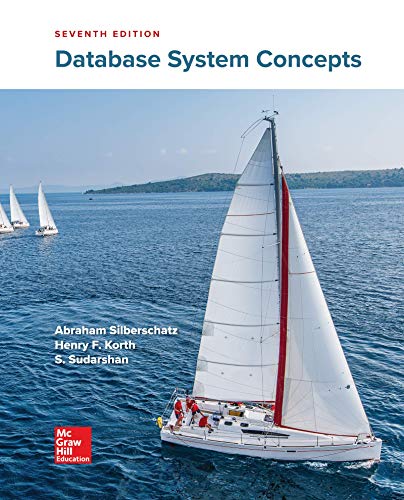
Modify the Date class below by adding a new method called nextDay() that increments the Date by 1 when called and returns a new Date object. This method should properly increment the Date across Month boundary (i.e from the last day of the month to the first day of the next month).
//Date class declaration
public class Date {
private int month; // 1-12
private int day; // 1-31 based on month
private int year; // any year
private static final int [] daysPerMonth =
{0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
//constructor: confirm proper value for month and day given the year
public Date (int month, int day, int year) {
//check if month in range
if (month <=0 II month > 12) {
throw new IllegalArgumentException(
“month (“ + month + “) must be 1-12”);
}
//check if day in range for month
if (day <= 0 II
(day > daysPerMonth[month] && ! (month == 2 && day == 29))) {
Throw new IllegalArgumentException(”day (“ + day +
“) out-of-range for the specified month and year”);
}
//check for leap year if month is 2 and day is 29
if (month == 2 && day == 29 && !(year % 400 ==0 II
(year % 4 == 0 && year % 100 ! = 0 ))) {
throw new IllegalArgumentException(“day (“ + day +
“) out-of-range for the specified month and year”);
}
this.month = month;
this.day = day;
this.year = year;
System.out.printf(“Date object constructor for date %s%n”, this);
}
//return a String of the form month/day/year
public String toString() {
return String.format(“%d/%d/%d”, month, day, year):
}
}
Write a program called DateTest that asks the user to enter 3 numbers (one at a time) corresponding to Month, Day and Year. The first two will be 2 digits(Month and Day) and 3rd will be 4 digits(Year). Read the numbers and create a corresponding Date object. Write a loop that increments this Date 3 times by calling nextDay()and displays each Date in the format mm/dd/yyyy on the console. You MUST use nextDay() to generate the dates. You CANNOT HARD CODE the output.
Your program should work for any valid data that user enters. (Assume that the user will enter VALID month,day and year numbers).
You can assume that all dates will be in the same year 2020.
Example:
If user enters 07 30 and 2020. The following should be displayed:
07/30/2020
07/31/2020
08/01/2020
Upload 2 Java files: Date.java and DateTest.java.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Javaarrow_forwardPYTHON CLASSES AND OBJECTarrow_forwardWrite a Circle class that has the following field: • radius: a double The class should have the following methods: no-arg constructor constructor: accepts the radius of the circle as an argument. setRadius: A mutator method for the radius field. getRadius: An accessor method for the radius field. getArea: returns the area of the circle. getDiameter: returns the diameter of the circle. getCircumference: returns the circumference of the circle Write a program that demonstrates the use of the Circle class by asking the user for the circle's radius, creating a Circle object, and then reporting the circle's area, diameter, and circumference.arrow_forward
- There is a class hierarchy that includes three classes: class Animal { protected int age; public int getAge() { return age; } public void setAge(int age) { this.age = age; }}class Pet extends Animal { protected String name; public String getName() { return name; } public void setName(String name) { this.name = name; }}class Cat extends Pet { protected String color; public String getColor() { return color; } public void setColor(String color) { this.color = color; }} Given the following object: Pet cat = new Cat(); Select all invalid method invocations.arrow_forwardjava Assignment Description: Write a class named Car that has the following fields: The yearModel field is an int that holds the car’s year model. The make field references a String object that holds the make of the car. The speed field is an int that holds the car’s current speed. In addition, the class should have the following constructor and other methods. The constructor should accept the car’s year and make as arguments. These values should be assigned to the object’s yearModel and make fields. The constructor should also assign 0 to the speed field. Constructor: The constructor should accept no arguments. Constructor: The constructor should accept the car’s year as argument.Assign the value to the object’s yearModel. No need to assign anything to the make and assign 0 to speed. Appropriate mutator methods should set the values in an object’s yearModel, make, and speed fields. Appropriate accessor methods should get the values stored in an object’s yearModel, make, and…arrow_forwardGiven the following class definition: class employee{public: employee(); employee(string, int, double); employee(int, double); employee(string); void setData(string, int, double); void print() const; void updatePay(double); int getNumOfServiceYears() const; double getPay() const;private: string name; int numOfServiceYears; double pay;}; Create a class parttime derived from the above class that includes private members payRate and hoursWorked of type double along with a constructor and the definition of a print function that prints out all information from the base and derived class. Provide a test program that would demonstrate the working parttime class. Note: You can assume all code is stored in a single file.arrow_forward
- helparrow_forwardpublic class Artwork { // TODO: Declare private fields - title, yearCreated // TODO: Declare private field artist of type Artist // TODO: Define default constructor // TODO: Define get methods: getTitle(), getYearCreated() // TODO: Define second constructor to initialize // private fields (title, yearCreated, artist) // TODO: Define printInfo() method // Call the printInfo() method in Artist.java to print an artist's information }} public class ArtworkLabel { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userTitle, userArtistName; int yearCreated, userBirthYear, userDeathYear; userArtistName = scnr.nextLine(); userBirthYear = scnr.nextInt(); scnr.nextLine(); userDeathYear = scnr.nextInt(); scnr.nextLine(); userTitle = scnr.nextLine(); yearCreated =…arrow_forwardJava A class always has a constructor that does not take any parameters even if there are other constructors in the class that take parameters. Choose one of the options:TrueFalsearrow_forward
- Write a member method for the Team class named : calculatePercentage that it will calculate and return the winning percentage of a team. The winning percentage is calculated as follows: wins / ( wins + losses)arrow_forwardpublic class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forwardSubclass toString should call include super.toString(); re-submit these codes. public class Vehicle { private String registrationNumber; private String ownerName; private double price; private int yearManufactured; Vehicle [] myVehicles = new Vehicle[100]; public Vehicle() { registrationNumber=""; ownerName=""; price=0.0; yearManufactured=0; } public Vehicle(String registrationNumber, String ownerName, double price, int yearManufactured) { this.registrationNumber=registrationNumber; this.ownerName=ownerName; this.price=price; this.yearManufactured=yearManufactured; } public String getRegistrationNumber() { return registrationNumber; } public String getOwnerName() { return ownerName; } public double getPrice() { return price; } public int getYearManufactured() { return yearManufactured; }…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
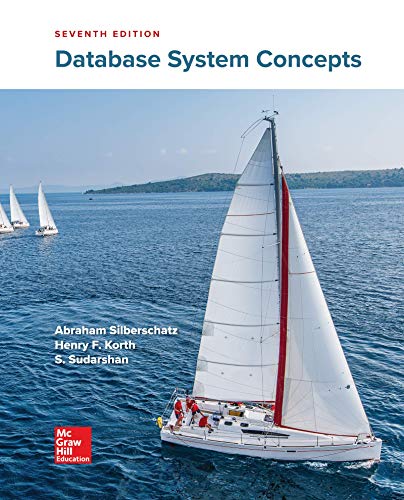
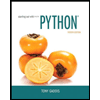
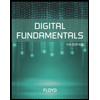
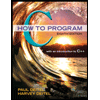
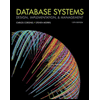
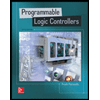