Java Help Please This is real please stop wasting time. Real Answer Please This questioned was answered in a wrong way several times someone else to answer it please Write a new class called DistanceFitnessTracker that extends FitnessTracker. DistanceFitnessTracker will represent a fitness tracker able to record only distance. Here, you will not be asked to convert between distance units(Kilometers is the unit) Distance.java public class Distance { // Enum of distance units public enum DistanceUnit { KILOMETRES, MILES } // Constants private static final double KMS_PER_MILE = 1.609; private static final double MILES_PER_KM = 0.621; // Instance variables private double value; private DistanceUnit distanceUnit; // Constructors public Distance(double value) { this.value = value; // Default unit is km this.distanceUnit = DistanceUnit.KILOMETRES; } public Distance(double value, DistanceUnit distanceUnit) { this.value = value; this.distanceUnit = distanceUnit; } // Methods: You should remove the comments and complete the method bodies for all the methods below // Think that all these methods are in the class for a reason, so you should use them all // DO NOT MODIFY the signature of these methods! public double getValue() { return value; } public double getValue(DistanceUnit unit) { if(unit==this.distanceUnit) return value; else{ if(unit==DistanceUnit.MILES) return convertToMiles(value); else if(unit==DistanceUnit.KILOMETRES) return convertToKilometers(value); } return 0; } public void setValue(double value) { this.value = value; } public DistanceUnit getDistanceUnit() { return distanceUnit; } public void changeDistanceUnit(DistanceUnit distanceUnit) { this.distanceUnit = distanceUnit; } public static double convertToMiles(double kilometers){ return kilometers*MILES_PER_KM; } public static double convertToKilometers(double miles){ return miles*KMS_PER_MILE; } public String toString() { return "Distance [value=" + value + ", distanceUnit=" + distanceUnit + "]"; } } FitnessTracker.java public class FitnessTracker { // String containing model name of fitness activity tracker private String modelName; public FitnessTracker(String modelName) { this.modelName = modelName; } public String getModelName() { return modelName; } @Override public boolean equals(Object obj) { // TODO Implement a method to check equality if(this == obj) // it will check if both the references are refers to same object return true; if(obj == null || obj.getClass()!= this.getClass()) //it check the argument of the type FitnessTracker by comparing the classes of the passed argument and this object. return false; FitnessTracker FT=(FitnessTracker)obj; // type casting of the argument. return (FT.modelName == this.modelName);// comparing the state of argument with the state of 'this' Object. } } StepFitnessTracker.java public class StepsFitnessTracker extends FitnessTracker { // Stores total number of steps private Steps totalSteps; public StepsFitnessTracker(String modelName, Steps steps) { super(modelName); this.totalSteps = steps; } // Add steps to the total public void addSteps(Steps stepsToAdd) { int numSteps = this.totalSteps.getValue() + stepsToAdd.getValue(); this.totalSteps.setValue(numSteps); } // Getter for total number of steps public Steps getTotalSteps() { return totalSteps; } public String toString() { return "Steps Tracker " + getModelName() + "; Total Steps: " + getTotalSteps().getValue(); } @Override public boolean equals(Object obj) { // TODO Implement a method to check equality if(this == obj) // it will check if both the references are refers to same object return true; if(obj == null || obj.getClass()!= this.getClass()) //it check the argument of the type StepsFitnessTracker by comparing the classes of the passed argument and this object. return false; StepsFitnessTracker SFFT=(StepsFitnessTracker)obj; // type casting of the argument. if(SFFT.getTotalSteps() == this.totalSteps && this.totalSteps == null) return true; // check whether they both are null // check if one is null and other is not. if(SFFT.getTotalSteps() == null && this.totalSteps != null) return false; if(SFFT.getTotalSteps() != null && this.totalSteps == null) return false; // at the end check whether value of both are same. return (SFFT.getTotalSteps().getValue() == this.totalSteps.getValue()); } } TestDistanceFitnessTracker,java public class TestDistanceFitnessTracker { @Test public void testTrackersDifferentSumNotEqual() { DistanceFitnessTracker a = new DistanceFitnessTracker("hr", new Distance(20)); DistanceFitnessTracker b = new DistanceFitnessTracker("hr", new Distance(20)); b.addDistance(new Distance(10)); assertNotEquals(a, b); assertNotEquals(b, a); } }
Java Help Please This is real please stop wasting time. Real Answer Please This questioned was answered in a wrong way several times someone else to answer it please
Write a new class called DistanceFitnessTracker that extends FitnessTracker. DistanceFitnessTracker will represent a fitness tracker able to
record only distance. Here, you will not be asked to convert between distance units(Kilometers is the unit)
Distance.java
public class Distance {
// Enum of distance units
public enum DistanceUnit {
KILOMETRES,
MILES
}
// Constants
private static final double KMS_PER_MILE = 1.609;
private static final double MILES_PER_KM = 0.621;
// Instance variables
private double value;
private DistanceUnit distanceUnit;
// Constructors
public Distance(double value) {
this.value = value;
// Default unit is km
this.distanceUnit = DistanceUnit.KILOMETRES;
}
public Distance(double value, DistanceUnit distanceUnit) {
this.value = value;
this.distanceUnit = distanceUnit;
}
// Methods: You should remove the comments and complete the method bodies for all the methods below
// Think that all these methods are in the class for a reason, so you should use them all
// DO NOT MODIFY the signature of these methods!
public double getValue() {
return value;
}
public double getValue(DistanceUnit unit) {
if(unit==this.distanceUnit)
return value;
else{
if(unit==DistanceUnit.MILES)
return convertToMiles(value);
else if(unit==DistanceUnit.KILOMETRES)
return convertToKilometers(value);
} return 0;
}
public void setValue(double value) {
this.value = value;
}
public DistanceUnit getDistanceUnit() {
return distanceUnit;
}
public void changeDistanceUnit(DistanceUnit distanceUnit) {
this.distanceUnit = distanceUnit;
}
public static double convertToMiles(double kilometers){
return kilometers*MILES_PER_KM;
}
public static double convertToKilometers(double miles){
return miles*KMS_PER_MILE;
}
public String toString() {
return "Distance [value=" + value + ", distanceUnit=" + distanceUnit + "]";
}
}
FitnessTracker.java
public class FitnessTracker {
// String containing model name of fitness activity tracker
private String modelName;
public FitnessTracker(String modelName) {
this.modelName = modelName;
}
public String getModelName() {
return modelName;
}
@Override
public boolean equals(Object obj) {
// TODO Implement a method to check equality
if(this == obj) // it will check if both the references are refers to same object
return true;
if(obj == null || obj.getClass()!= this.getClass()) //it check the argument of the type FitnessTracker by comparing the classes of the passed argument and this object.
return false;
FitnessTracker FT=(FitnessTracker)obj; // type casting of the argument.
return (FT.modelName == this.modelName);// comparing the state of argument with the state of 'this' Object.
}
}
StepFitnessTracker.java
public class StepsFitnessTracker extends FitnessTracker {
// Stores total number of steps
private Steps totalSteps;
public StepsFitnessTracker(String modelName, Steps steps) {
super(modelName);
this.totalSteps = steps;
}
// Add steps to the total
public void addSteps(Steps stepsToAdd) {
int numSteps = this.totalSteps.getValue() + stepsToAdd.getValue();
this.totalSteps.setValue(numSteps);
}
// Getter for total number of steps
public Steps getTotalSteps() {
return totalSteps;
}
public String toString() {
return "Steps Tracker " + getModelName() +
"; Total Steps: " + getTotalSteps().getValue();
}
@Override
public boolean equals(Object obj) {
// TODO Implement a method to check equality
if(this == obj) // it will check if both the references are refers to same object
return true;
if(obj == null || obj.getClass()!= this.getClass()) //it check the argument of the type StepsFitnessTracker by comparing the classes of the passed argument and this object.
return false;
StepsFitnessTracker SFFT=(StepsFitnessTracker)obj; // type casting of the argument.
if(SFFT.getTotalSteps() == this.totalSteps && this.totalSteps == null) return true; // check whether they both are null
// check if one is null and other is not.
if(SFFT.getTotalSteps() == null && this.totalSteps != null) return false;
if(SFFT.getTotalSteps() != null && this.totalSteps == null) return false;
// at the end check whether value of both are same.
return (SFFT.getTotalSteps().getValue() == this.totalSteps.getValue());
}
}
TestDistanceFitnessTracker,java
public class TestDistanceFitnessTracker {
@Test
public void testTrackersDifferentSumNotEqual() {
DistanceFitnessTracker a = new DistanceFitnessTracker("hr", new Distance(20));
DistanceFitnessTracker b = new DistanceFitnessTracker("hr", new Distance(20));
b.addDistance(new Distance(10));
assertNotEquals(a, b);
assertNotEquals(b, a);
}
}
To make it simple we need to create a new java class called distancefitnesstracker that gets information from distance.java and extedns fitnesstracker and is similar to stepfitnesstracker and heartratefitnesstraker( in pictures) the distancefitnesstracker needs to pass the testdistancefitnesstracker code in the question
Also picture that testdistancetracker passed all tests


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

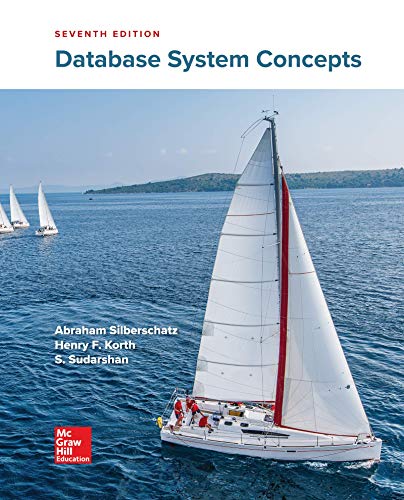
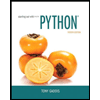
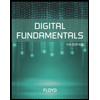
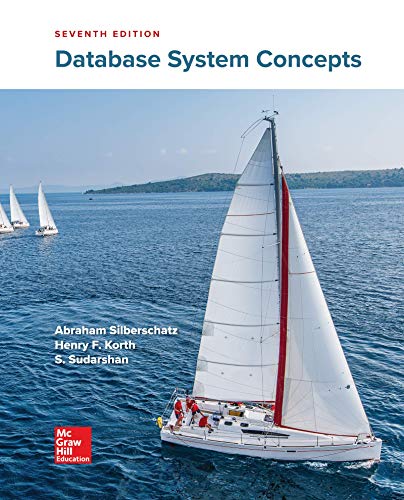
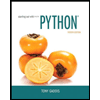
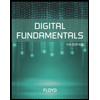
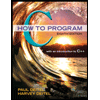
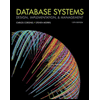
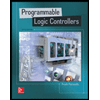