Java Code JDK 11 Please Turn my min-heap into a max -heap. Thank you! public MinHeap(ArrayList data) { // Initialize the backing array backingArray = (T[]) new Comparable[2 * data.size() + 1]; // Copy the data to the backing array int i = 1; for (T value : data) backingArray[i++] = value; size = data.size(); // Heapify the backing array for (int parentIndex = size / 2; parentIndex >= 1; parentIndex--) heapify(parentIndex); } /** * A recursive helper method that will heapify the backing array so that * it will become a min heap. * * @param parentIndex Parent index being processed */ private void heapify(int parentIndex) { // Stop if we end up in an empty slot if (backingArray[parentIndex] == null) return; int leftChildIndex = parentIndex * 2; int rightChildIndex = parentIndex * 2 + 1; // Find which is smaller between the parent and any of its 2 children int smallerIndex = parentIndex; if (leftChildIndex <= size && backingArray[leftChildIndex].compareTo(backingArray[smallerIndex]) < 0) smallerIndex = leftChildIndex; if (rightChildIndex <= size && backingArray[rightChildIndex].compareTo(backingArray[smallerIndex]) < 0) smallerIndex = rightChildIndex; // Swap the smaller value to the parent if there is smaller than the parent if (smallerIndex != parentIndex) { T value = backingArray[parentIndex]; backingArray[parentIndex] = backingArray[smallerIndex]; backingArray[smallerIndex] = value; // Since there's a new parent, repeat the process on that parent heapify(smallerIndex); } }
Java Code JDK 11
Please Turn my min-heap into a max -heap. Thank you!
public MinHeap(ArrayList<T> data) {
// Initialize the backing array
backingArray = (T[]) new Comparable[2 * data.size() + 1];
// Copy the data to the backing array
int i = 1;
for (T value : data)
backingArray[i++] = value;
size = data.size();
// Heapify the backing array
for (int parentIndex = size / 2; parentIndex >= 1; parentIndex--)
heapify(parentIndex);
}
/**
* A recursive helper method that will heapify the backing array so that
* it will become a min heap.
*
* @param parentIndex Parent index being processed
*/
private void heapify(int parentIndex) {
// Stop if we end up in an empty slot
if (backingArray[parentIndex] == null)
return;
int leftChildIndex = parentIndex * 2;
int rightChildIndex = parentIndex * 2 + 1;
// Find which is smaller between the parent and any of its 2 children
int smallerIndex = parentIndex;
if (leftChildIndex <= size && backingArray[leftChildIndex].compareTo(backingArray[smallerIndex]) < 0)
smallerIndex = leftChildIndex;
if (rightChildIndex <= size && backingArray[rightChildIndex].compareTo(backingArray[smallerIndex]) < 0)
smallerIndex = rightChildIndex;
// Swap the smaller value to the parent if there is smaller than the parent
if (smallerIndex != parentIndex) {
T value = backingArray[parentIndex];
backingArray[parentIndex] = backingArray[smallerIndex];
backingArray[smallerIndex] = value;
// Since there's a new parent, repeat the process on that parent
heapify(smallerIndex);
}
}

Step by step
Solved in 2 steps

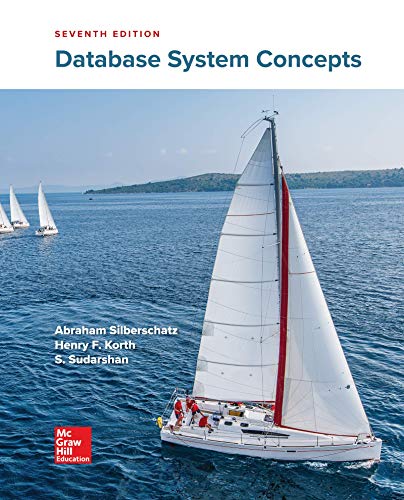
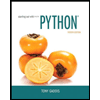
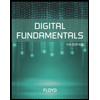
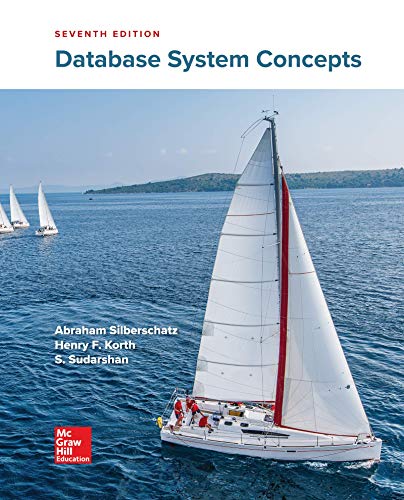
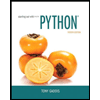
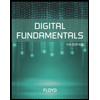
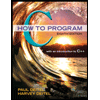
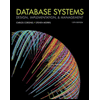
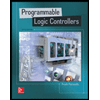