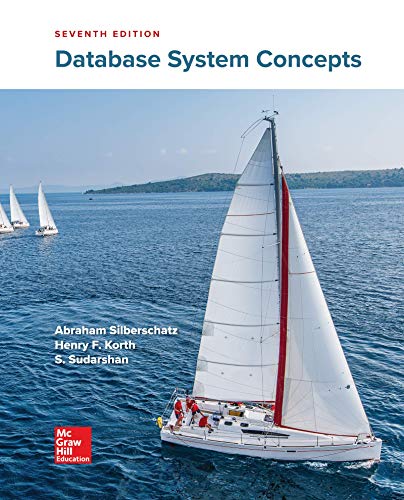
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Microsoft Visual C# debugging exercise 5-2 for chapter 5.
This is my code but it gave me an error. It likes an infinitive loop. What did I do wrong, please?
// Program asks user to enter a stock number
// If the stock number is not 209, 312, or 414 the user must reenter the number
// The program displays the correct price
using System;
using static System.Console;
using System.Globalization;
class DebugFive2
{
static void Main()
{
const string ITEM209 = "209";
const string ITEM312 = "312";
const string ITEM414 = "414";
const double PRICE209 = 12.99;
const double PRICE312 = 16.77;
const double PRICE414 = 109.07;
double price=0;
string stockNum;
Write("Please enter the stock number of the item you want ");
stockNum = ReadLine();
while(stockNum != ITEM209 || stockNum != ITEM312 || stockNum != ITEM414)
{
WriteLine("Invalid stock number. Please enter again. ");
stockNum = ReadLine();
}
if(stockNum!=null && stockNum==ITEM209)
{
price = PRICE209;
stockNum = ReadLine();
}
else
if(stockNum!=null && stockNum==ITEM312)
{
price = PRICE312;
stockNum = ReadLine();
}
else
if(stockNum!=null && stockNum==ITEM414)
{
price = PRICE414;
stockNum = ReadLine();
}
WriteLine("The price for item # {0} is {1}", stockNum, price.ToString("C", CultureInfo.GetCultureInfo("en-US")));
}
}
Expert Solution

arrow_forward
Step 1
// Hi Buddy, You have done a fabulous job but made a mistake at one point i.e. in while loop, you have tested with || ( OR Operator), so if any of the condition passes, the loop will execute, so even if you put correct stock number then also, one condition will fail, while other two will be passed so our loop will execute continuously.
// It is highly recommended to use && ( And operator ) instead of || operator.
while(stockNum != ITEM209 && stockNum != ITEM312 && stockNum != ITEM414)
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Create an object-oriented program that allows you to enter data for customers and employees. Console Customer/Employee Data Entry Customer or employee? (c/e): c DATA ENTRY First name: Frank Last name: Wilson Email: frank44@gmail.com Number: M10293 CUSTOMER Name: Frank Wilson Email: frank44@gmail.com Number: M10293 Continue? (y/n): y Customer or employee? (c/e): e DATA ENTRY First name: joel Last name: murach Email: joel@murach.com SSN: 123-45-6789 EMPLOYEE Name: Joel Murach Email: joel@murach.com SSN: 123-45-6789 Continue? (y/n): n Bye! Specifications ● ● Create a Person class that provides attributes for first name, last name, and email address. This class should provide a property or method that returns the person's full name. Create a Customer class that inherits the Person class. This class should add an attribute for a customer number. Create an Employee class that inherits the Person class. This class should add an attribute for a social security number (SSN). The program should…arrow_forwardarrow_back Starting Out With Visual C# (5th Edition) 5th Edition Chapter 11, Problem 1PP arrow_back_ios PREVIOUS NEXT arrow_forward_ios Question share_out_linedSHARE SOLUTION Chapter 11, Problem 1PP Program Plan Intro Employee and ProductionWorker Classes Program plan: Design the form: Place a three text boxes control on the form, and change its name and properties to get the employee name, number, and hourly pay rate from the user. Place a four label boxes control on the form, and change its name and properties. Place a two radio buttons control on the form, and change its name and properties. Place a one group box control on the form, and change its name and properties. Place a command button on the form, and change its name and properties to retrieve the object properties and then display the values into label box. In code window, write the code: Program.cs: Include the required libraries. Define the namespace “Program11_1”. Define a class “Program”. Define a constructor for the…arrow_forwardWrite program in C++ Language The other solutions on chegg were incorrect! Please read carefully! Write a program that defines and tests a class called COP3014. The class resembles aclassroom. It has the following grading scheme:3 quizzes 20 points each 20%Mid term 100 points 30%Final 100 points 50%Based on the total grade of any student for the course, the letter grade of each student can becomputed as follows:Any grade of 90 or more is an A, any grade of 80 or more (but less than 90) is a B, any grade of70 or more (but less than 80) is a C, any grade of 60 or more (but less than 70) is a D, and anygrade below 60 is an F.This class needs to have some member variables. The first name, last name, the Z-number, thegrades, the total grade and final letter grade are all considered to be private member variablesof this class.The class should also have the following public member functions/procedural attributes that canbring the objects (students of the class) to life and give them some…arrow_forward
- CSCI 140/L Java Project: Menu-Driven System Part A Write a menu-driven program that will give the user the three choices: 1) Wage calculator, 2) Coupon Calculator, and 3) Exit. Class Name: PartA Wage Calculator: For the wage calculator, prompt for the name and hourly pay rate of an employee. Here the hourly pay rate is a floating-point number, such as $9.25. Then ask how many hours the employee worked in the past week. Be sure to accept fractional hours. Compute the pay. Any overtime work (over 40 hours per week) is paid at 150 percent of the regular wage (1.5 the hourly pay rate). Print the employee's name, regular hours worked, regular hours pay, overtime hours worked (do not show overtime hours, if there are none), overtime hours pay (do not show overtime pay if there is none), and total pay. [Do not prompt for overtime hours] Coupon Calculator: For the coupon calculator, the total coupon amount is calculated based on the type of items purchased. Ask for the shopper's total purchase…arrow_forwardComputer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwardI need help with this PLEASE NO JAVA NO C++ ONLY PYTHON PLZ Create a class object with the following attributes and actions: Class Name Class number Classroom Semester Level Subject Actions: Store a class list Print the class list as follows: Class name Class Number Semester Level Subject Test your object: Ask the user for all the information and to enter at least 3 classes test using all the actions of the object print using the to string action Describe the numbers and text you print. Do not just print numbers or strings to the screen explain what each number represents.arrow_forward
- Asking help for Java Programming Create a program that simulates a meeting reservation system. Part 1: Basic requirements The program shall allow the user to select from the following options: Create a new meeting Show meetings on the calendar Clear all meetings Each meeting has a subject, start day/time and end day/time Subject is a short text description of the meeting Day is a date that contains month, day, and year Meeting times need only deal with hour and minute When the user wants to create a new meeting, the program asks for the subject, start and end day/times for it and adds it to the calendar For the basic requirements, meetings are not allowed to overlap. If a meeting the user wants to schedule overlaps with an existing meeting, the program presents an error message showing which meeting the one the user wants to schedule overlaps with When the user wants to show all meetings for the week, the report displays all meetings each day as follows Show all meetings…arrow_forwardIn C# using System; using static System.Console; using System.Globalization; class JobDemo4 { static void Main() { RushJob[] jobs = new RushJob[5]; int x, y; double grandTotal = 0; bool goodNum; for(x = 0 ; x < jobs.Length; ++x) { jobs[x] = new RushJob(); Write("Enter job number "); jobs[x].JobNumber = Convert.ToInt32(ReadLine()); goodNum = true; for(y = 0; y < x; ++y) { if(jobs[x].Equals(jobs[y])) goodNum = false; } while(!goodNum) { Write("Sorry, the job number " + jobs[x].JobNumber + " is a duplicate. " + "\nPlease reenter "); jobs[x].JobNumber = Convert.ToInt32(ReadLine()); goodNum = true; for(y = 0; y < x; ++y) { if(jobs[x].Equals(jobs[y])) goodNum = false; } }…arrow_forwardstructions: Write the following programs in C# using concepts learnt in this chapter and abmit the .cs file with the screenshot of your output for each question in the Lab Assignment 2 Submission page. 1. FRENCH TRANSLATOR Look at the following list of French words and their meanings: In French: gauche milieu droite middle In English: right left Create an application that translates the French words to English. The form should have three buttons, one for each French word. When the user clicks a button, the application should display the English translation in a Label control.arrow_forward
- public static class Lab2{public static void Main(){int idNum;double payRate, hours, grossPay;string firstName, lastName;// prompt the user to enter employee's first nameConsole.Write("Enter employee's first name => ");firstName = Console.ReadLine();// prompt the user to enter employee's last nameConsole.Write("Enter employee's last name => ");lastName = Console.ReadLine()// prompt the user to enter a six digit employee numberConsole.Write("Enter a six digit employee's ID => ");idNum = Convert.ToInt32(Console.ReadLine());// prompt the user to enter the number of hours employeeworkedConsole.Write("Enter the number of hours employee worked =>");// prompt the user to enter the employee's hourly pay rateconsole.Write("Enter employee's hourly pay rate: ");payRate = Convert.ToDouble(Console.ReadLine());// calculate gross paygrossPay = hours * payRate;// output resultsConsole.WriteLine("Employee {0} {1}, (ID: {2}) earned {3}",firstName, lastName, idNum,…arrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forwardWrite in c#arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
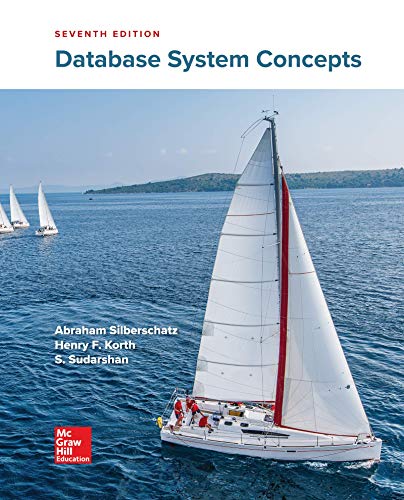
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
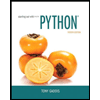
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
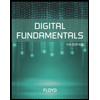
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
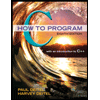
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
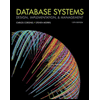
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
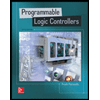
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education