Enhance the “Random Letter Compare” program from the previous exercise, to make it a Letter Guessing Game. Modifications, - let the first random letter be the computer’s pick of random letter that the user must guess and let the guess be from A…Z (all 26 letters) - remove the display of the random letter (but see below, TESTING and DEBUGGING) - let the second letter be input from the user, so prompt the user for a guess: "Guess which letter: " o to convert console input from String to a char, consider using: guessLetter = scan.next().toUpperCase().charAt(0); // return input character - compare the user’s character against the computer’s pick, to evaluate the correct result: o if the user guessed correctly, display, “You guessed correctly!” o else, if the user’s guess is before the computer’s pick, display, “Oh, too bad, the letter is after.” o else, the user’s guess must be after the computer’s pick, so display, “Oh, too bad, the letter is before.” Run your program to test all possible results. TESTING and DEBUGGING: - to help yourself while testing (since there are so many letters), display the computer’s pick, but comment it out to really play the game - if the computer pick is commented (so it doesn’t display), consider reducing the number of letters (A..D, instead of A..Z) to help making guessing easier 3. Further enhance the Guessing Game program so that it repeats to give the user up to 10 guesses. Modifications, - declare a constant variable to store the maximum number of guesses: static final int MAXGUESSES = 10; (declare this above and outside the main() ) - declare an int variable to count the number of guesses, (which is incremented (++) inside the loop) - after the statement for the computer’s pick, add a loop (while, for, or even do_while) surrounding the user’s input and evaluation statements, o ask the user for a guess letter o increment (++) the guesses counter inside the loop o the condition for the loop is based on both: a) guesses counter hasn’t reach MAXGUESSES iterations, AND b) user’s guess doesn’t match the computer’s pick o display the number of iterations (counter); or the number of remaining iterations (10 – counter) - after the loop, check if the user did not guess correctly, display, “The correct letter is #” (where # is the computer’s pick)
Enhance the “Random Letter Compare”
Modifications,
- let the first random letter be the computer’s pick of random letter that the user must guess
and let the guess be from A…Z (all 26 letters)
- remove the display of the random letter (but see below, TESTING and DEBUGGING)
- let the second letter be input from the user, so prompt the user for a guess: "Guess which letter: "
o to convert console input from String to a char, consider using:
guessLetter = scan.next().toUpperCase().charAt(0); // return input character
- compare the user’s character against the computer’s pick, to evaluate the correct result:
o if the user guessed correctly, display,
“You guessed correctly!”
o else, if the user’s guess is before the computer’s pick, display,
“Oh, too bad, the letter is after.”
o else, the user’s guess must be after the computer’s pick, so display,
“Oh, too bad, the letter is before.”
Run your program to test all possible results.
TESTING and DEBUGGING:
- to help yourself while testing (since there are so many letters), display the computer’s pick, but comment it out to
really play the game
- if the computer pick is commented (so it doesn’t display), consider reducing the number of letters (A..D, instead of A..Z)
to help making guessing easier
3. Further enhance the Guessing Game program so that it repeats to give the user up to 10 guesses.
Modifications,
- declare a constant variable to store the maximum number of guesses: static final int MAXGUESSES = 10;
(declare this above and outside the main() )
- declare an int variable to count the number of guesses, (which is incremented (++) inside the loop)
- after the statement for the computer’s pick, add a loop (while, for, or even do_while) surrounding the user’s input and
evaluation statements,
o ask the user for a guess letter
o increment (++) the guesses counter inside the loop
o the condition for the loop is based on both: a) guesses counter hasn’t reach MAXGUESSES iterations, AND
b) user’s guess doesn’t match the computer’s pick
o display the number of iterations (counter); or the number of remaining iterations (10 – counter)
- after the loop, check if the user did not guess correctly, display,
“The correct letter is #” (where # is the computer’s pick)

Step by step
Solved in 2 steps with 1 images

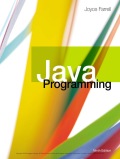
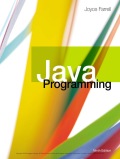