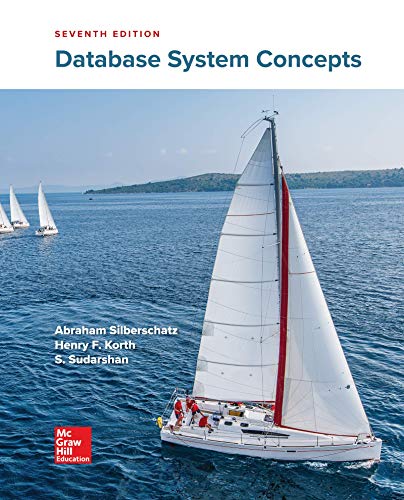
Java Code: How to implement logic for ParseIf, ParseFor, and ParseWhile where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these three methods.

It takes careful handling while parsing and processing programming language features in Java, such as if, for, and while statements, to make sure that the input is well-formed and to offer useful error messages in the event of syntax mistakes. This is crucial for creating a reliable programming language parser. In this context, we'll look at how to put logic for parsing if, for, and while statements into practice, along with error handling and helpful exception messages.
Please refer to the following steps for the complete solution to the problem above.
Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 5 images

Create more methods for ParseDoWhile, ParseDelete, and ParseReturn where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these three methods.
Create more methods for ParseDoWhile, ParseDelete, and ParseReturn where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these three methods.
- Do it in C++ template (STL)arrow_forwardJava Code: How to implement logic for ParseIf ParseFor, ParseWhile, ParseDoWhile, ParseDelete, ParseReturn, and ParseFunctionCall where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these methods.arrow_forwarddon't use others answers java 1. Write a generic static method that takes a Stack of any type element as a parameter, pops each element from the stack, and prints it. It should have a type parameter that represents the Stack’s element type.arrow_forward
- In Java In this assignment, you should provide a complete CircularQueueDriver class that fullytests the functionality of your CircularQueue class that you have improved in Lab 8. YourCircularQueueDriver class should:• Instantiate a 3-element CircularQueue.• Use a loop to add strings to the queue until the add method returns false (whichindicates a full queue).• Call showQueue.• Use a loop to remove strings from the queue until the remove method returns null(which indicates an empty queue). As you remove each string from the queue, print theremoved string.Sample Run:Monsieur AMonsieur BMonsieur Cremoved: Monsieur Aremoved: Monsieur Bremoved: Monsieur C CircularQueue Class: public class CircularQueue { private String[] queue; // array that implements a circular queue private int length = 0; // number of filled elements private int capacity; // size of array private int front = 0; // index of first item in queue private int rear = 0; // one past the index of the last item…arrow_forwardusing java code Problem: Suppose we want to write a program for the class BinaryTree that counts the number of times an object occurs in the tree. We need to use a method with the following header, public int count (O anObject) 1. Name your class objectCounter 2. Define main method, test the program 3. Write a method using one of the iterators of the binary tree. 4. Write another method using a private recursive method of the same name NB: Can you add main method to test the code please for 2. Define main method, test the programarrow_forwardInput : 1->2->3->2->1->NULL Output: It's a palindrome !!! Create a palindrome of your student ID and then push the element to the stack and queue. Pop each element from the stack and the queue and then check for the mismatch.arrow_forward
- //No need for the whole code //just the methods please for both Write a recursive method “int sumPos(Node head)” to calculate the sum of positive integers in a linked list of integers referenced by head. No global variables are allowed. Node is declared as: Node { int value; Node next; } Write a non-recursive method “int sumPos(Node head)” to calculate the sum of positive integers in a linked list of integers referenced by head. No global variables are allowed. Node is declared as: Node { int value; Node next; }arrow_forwardSuppose you are trapped on a desert island with nothing but a priority queue, and you need to implement a stack. Complete the following class that stores pairs (count, element) where the count is incremented with each insertion. Recall that make_pair(count, element) yields a pair object, and that p.second yields the second component of a pair p. The pair class defines an operator< that compares pairs by their first component, and uses the second component only to break ties.Code: #include <iostream>#include <queue>#include <string>#include <utility> using namespace std; class Stack{public: Stack(); string top(); void pop(); void push(string element);private: int count; priority_queue<pair<int, string>> pqueue;}; Stack::Stack(){ /* Your code goes here */} string Stack::top(){ /* Your code goes here */} void Stack::pop(){ /* Your code goes here */} void Stack::push(string element){ /* Your code goes here */} int main(){ Stack…arrow_forwardJava Code: How to implement logic for ParseDoWhile, ParseDelete, and ParseReturn where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these three methods.arrow_forward
- Java Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forwardPlease answer the question in the screenshot. The language used here is Java. Please use the starting code.arrow_forwardAssume class MyStack implements the following StackGen interface. For this question, make no assumptions about the implementation of MyStack except that the following interface methods are implemented and work as documented. Write a public instance method for MyStack, called interchange(T element) to replace the bottom "two" items in the stack with element. If there are fewer than two items on the stack, upon return the stack should contain exactly two items that are element.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
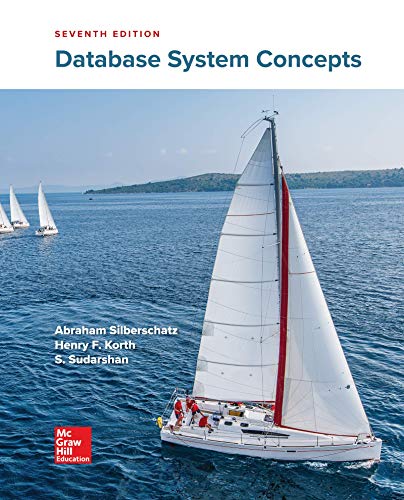
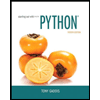
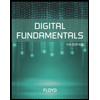
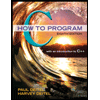
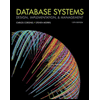
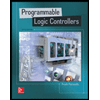