loyees. To display payment type/salary This must calculate pay for either an hourly paid worker or a salaried worker. Hourly paid workers are paid their hourly pay rate times the number of hours worked. Salaried workers are paid their regular salary plus any bonus they may have earned. The program should declare two structures for the following data: - Hourly Paid: - - HoursWorked - - HourlyRate - Salaried: - - Salary - - Bonus The program should ask the user whether he or she is calculating the pay for an hourly paid worker or a salaried worker. Regardless of which the user selects
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
(In C++) Part 1: Write a program that tracks employee information. The program should use classes for employee records. Each employee should have a record containing the following information: - First Name - Last Name - Phone Number - Address - City - State Zip - Position - Job title - Payment type - The program should be able to allow for the following: - the input of new employees, - display of existing employees, - editing existing employees, - deleting employees. To display payment type/salary This must calculate pay for either an hourly paid worker or a salaried worker. Hourly paid workers are paid their hourly pay rate times the number of hours worked. Salaried workers are paid their regular salary plus any bonus they may have earned. The program should declare two structures for the following data: - Hourly Paid: - - HoursWorked - - HourlyRate - Salaried: - - Salary - - Bonus The program should ask the user whether he or she is calculating the pay for an hourly paid worker or a salaried worker. Regardless of which the user selects, the appropriate members of the union will be used to store the data that will be used to calculate the pay. Input Validation: Do not accept negative numbers. Do not accept values greater than 80 for HoursWorked.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

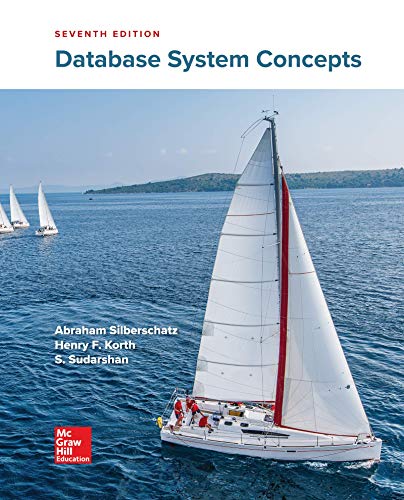
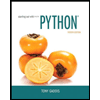
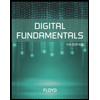
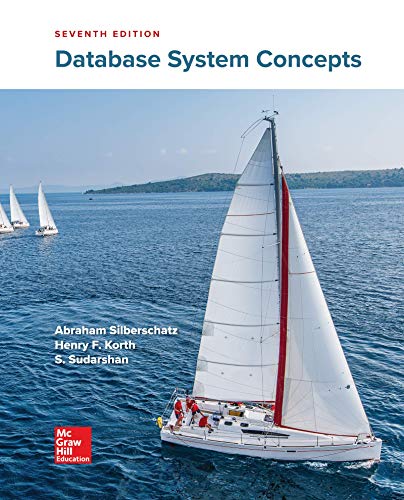
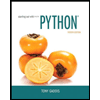
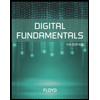
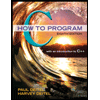
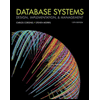
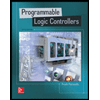