int [] list = {18,57,8,89,7} and the length = 5 Sort the array using insertion sort algorithm. Fill out the trace table public class InsertionSort { /** The method for sorting the numbers */ public static void insertionSort(int[] list) { for (int i = 1; i < list.length; i++) { /** insert list[i] into a sorted sublist list[0..i-1] so that list[0..i] is sorted. */ int currentElement = list[i]; int k; for (k = i - 1; k >= 0 && list[k] > currentElement; k--) { list[k + 1] = list[k]; } // Insert the current element into list[k+1] list[k + 1] = currentElement; } } /** A test method */ public static void main(String[] args) { int[] list = {18,57,8,89,7}; insertionSort(list); for (int i = 0; i < list.length; i++) System.out.print(list[i] + " "); } }
int [] list = {18,57,8,89,7} and the length = 5
Sort the array using insertion sort
public class InsertionSort {
/** The method for sorting the numbers */
public static void insertionSort(int[] list) {
for (int i = 1; i < list.length; i++) {
/** insert list[i] into a sorted sublist list[0..i-1] so that list[0..i] is sorted. */
int currentElement = list[i];
int k; for (k = i - 1; k >= 0 && list[k] > currentElement; k--) {
list[k + 1] = list[k];
}
// Insert the current element into list[k+1]
list[k + 1] = currentElement;
}
}
/** A test method */
public static void main(String[] args) {
int[] list = {18,57,8,89,7};
insertionSort(list);
for (int i = 0; i < list.length; i++)
System.out.print(list[i] + " "); } }
![i CE=list[i]
k
list[k]>CE
list[k+1]=list[k]
list[k+1]=CE](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2ec814c7-90f3-4435-9bb8-fcbab3f733ac%2Fab0fe789-8239-4a76-89c2-79608809c583%2Fgsdcxwm_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

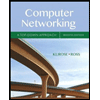
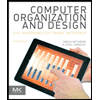
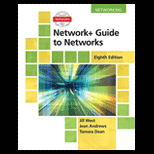
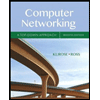
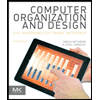
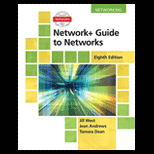
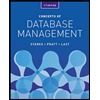
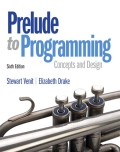
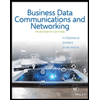