Modify task5.c so it will print out a content of a file backward. You will need to use lseek() to accomplish this task. Modify this program to print the file backward. */ #include #include #include int main(int argc, char *argv[]) { char *filename = argv[1]; int input = open(filename, O_RDONLY); char c; while(1){ int r = read(input,&c,1); if(r==0) break; write(1,&c, 1); } close(input); return 0; }
Modify task5.c so it will print out a content of a file backward. You will need to use lseek() to accomplish this task. Modify this program to print the file backward. */ #include #include #include int main(int argc, char *argv[]) { char *filename = argv[1]; int input = open(filename, O_RDONLY); char c; while(1){ int r = read(input,&c,1); if(r==0) break; write(1,&c, 1); } close(input); return 0; }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Modify task5.c so it will print out a content of a file backward. You will need to use lseek() to accomplish this task.
Modify this program to print the file backward.
*/
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
char *filename = argv[1];
int input = open(filename, O_RDONLY);
char c;
while(1){
int r = read(input,&c,1);
if(r==0)
break;
write(1,&c, 1);
}
close(input);
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
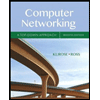
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
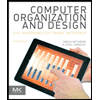
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
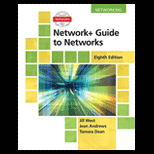
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
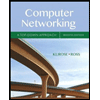
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
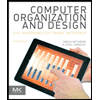
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
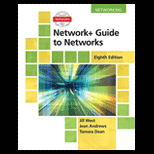
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
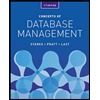
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
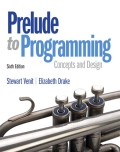
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
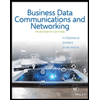
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY