("The answer is "+evalAS1(problem)); break; case 2: System.out.println("The answer is "+evalAS(problem)); break; case 3: System.out.println("The answer is "+evalMD(problem)); break; case 4: System.out.println("Goodbye!"); System.exit(0); default: System.out.println("You entered an invalid menu option."); System.out.println("Please try again."); } System.out.println("Would you like to enter a new expression?"); again=input.nextLine(); }while(again.toLowerCase().charAt(0)!='n'); } }
Modify the provided starter code by completing the two methods, evalAS(String e) and
evalMD(String e), adding the ability to evaluate arithmetic expressions with + and – or * and /
operators and multi-digit integer operands to the program. An example method has been written for
you in the starter code that does + and – operations with single digit operands. Use that example to
come up with your code to complete the other two methods.
Test your program with the math expressions provided in the comments of the starter code. Then try
other longer valid expressions you come up with for testing. You can assume that the provided
expressions do not have spaces or other characters in them other than valid math operators ( +, - , *, /)
and integers. You can also assume that all expressions begin with a positive integer.
import java.util.Scanner;
public class Lab9{
//if e = "1-2+3+4-5", return 1.
//if e = "9", return 9
//convert
//'0' -> 0,
//'1' -> 1,
//
//'9' -> 9
//this method assumes a positive number to start
public static int evalAS1(String e) {
int r = e.charAt(0)-'0';
for (int i = 1; i < e.length(); i+=2)
if (e.charAt(i) == '+')
r += e.charAt(i+1)-'0';
else
r -= e.charAt(i+1)-'0';
return r;
}
//add a method here for multi-digit numbers using addition and subtraction only
//if e = "123-24+3+467-50", return 519
public static int evalAS(String e){
//add your code here
}
//add a method here for multi-digit numbers using multiply and division only
//integer division is being used here so 123/24 = 5 in the example below
//if e = "123/24*3*467/50", return 140
public static int evalMD(String e) {
//add your code here
}
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int choice;
String again;
do{
System.out.println("Enter your math expression.");
String problem = input.nextLine();
System.out.println("1. My expression contains single digit numbers with addition and subtraction.");
System.out.println("2. My expression contains multi-digit numbers with only addition and subtraction.");
System.out.println("3. My expression contains multi-digit numbers with only multiplication and division.");
System.out.println("4. Exit.");
System.out.println("Pick an option from the menu.");
choice = input.nextInt();
input.nextLine();
switch(choice){
case 1:
System.out.println("The answer is "+evalAS1(problem));
break;
case 2:
System.out.println("The answer is "+evalAS(problem));
break;
case 3:
System.out.println("The answer is "+evalMD(problem));
break;
case 4:
System.out.println("Goodbye!");
System.exit(0);
default:
System.out.println("You entered an invalid menu option.");
System.out.println("Please try again.");
}
System.out.println("Would you like to enter a new expression?");
again=input.nextLine();
}while(again.toLowerCase().charAt(0)!='n');
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

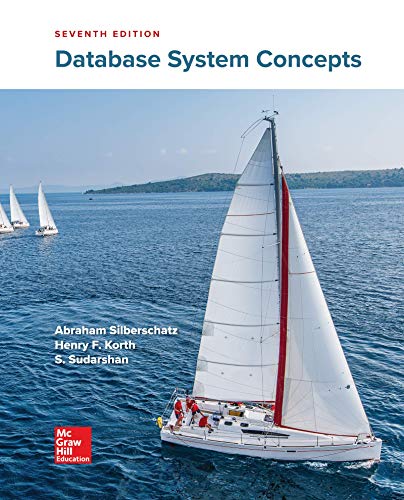
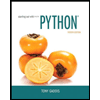
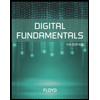
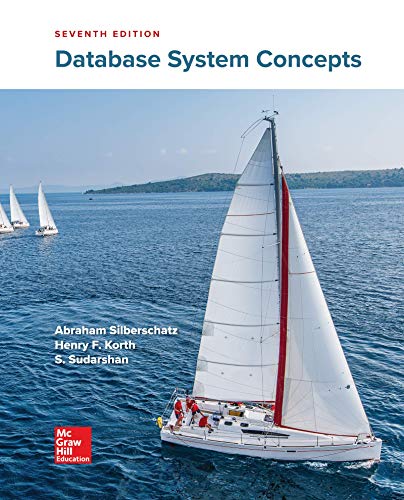
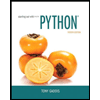
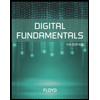
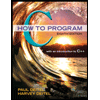
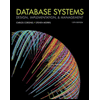
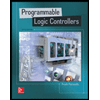