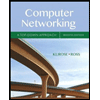
modify task6.c to create a tool that remove those files from the current working directory. You can use strstr() function to search for .bak, or .old in entry->d_name.
Modify this program to clean up .bak .old files.
*/
#include <stdio.h>
#include <dirent.h>
#include <string.h>
#include <errno.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd.h>
#include <time.h>
int main(int argc, char *argv[])
{
if(argc!=1)
return 1;
char *path = "."; //current working directory
DIR *dir = opendir(path);
if(dir==NULL){
fprintf(stderr, "Cannot open dir %s: %s\n", path, strerror(errno));
return 1;
}
struct dirent *entry;
while((entry=readdir(dir))!=NULL){
printf("%ld %s\n", entry->d_ino, entry->d_name);
}

Step by stepSolved in 3 steps with 2 images

- Plz solve assignment 5 by using assignment 8, use c programming and plz don't use any other libraries other than stdio . harrow_forwardPlease answer as soon as possible using python as the programming language.arrow_forwardHW1 Instructions: Your homework must have a cover page contains homework number, your name and student id. Submit the code on word file or pdf file. Write your name and student id as comment in the code (see the example). 回HW1 * HW1.Program B// Name: Your name // ID : Your university ID Busing System; using System.Collections. Generic; using System. Linq; using System. Text; using System.Threading. Tasks; 6. 7. 8. 10 Bnamespace HW1 11 O references class Program { 12 13 re static void Main(string[] args) Submit screenshot after running the code for each part (The screenshot must have your name and ID, see the example). ON CAWINDOWS\system32\cmd.exe Name: Your name ID : Your university ID MENU 1. Show grades 2. Show average 3. Max grade 4. Min grade 5. Modify a grade 6. Search by ID 0. Exit Please enter your choice: Write a menu program in C# that manages students grades for one class. The program lets the user select from a list of options, and if the input is not one of the options,…arrow_forward
- Before starting this question, first, download the data file pokemonTypes.txt from the class Moodle. Make sure to put it in the same folder as your a5.py python code. Write a function called read_pokedata() that takes as parameter(s): • a string indicating the name of a pokemon type data file This function should return a database (i.e. a dictionary-of-dictionaries) that stores all of the Pokemon data in a format that we will describe further below. You can review section 11.1.9 of the text for a refresher on what databases look like using dictionaries. Input File Format The data file for this question looks like this: bulbasaur,grass,South America ivysaur,grass,Asia,Antarctica Each line of the file contains all of the data for a single pokemon. The first item on the line is always the pokemon's name; names are guaranteed to be unique. The second item is always the pokemon's type, which in the example above, is the type grass. Following that are one or more continents where that…arrow_forwardFocus on string operations and methods You work for a small company that keeps the following information about its clients: • first name • last name • a 5-digit user code assigned by your company. The information is stored in a file clients.txt with the information for each client on one line (last name first), with commas between the parts. For example Jones, Sally,00345 Lin ,Nenya,00548 Fule,A,00000 Smythe , Mary Ann , 00012 Your job is to create a program assign usernames for a login system. First: write a function named get_parts(string) that will that will receive as its arguments a string with the client data for one client, for example “Lin ,Nenya,00548”, and return the separate first name, last name, and client code. You should remove any extra whitespace from the beginning and newlines from the end of the parts. You’ll need to use some of the string methods that we covered in this lesson You can test your function by with a main() that is just the function call with the…arrow_forward* Question Completion Status: QUESTION 11 Wite a program (mymv infile outfile) to rename a infile as outfile using file system calls, link(), and unlink() For the toolbar, press ALT+F10 (PC) or ALT+FN+F10 (Mac). BIUS Paragraph Arial 10pt P. QUESTION 12 Write a program that takes the file name as the command line argument and outputs the size of the file in byte the file) !! !!!arrow_forward
- Filename: runlength_decoder.py Starter code for data: hw4data.py Download hw4data.py Download hw4data.pyYou must provide a function named decode() that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this:def decode(data : list) -> list: Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run. For example, using Python lists, the initial list: [‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’] would be encoded as: ['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2] So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the…arrow_forward1. Create a file that contains 20 integers or download the attached file twenty-integers.txttwenty-integers.txt reads:12 20 13 45 67 90 100 34 56 89 33 44 66 77 88 99 20 69 45 20 Create a program that: 2. Declares a c-style string array that will store a filename. 3. Prompts the user to enter a filename. Store the file name declared in the c-string. 4. Opens the file. Write code to check the file state. If the file fails to open, display a message and exit the program. 5. Declare an array that holds 20 integers. Read the 20 integers from the file into the array. 6. Write a function that accepts the filled array as a parameter and determines the MAXIMUM value in the array. Return the maximum value from the function (the function will be of type int). 7. Print ALL the array values AND print the maximum value in the array using a range-based for loop. Use informational messages. Ensure the output is readable.arrow_forwardPlease Help ASAP!!!arrow_forward
- When I run this code i get an error that says. Traceback (most recent call last):File "C:\Users\ept20\OneDrive\Desktop\student_file1.py", line 49, in <module>stu_info.add_students(name, number, email)AttributeError: 'Studentinfo' object has no attribute 'add_students' import pathlib class Studentinfo:def __init__(self):self.student_list = [] def save_students(self):"""Saving the information to the record file"""record_file = pathlib.Path("c://users//ept20//onedrive//Desktop//student_file.txt")with record_file.open(mode='a', encoding='utf-8') as file:for info in self.student_list:name, number, email = infofile.write(f'{name},{number},{email}\n') def add_students(self,name,number,email):"""Add a student to the student list"""student_info = (name, number, email)self.student_list.append(student_info) class Student:def __init__(self, name, number, email):self.name = nameself.number = numberself.email = emailprint(f'A new student object: {self.name} is created')def…arrow_forwardThis is using basic C and Linux System Callsarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
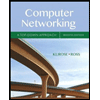
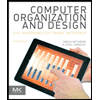
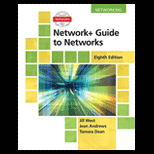
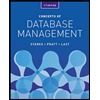
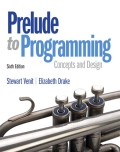
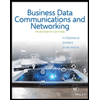