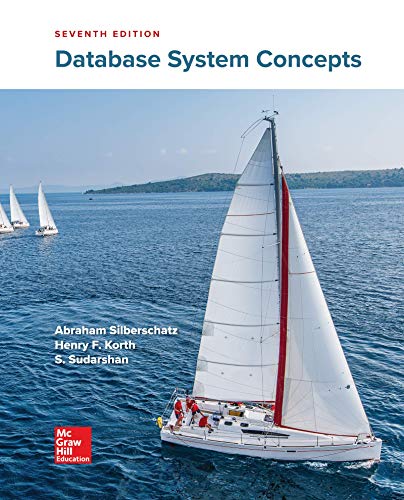
Concept explainers
I need to convert this code using C++, that currently works with structs into classes, with the main class being called myGraph and a node class (if necessary) using composition.
#include <stdio.h>
#include <stdlib.h>
#include <iostream>
using namespace std;
struct AdjListNode //node class
{
int dest;
struct AdjListNode* next;
};
struct AdjList //node class
{
struct AdjListNode *head;
};
struct Graph //myGraph class
{
int V;
struct AdjList* array;
};
struct AdjListNode * newAdjListNode(int dest)
{
struct AdjListNode * newNode = new AdjListNode;
newNode->dest = dest;
newNode->next = NULL;
return newNode;
}
struct Graph* createGraph(int V) //constructor
{
struct Graph* graph = new Graph;
graph->V = V;
graph->array = new AdjList;
int i;
for (i = 0; i < V; ++i)
graph->array[i].head = NULL;
return graph;
}
void addEdge(struct Graph* graph, int src, int dest)
{
struct AdjListNode* newNode = newAdjListNode(dest);
newNode->next = graph->array[src].head;
graph->array[src].head = newNode;
newNode = newAdjListNode(src);
newNode->next = graph->array[dest].head;
graph->array[dest].head = newNode;
}
void printGraph(struct Graph* graph)
{
int v;
for (v = 0; v < graph->V; ++v)
{
struct AdjListNode* pCrawl = graph->array[v].head;
cout << endl << "Adjacency list of vertex " << v << endl << " head";
while (pCrawl)
{
cout << "-> " << pCrawl->dest;
pCrawl = pCrawl->next;
}
cout << endl;
}
}
int main()
{
int V = 5;
struct Graph* graph = createGraph(V);
addEdge(graph, 0, 1);
addEdge(graph, 0, 4);
addEdge(graph, 1, 2);
addEdge(graph, 1, 3);
addEdge(graph, 1, 4);
addEdge(graph, 2, 3);
addEdge(graph, 3, 4);
printGraph(graph);
system("pause");
return 0;
}

Step by stepSolved in 4 steps with 2 images

- C++ pleasearrow_forwardThe C++ Vertex class represents a vertex in a graph. The class's only data member is _____ Ⓒlabel 1000 O weight O framEdges O toEdges Question 36 The C++ Graph class's fromEdges member is a(n) _________ O string Ⓒdouble O vector O unordered_map Question 37 The C++ Graph class's AddVertex() function's return type is _____ void O Edge+ Overtex) O vectorarrow_forwardRe-write Sample Program 11.2 so that it works for an array of structures. Write the program so that it compares 6 circles. You will need to come up with a new way of determining which circle’s center is closest to the origin. Sample code 11.2 #include <iostream>#include <cmath> // necessary for pow function#include <iomanip>using namespace std;struct circle // declares the structure circle{ // This structure has 6 membersfloat centerX; // x coordinate of centerfloat centerY; // y coordinate of centerfloat radius;float area;float circumference;float distance_from_origin;};const float PI = 3.14159;int main(){circle circ1, circ2; // defines 2 circle structure variablescout << "Please enter the radius of the first circle: ";cin >> circ1.radius;cout << endl<< "Please enter the x-coordinate of the center: ";cin >> circ1.centerX;cout << endl<< "Please enter the y-coordinate of the center: ";cin >>…arrow_forward
- Computer science JAVA programming question i need help with this entire question pleasearrow_forwardC programming fill in the following code #include "graph.h" #include <stdio.h>#include <stdlib.h> /* initialise an empty graph *//* return pointer to initialised graph */Graph *init_graph(void){} /* release memory for graph */void free_graph(Graph *graph){} /* initialise a vertex *//* return pointer to initialised vertex */Vertex *init_vertex(int id){} /* release memory for initialised vertex */void free_vertex(Vertex *vertex){} /* initialise an edge. *//* return pointer to initialised edge. */Edge *init_edge(void){} /* release memory for initialised edge. */void free_edge(Edge *edge){} /* remove all edges from vertex with id from to vertex with id to from graph. */void remove_edge(Graph *graph, int from, int to){} /* remove all edges from vertex with specified id. */void remove_edges(Graph *graph, int id){} /* output all vertices and edges in graph. *//* each vertex in the graphs should be printed on a new line *//* each vertex should be printed in the following format:…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
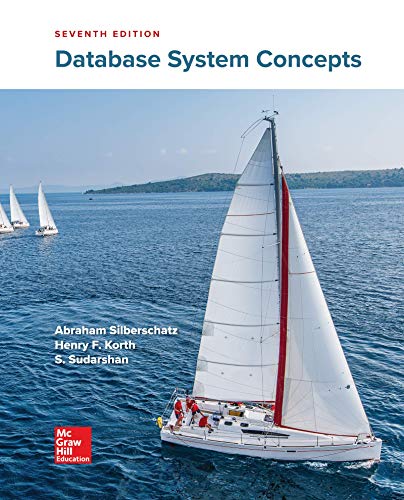
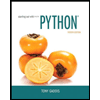
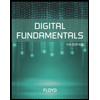
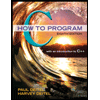
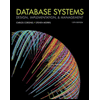
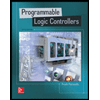