JAVA PLEASE : Assume the Tree consists of your ID digits, inserted in the fashion to minimize the height of the tree. Remove duplicates if needed. Trace the delete() method above in a similar fashion as the insert was traced, delete 3 last digits of your ID from the tree in the same order as they are present in you ID.
JAVA PLEASE : Assume the Tree consists of your ID digits, inserted in the fashion to minimize the height of the tree. Remove duplicates if needed. Trace the delete() method above in a similar fashion as the insert was traced, delete 3 last digits of your ID from the tree in the same order as they are present in you ID.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
JAVA PLEASE :
Assume the Tree consists of your ID digits, inserted in the fashion to minimize the height of the tree. Remove duplicates if needed. Trace the delete() method above in a similar fashion as the insert was traced, delete 3 last digits of your ID from the tree in the same order as they are present in you ID.

Transcribed Image Text:The image provides pseudocode for insert and delete methods in a binary search tree. Below is a detailed transcription:
---
### Insert Method
**boolean insert(E e)**
1. **Check if the root is null:**
- If true, create a new root node.
- `root = createNewNode(e)` // Create root
2. **Locate the parent node:**
- Initialize `TreeNode<E> parent = null` and `TreeNode<E> current = root`
- Use a while loop (`while (current != null)`) to find the correct position:
- If `c.compare(e, current.element) < 0`:
- `parent = current`
- `current = current.left`
- Else if `c.compare(e, current.element) > 0`:
- `parent = current`
- `current = current.right`
- Else
- Return false // No duplicates allowed. Skip it.
**Create the new node and attach it:**
- If `c.compare(e, parent.element) < 0`:
- `parent.left = createNewNode(e)`
- Else:
- `parent.right = createNewNode(e)`
Increment size and return true // Element inserted
---
### Delete Method
**public boolean delete(E e)**
1. **Find the node to delete and its parent:**
- Initialize `TreeNode<E> parent = null` and `TreeNode<E> current = root`
- Use a while loop (`while (current != null)`) to locate the node:
- If `c.compare(e, current.element) < 0`:
- `parent = current`
- `current = current.left`
- Else if `c.compare(e, current.element) > 0`:
- `parent = current`
- `current = current.right`
- Else
- Break // Current points to the node to delete
- If `current == null`, return false // Element not found
2. **Deletion cases:**
- **Case 1:** Current has no left child
- If the parent is null, set `root = current.right`
- Else, update parent's pointers:
- If `c.compare(e, parent.element) < 0`:
- `parent.left = current.right`
- Else:
- `parent.right = current

Transcribed Image Text:# Insertion and Deletion in a Binary Search Tree
## Inserting 1, 2, 3, 4 in Order
### Step 1: Insert 1
- **Condition**: The tree is empty.
- **Action**:
- Since `root == null` is true, create a new node with value 1. Set it as the root.
- **Result**: Tree size is 1 (1 node).
### Step 2: Insert 2
- **Condition**: Root is 1.
- **Action**:
- **Locate the Parent Node**
- Initialize `current` as root (1).
- Iterate: Since `current` is not null and `2 - 1 = 1 > 0`, the new node becomes the right child.
- **Create New Node**:
- Create a new node with value 2 and assign it as the right child of root 1.
### Step 3 & 4: (Omitted for Conciseness)
- Follow similar steps as above for inserting 3 and 4 in order.
### Deletion Process (Indicated by Code Comments)
- **Identify Rightmost Node**:
- Traverse to rightmost leaf node of the left subtree.
- **Replace Current**:
- Set `current.element` to `rightMost.element`.
- **Eliminate Rightmost Node**:
- Re-adjust pointers to remove the node.
- **Reduce Tree Size**:
- Decrement tree size after deletion.
This step-by-step process helps in maintaining the properties of a Binary Search Tree during insertion and deletion operations.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
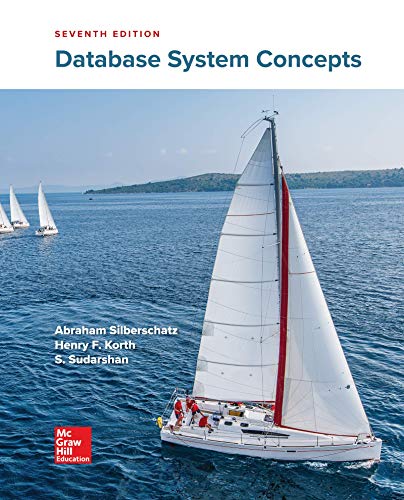
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
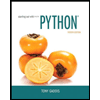
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
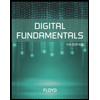
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
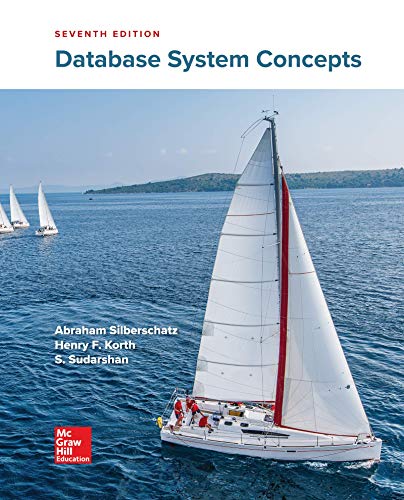
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
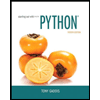
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
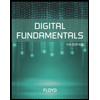
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
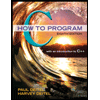
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
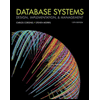
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
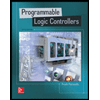
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education