Java you should have one homework.java file For this problem set, you will submit a single java file named Homework.java. You are supposed to add the functions described below to your Homework class. The functional
Java you should have one homework.java file
For this problem set, you will submit a single java file named Homework.java. You are supposed to add the functions described below to your Homework class. The functional signatures of the functions you create must exactly match the signatures in the problem titles. You are not allowed to use any 3rd party libraries in the homework assignment nor are you allowed to use any dependencies from the java.util package besides the Pattern Class. When you hava completed your homework, upload the Homework.java file to Grader Than. All tests will timeout and fail if your code takes longer than 10 seconds to complete.
privateFunction()
This is a private non-static function that takes no arguments and returns an integer.
You don't need to put any code in this function, you may leave the function's code empty. Don't overthink this problem. This is to test your knowledge on how to create a non-static private function.
Arguments:
returns int - You may choose any integer you would like.
protectedFunction(String, int)
This is a protected non-static function that takes a String and an int as its arguments and returns nothing.
You don't need to put any code in this function, you may leave the function's code empty. Don't overthink this problem. This is to test your knowledge on how to create a non-static protected function.
Arguments:
String text - An string
String int - An integer
mergeAndRemove(int[], int[])
This is a public static function that takes a int[] and int[] for the parameters and returns an int[].
Given two arrays of integers. Your job is to combine them into a single array and remove any duplicates, finally return the merged array.
Arguments:
int[] array_1 - An array of integers
int[] array_2 - An array of integers
returns int[] - An array of integers containing the unique values from both array_1 and array_2
Example:
array_1 = [1,2,3] array_2 = [2,3,4] output = [1,2,3,4]

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

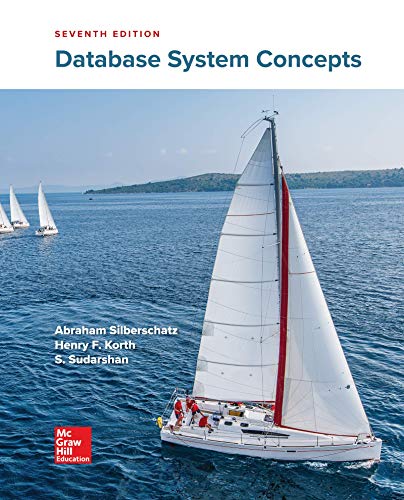
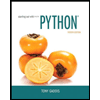
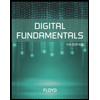
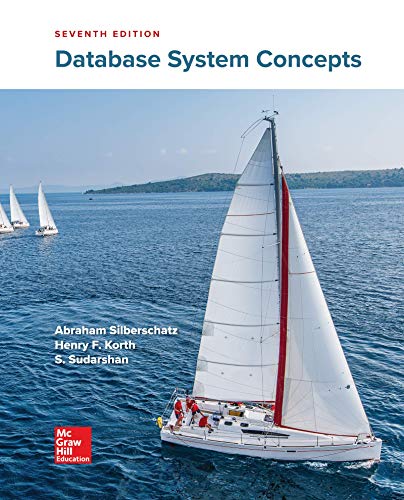
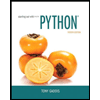
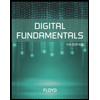
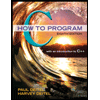
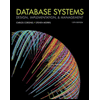
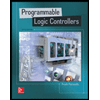