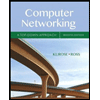
Macro parameters can be converted to strings inside the macro, a process called stringizing. Use that to modify the ASSERT macro so that it prints the assertion which failed.
#ifndef MYASSERT_H
#define MYASSERT_H
#include <iostream>
#define ASSERT(CONDITION, MSG) \
if (!(CONDITION)) std::cerr << "ASSERT failed: "<< MSG << std::endl; \
else std::cerr << "ASSERT passed" << std::endl;
#endif
Tester.cpp
#include <iostream> #include <iomanip> #include <string> #include <cmath> using namespace std; #include "myassert.h" int main() { double n = 1 / 4; ASSERT(n == .25, "Invalid division"); cout << "Expected: ASSERT failed: n == .25: Invalid Division" << endl; double x = 12 % 5; ASSERT(x == 2.0, "Invalid remainder"); cout << "Expected: ASSERT passed: x == 2.0" << endl; }

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- c code neededarrow_forwardProject 8 Specifications Using OOP, write a C++ program that will read in a file of names and a file of birth years. The file of names is called Names.txt and the file of birth years is called BirthYear.txt. Both input files should be located in the current directory of your program. The list of names in the Names.txt file corresponds to the list of birth years in the BirthYears.txt file. This means:The first name in the names.txt file corresponds to the first birth year in the birthyear.txt file. The second name in the names.txt file corresponds to the second birth year in the birthyear.txt file...and so on.Read in and store the names into an array of 30. Next read in and store the birth years into another array of 30 integers.Sort the arrays using the selection sort or the bubblesort code found in your textbook. List the roster of names in ascending alphabetical order displaying their birth years beside their names.Next, prompt the user to enter a birth year. Validate this…arrow_forwardfunction name- def my-polyarrow_forward
- FileAttributes.c 1. Create a new C source code file named FileAttributes.c preprocessor 2. Include the following C libraries a. stdio.h b. stdlib.h c. time.h d. string.h e. dirent.h f. sys/stat.h 3. Function prototype for function printAttributes() main() 4. Write the main function to do the following a. Return type int b. Empty parameter list c. Declare a variable of data type struct stat to store the attribute structure (i.e. statBuff) d. Declare a variable of data type int to store an error code (i.e. err) e. Declare a variable of data type struct dirent as a pointer (i.e. de) f. Declare a variable of data type DIR as a pointer set equal to function call opendir() passing explicit text “.” as an argument to indicate the current directory (i.e. dr) g. If the DIR variable is equal to NULL do the following i. Output to the…arrow_forwardTemplate functions may also have reference parameters. Write the function template consume which reads a value from standard input, and returns the value. Complete the following file: consumer.h 1 #ifndef CONSUMER_H 2 #define CONSUMER_H 3 4 #include 5 6 // Add your function template here 7 8 Submit } #endif Use the following file: Demo.cpp #include #include #include #include using namespace std; #include "consumer.h" int main() { int a; cout << consume (a) * 2 << endl; double b; cout << sqrt(consume (b)) << endl; string s; cout << consume(s).size() << endl;arrow_forwardpseudo code for the code //C++ Code #include<iostream>#include<fstream>using namespace std;/*Create a global variable of type ofstream for the output file*/ofstream outfile; /** This function asks the user for the number of employees in the company. This value should be returned as an int. The function accepts no arguments (No parameter/input).*/int NumOfEmployees();/** accepts an argument of type int for the number of employees in the company and returns the total of missed days as an int. This function should do the following:Asks the user to enter the following information for each employee:The employee number (ID) (Assume the employee number is 4 digits or fewer, but don't validate it).The number of days that employee missed during the past year.Writes each employee number (ID) and the number of days missed to the output file */int TotDaysAbsent(int numberOfEmployees);/* calculates the average number of days absent.The function takes two arguments:the number of…arrow_forward
- pseudo code for the code //C++ Code #include<iostream>#include<fstream>using namespace std;/*Create a global variable of type ofstream for the output file*/ofstream outfile; /** This function asks the user for the number of employees in the company. This value should be returned as an int. The function accepts no arguments (No parameter/input).*/int NumOfEmployees();/** accepts an argument of type int for the number of employees in the company and returns the total of missed days as an int. This function should do the following:Asks the user to enter the following information for each employee:The employee number (ID) (Assume the employee number is 4 digits or fewer, but don't validate it).The number of days that employee missed during the past year.Writes each employee number (ID) and the number of days missed to the output file */int TotDaysAbsent(int numberOfEmployees);/* calculates the average number of days absent.The function takes two arguments:the number of…arrow_forwardPlease answer in C++arrow_forwardEXCEL VBA Write a user-defined function called NoSpace which takes a string as input and outputs the same string with all of the spaces removed. For example, given the string The quick brown fox it should return the string Thequickbrownfox Use a a suitable loop, declare all variable types, and include appropriate comments in your code. Code to be written in EXCEL VBA Code to be written for a user-defined function and not a macroarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
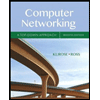
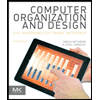
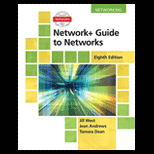
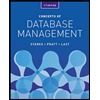
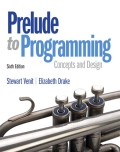
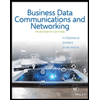