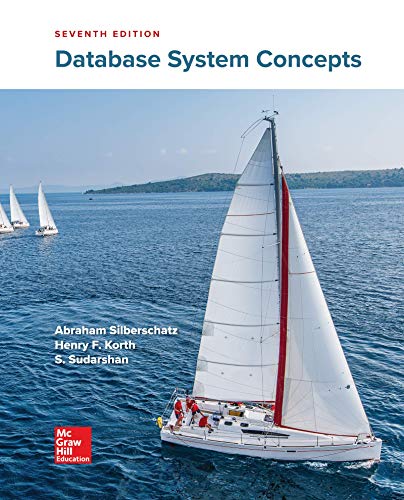
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
In C#
using System;
using static System.Console;
using System.Globalization;
class JobDemo4
{
static void Main()
{
RushJob[] jobs = new RushJob[5];
int x, y;
double grandTotal = 0;
bool goodNum;
for(x = 0 ; x < jobs.Length; ++x)
{
jobs[x] = new RushJob();
Write("Enter job number ");
jobs[x].JobNumber = Convert.ToInt32(ReadLine());
goodNum = true;
for(y = 0; y < x; ++y)
{
if(jobs[x].Equals(jobs[y]))
goodNum = false;
}
while(!goodNum)
{
Write("Sorry, the job number " +
jobs[x].JobNumber + " is a duplicate. " +
"\nPlease reenter ");
jobs[x].JobNumber = Convert.ToInt32(ReadLine());
goodNum = true;
for(y = 0; y < x; ++y)
{
if(jobs[x].Equals(jobs[y]))
goodNum = false;
}
}
Write("Enter customer name ");
jobs[x].Customer = ReadLine();
Write("Enter description ");
jobs[x].Description = ReadLine();
Write("Enter estimated hours ");
jobs[x].Hours = Convert.ToDouble(ReadLine());
}
WriteLine("\nSummary:\n");
for(x = 0; x < jobs.Length; ++x)
{
WriteLine(jobs[x].ToString());
grandTotal += jobs[x].Price;
}
WriteLine("\nTotal for all jobs is " + grandTotal.ToString("C", CultureInfo.GetCultureInfo("en-US")));
}
}
class Job
{
protected double hours;
protected double price;
public const double RATE = 45.00;
public Job(int num, string cust, string desc, double hrs)
{
JobNumber = num;
Customer = cust;
Description = desc;
Hours = hrs;
}
public int JobNumber {get; set;}
public string Customer {get; set;}
public string Description {get; set;}
public double Hours
{
get
{
return hours;
}
set
{
hours = value;
price = hours * RATE;
}
}
public double Price
{
get
{
return price;
}
}
public override string ToString()
{
return(GetType() + " " + JobNumber + " " + Customer + " " +
Description + " " + Hours + " hours @" + RATE.ToString("C") +
" per hour. Total price is " + Price.ToString("C", CultureInfo.GetCultureInfo("en-US")));
}
public override bool Equals(Object e)
{
bool equal;
Job temp = (Job)e;
if(JobNumber == temp.JobNumber)
equal = true;
else
equal = false;
return equal;
}
public override int GetHashCode()
{
return JobNumber;
}
}
class RushJob : Job
{
public const double PREMIUM = 150.00;
public RushJob() :base(0, "", "", 0)
{
}
public override string ToString()
{
return(GetType() + " " + JobNumber + " " + Customer + " " +
Description + " " + Hours + " hours @" + RATE.ToString("C") +
" per hour. Rush job adds " + PREMIUM +
" premium. Total price is " + Price.ToString("C", CultureInfo.GetCultureInfo("en-US")));
}
public new double Hours
{
get
{
return hours;
}
set
{
hours = value;
price = hours * RATE + PREMIUM;
}
}
}
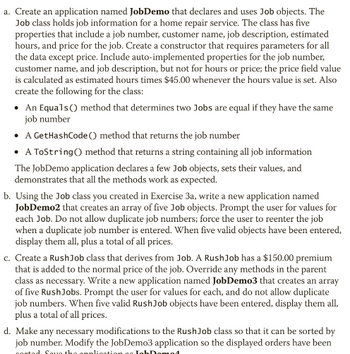
Transcribed Image Text:a. Create an application named JobDemo that declares and uses Job objects. The
Job class holds job information for a home repair service. The class has five
properties that include a job number, customer name, job description, estimated
hours, and price for the job. Create a constructor that requires parameters for all
the data except price. Include auto-implemented properties for the job number,
customer name, and job description, but not for hours or price; the price field value
is calculated as estimated hours times $45.00 whenever the hours value is set. Also
create the following for the class:
• An Equals() method that determines two Jobs are equal if they have the same
job number
• A GetHashCode () method that returns the job number
• A ToString() method that returns a string containing all job information
The JobDemo application declares a few Job objects, sets their values, and
demonstrates that all the methods work as expected.
b. Using the Job class you created in Exercise 3a, write a new application named
JobDemo2 that creates an array of five Job objects. Prompt the user for values for
each Job. Do not allow duplicate job numbers; force the user to reenter the job
when a duplicate job number is entered. When five valid objects have been entered,
display them all, plus a total of all prices.
c. Create a RushJob class that derives from Job. A RushJob has a $150.00 premium
that is added to the normal price of the job. Override any methods in the parent
class as necessary. Write a new application named JobDemo3 that creates an array
of five RushJobs. Prompt the user for values for each, and do not allow duplicate
job numbers. When five valid Rush Job objects have been entered, display them all,
plus a total of all prices.
d. Make any necessary modifications to the RushJob class so that it can be sorted by
job number. Modify the JobDemo3 application so the displayed orders have been
corted Saue the
lication
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 11 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C++ complete and create magical square #include <iostream> using namespace std; class Vec {public:Vec(){ }int size(){return this->sz;} int capacity(){return this->cap;} void reserve( int n ){// TODO:// (0) check the n should be > size, otherwise// ignore this action.if ( n > sz ){// (1) create a new int array which size is n// and get its addressint *newarr = new int[n];// (2) use for loop to copy the old array to the// new array // (3) update the variable to the new address // (4) delete old arraydelete[] oldarr; } } void push_back( int v ){// TODO: if ( sz == cap ){cap *= 2; reserve(cap);} // complete others } int at( int idx ){ } private:int *arr;int sz = 0;int cap = 0; }; int main(){Vec v;v.reserve(10);v.push_back(3);v.push_back(2);cout << v.size() << endl; // 2cout << v.capacity() << endl; // 10v.push_back(3);v.push_back(2);v.push_back(3);v.push_back(4);v.push_back(3);v.push_back(7);v.push_back(3);v.push_back(8);v.push_back(2);cout…arrow_forwardusing System; class Program { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { Console.WriteLine(number); number = number + 2; } int[] randNo = newint[88]; Random r = new Random(); int i=0; while (number <= 88) { randNo[i] = number; number+=1; i+=1; } for (i = 0; i < 3; i++) { Console.WriteLine("Random Numbers between 1 and 88 are " + randNo[r.Next(1, 88)]); } } } this code counts from 1-88 in odds and then selects three different random numbers. it keeps choosing 0 as a random number everytime. how can that be fixed?arrow_forwardProgram: File GamePurse.h: class GamePurse { // data int purseAmount; public: // public functions GamePurse(int); void Win(int); void Loose(int); int GetAmount(); }; File GamePurse.cpp: #include "GamePurse.h" // constructor initilaizes the purseAmount variable GamePurse::GamePurse(int amount){ purseAmount = amount; } // function definations // add a winning amount to the purseAmount void GamePurse:: Win(int amount){ purseAmount+= amount; } // deduct an amount from the purseAmount. void GamePurse:: Loose(int amount){ purseAmount-= amount; } // return the value of purseAmount. int GamePurse::GetAmount(){ return purseAmount; } File main.cpp: // include necessary header files #include <stdlib.h> #include "GamePurse.h" #include<iostream> #include<time.h> using namespace std; int main(){ // create the object of GamePurse class GamePurse dice(100); int amt=1; // seed the random generator srand(time(0)); // to play the…arrow_forward
- interface StudentsADT{void admissions();void discharge();void transfers(); }public class Course{String cname;int cno;int credits;public Course(){System.out.println("\nDEFAULT constructor called");}public Course(String c){System.out.println("\noverloaded constructor called");cname=c;}public Course(Course ch){System.out.println("\nCopy constructor called");cname=ch;}void setCourseName(String ch){cname=ch;System.out.println("\n"+cname);}void setSelectionNumber(int cno1){cno=cno1;System.out.println("\n"+cno);}void setNumberOfCredits(int cdit){credits=cdit;System.out.println("\n"+credits);}void setLink(){System.out.println("\nset link");}String getCourseName(){System.out.println("\n"+cname);}int getSelectionNumber(){System.out.println("\n"+cno);}int getNumberOfCredits(){System.out.println("\n"+credits); }void getLink(){System.out.println("\ninside get link");}} public class Students{String sname;int cno;int credits;int maxno;public Students(){System.out.println("\nDEFAULT constructor…arrow_forwardvoid fun(int i) { do { if (i % 2 != 0) cout =1); cout << endl; } int main() { int i = 1; while (i <= 8) { fun(i); it; } cout <arrow_forwardint main(){ long long int total; long long int init; scanf("%lld %lld", &total, &init); getchar(); long long int max = init; long long int min = init; int i; for (i = 0; i < total; i++) { char op1 = '0'; char op2 = '0'; long long int num1 = 0; long long int num2 = 0; scanf("%c %lld %c %lld", &op1, &num1, &op2, &num2); getchar(); long long int maxr = max; long long int minr = min; if (op1 == '+') { long long int sum = max + num1; maxr = sum; minr = sum; long long int res = min + num1; if (res > maxr) { max = res; } if (res < minr) { minr = res; } } else { long long int sum = max * num1; maxr = sum; minr = sum; long long int res = min * num1;…arrow_forwardclass implementation file -- Rectangle.cpp class Rectangle { #include #include "Rectangle.h" using namespace std; private: double width; double length; public: void setWidth (double); void setLength (double) ; double getWidth() const; double getLength() const; double getArea () const; } ; // set the width of the rectangle void Rectangle::setWidth (double w) { width = w; } // set the length of the rectangle void Rectangle::setLength (double l) { length l; //get the width of the rectangle double Rectangle::getWidth() const { return width; // more member functions herearrow_forwardusing System;class TicTacToe{ static void Print(char[] board) { Console.WriteLine(); Console.WriteLine($" {board[0]} | {board[1]} | {board[2]} "); Console.WriteLine($" {board[3]} | {board[4]} | {board[5]} "); Console.WriteLine($" {board[6]} | {board[7]} | {board[8]} "); Console.WriteLine(); } static void Main(string[] args) { char[] board = new char[9]; for (int i = 0; i < 9; i = i + 1) { board[i] = ' '; } Print(board); board[4] = 'X'; Print(board); board[0] = 'O'; Print(board); board[3] = 'X'; Print(board); board[5] = 'O'; Print(board); board[6] = 'X'; Print(board); board[2] = 'O'; Print(board); }} Hello! This program is in C# could a line of code be added to ask the user if they want to be "X" or if they want to be "O"?arrow_forwardTrace through the following program and show the output. Show your work for partial credit. public class Employee { private static int empID = 1111l; private String name , position; double salary; public Employee(String name) { empID ++; this.name 3 пате; } public Employee(Employee obj) { empID = obj.empĪD; пате %3D оbj.naте; position = obj.position; %3D public void empPosition(String empPosition) {position = empPosition;} public void empSalary(double empSalary) { salary = empSalary;} public String toString() { return name + " "+empID + " "+ position +" $"+salary; public void setName(String empName){ name = empName;} public static void main(String args[]) { Employee empOne = new Employee("James Smith"), empTwo; %3D empOne.empPosition("Senior Software Engineer"); етрOпе.етpSalary(1000); System.out.println(empOne); еmpTwo empTwo.empPosition("CEO"); System.out.println(empOne); System.out.println(empTwo); %3D етpОпе empOne ;arrow_forwardarrow_back_iosarrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
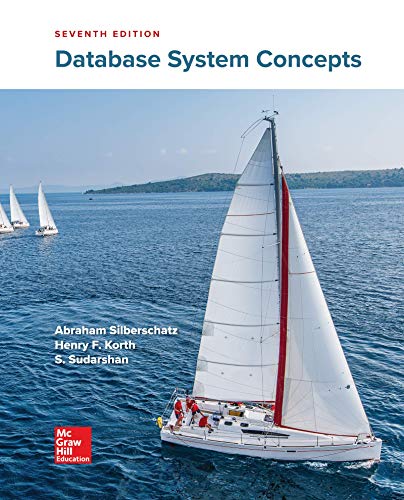
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
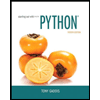
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
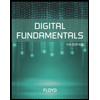
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
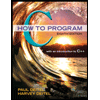
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
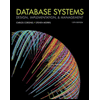
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
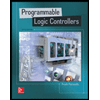
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education