Is there a way to simataniously get both student names' and roll numbers' to be in ascending order at the same time in one block display? What would that look like? Right now I have to call both methods seperatly in order to see both ascending methods in action and it doesn't look good...Also is it possible to separate the sorting logic from the main method and encapsulate it within a separate class or method that takes the ArrayList of students and the two comparators as arguments?... allowing the program to sort students by different criteria without duplicating the sorting logic? The attached image is what my program is displaying right now and I want it to display like: Student [roll number =1, name =Allan, address =620 pioneer st] Student [roll number =2, name =Billy, address =700 ash st] Student [roll number =3, name =Chris, address =801 cheney dr] Student [roll number =4, name =Diana, address =830 washington st] Student [roll number =5, name =Eliza, address =200 main st] Student [roll number =6, name =Fedrick, address =504 plymouth ave] Student [roll number =7, name =George, address =910 oak st] Student [roll number =8, name =Hannah, address =300 maple ave] Student [roll number =9, name =Julia, address =100 pine st] Student [roll number =10, name =Kevin, address =500 elm st] Source Code: package application; public class Student { introllNum; String name; String address; public Student(introllNum, String name, String address) { this.rollNum = rollNum; this.name = name; this.address = address; } //getters and setters public String getName() { returnname; } publicvoid setName(String name) { this.name = name; } publicint getRollNum() { returnrollNum; } publicvoid setRollNum(introllNum) { this.rollNum = rollNum; } public String getAddress() { returnaddress; } publicvoid setAddress(String address) { this.address = address; } @Override public String toString() { return"Student [roll number =" + rollNum + ", name =" + name + ", address =" + address + "]"; } } package application; import java.util.ArrayList; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.JTextArea; public class Main { publicstaticvoid main(String[] args) { ArrayList students = new ArrayList<>(); int[] rolls = {2,4,1,5,6,7,3,9,8,10}; String[] names = {"Allan", "Chris", "Fedrick", "Diana", "Eliza", "George", "Hannah", "Billy", "Julia", "Kevin"}; String[] addresses = {"620 pioneer st", "801 cheney dr", "504 plymouth ave", "830 washington st", "200 main st", "910 oak st", "300 maple ave", "700 ash st", "100 pine st", "500 elm st"}; for (inti = 0; i < 10; i++) { introllNum = rolls[i]; String name = names[i]; String address = addresses[i]; students.add(new Student(rollNum, name, address)); } // Create the GUI JFrame frame = new JFrame("Sorted Students"); JPanel panel = new JPanel(); JTextArea textArea = new JTextArea(15, 30); // 15 rows, 30 columns // Append the sorted student information to the text area StringBuilder string = new StringBuilder(); selectionSortByName(students); for (Student s : students) { string.append(s).append("\n"); } selectionSortByRollNum(students); for (Student s : students) { string.append(s).append("\n"); } textArea.setText(string.toString()); // Add the text area to the panel and the panel to the frame panel.add(textArea); frame.add(panel); // Set frame properties and display it frame.pack(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } publicstaticvoid selectionSortByName(ArrayList students) { StudentComparatorName name = new StudentComparatorName(); for (inti = 0; i < students.size(); i++) { intminIndex = i; for (intj = i; j < students.size(); j++) { //if the element at j is less than element at minIndex then update the minIndex if(name.compare(students.get(j), students.get(minIndex)) < 0){ minIndex = j; } } Student temp = students.get(i); //setting the element at minIndex to index i students.set(i,students.get(minIndex)); students.set(minIndex,temp); } } publicstaticvoid selectionSortByRollNum(ArrayList students) { StudentComparatorRollNum sortByRollNo = new StudentComparatorRollNum(); for (inti = 0; i < students.size(); i++) { intminIndex = i; for (intj = i; j < students.size(); j++) { if(sortByRollNo.compare(students.get(j), students.get(minIndex)) < 0){ minIndex = j; } } Student temp = students.get(i); students.set(i,students.get(minIndex)); students.set(minIndex,temp); } } } package application; import java.util.Comparator; public class StudentComparatorName implements Comparator { @Override publicint compare(Student student1, Student student2) { //using the string compareTo method to sort the students according to their name returnstudent1.getName().compareTo(student2.getName()); } } package application; import java.util.Comparator; public class StudentComparatorRollNum implements Comparator { @Override publicint compare(Student student1, Student student2) { if(student1.getRollNum() < student2.getRollNum()) return -1; elseif(student1.getRollNum() > student2.getRollNum()) return 1; else return 0; } }
Is there a way to simataniously get both student names' and roll numbers' to be in ascending order at the same time in one block display? What would that look like? Right now I have to call both methods seperatly in order to see both ascending methods in action and it doesn't look good...Also is it possible to separate the sorting logic from the main method and encapsulate it within a separate class or method that takes the ArrayList of students and the two comparators as arguments?... allowing the program to sort students by different criteria without duplicating the sorting logic?
The attached image is what my program is displaying right now and I want it to display like:
Student [roll number =1, name =Allan, address =620 pioneer st]
Student [roll number =2, name =Billy, address =700 ash st]
Student [roll number =3, name =Chris, address =801 cheney dr]
Student [roll number =4, name =Diana, address =830 washington st]
Student [roll number =5, name =Eliza, address =200 main st]
Student [roll number =6, name =Fedrick, address =504 plymouth ave]
Student [roll number =7, name =George, address =910 oak st]
Student [roll number =8, name =Hannah, address =300 maple ave]
Student [roll number =9, name =Julia, address =100 pine st]
Student [roll number =10, name =Kevin, address =500 elm st]
Source Code:
package application;
public class Student {
introllNum;
String name;
String address;
public Student(introllNum, String name, String address) {
this.rollNum = rollNum;
this.name = name;
this.address = address;
}
//getters and setters
public String getName() {
returnname;
}
publicvoid setName(String name) {
this.name = name;
}
publicint getRollNum() {
returnrollNum;
}
publicvoid setRollNum(introllNum) {
this.rollNum = rollNum;
}
public String getAddress() {
returnaddress;
}
publicvoid setAddress(String address) {
this.address = address;
}
@Override
public String toString()
{
return"Student [roll number =" + rollNum + ", name =" + name + ", address =" + address + "]";
}
}
package application;
import java.util.ArrayList;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextArea;
public class Main {
publicstaticvoid main(String[] args) {
ArrayList<Student> students = new ArrayList<>();
int[] rolls = {2,4,1,5,6,7,3,9,8,10};
String[] names = {"Allan", "Chris", "Fedrick", "Diana", "Eliza", "George", "Hannah", "Billy", "Julia", "Kevin"};
String[] addresses = {"620 pioneer st", "801 cheney dr", "504 plymouth ave", "830 washington st", "200 main st", "910 oak st", "300 maple ave", "700 ash st", "100 pine st", "500 elm st"};
for (inti = 0; i < 10; i++) {
introllNum = rolls[i];
String name = names[i];
String address = addresses[i];
students.add(new Student(rollNum, name, address));
}
// Create the GUI
JFrame frame = new JFrame("Sorted Students");
JPanel panel = new JPanel();
JTextArea textArea = new JTextArea(15, 30); // 15 rows, 30 columns
// Append the sorted student information to the text area
StringBuilder string = new StringBuilder();
selectionSortByName(students);
for (Student s : students) {
string.append(s).append("\n");
}
selectionSortByRollNum(students);
for (Student s : students) {
string.append(s).append("\n");
}
textArea.setText(string.toString());
// Add the text area to the panel and the panel to the frame
panel.add(textArea);
frame.add(panel);
// Set frame properties and display it
frame.pack();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
publicstaticvoid selectionSortByName(ArrayList<Student> students) {
StudentComparatorName name = new StudentComparatorName();
for (inti = 0; i < students.size(); i++) {
intminIndex = i;
for (intj = i; j < students.size(); j++) {
//if the element at j is less than element at minIndex then update the minIndex
if(name.compare(students.get(j), students.get(minIndex)) < 0){
minIndex = j;
}
}
Student temp = students.get(i);
//setting the element at minIndex to index i
students.set(i,students.get(minIndex));
students.set(minIndex,temp);
}
}
publicstaticvoid selectionSortByRollNum(ArrayList<Student> students) {
StudentComparatorRollNum sortByRollNo = new StudentComparatorRollNum();
for (inti = 0; i < students.size(); i++) {
intminIndex = i;
for (intj = i; j < students.size(); j++) {
if(sortByRollNo.compare(students.get(j), students.get(minIndex)) < 0){
minIndex = j;
}
}
Student temp = students.get(i);
students.set(i,students.get(minIndex));
students.set(minIndex,temp);
}
}
}
package application;
import java.util.Comparator;
public class StudentComparatorName implements Comparator<Student> {
@Override
publicint compare(Student student1, Student student2) {
//using the string compareTo method to sort the students according to their name
returnstudent1.getName().compareTo(student2.getName());
}
}
package application;
import java.util.Comparator;
public class StudentComparatorRollNum implements Comparator<Student> {
@Override
publicint compare(Student student1, Student student2) {
if(student1.getRollNum() < student2.getRollNum())
return -1;
elseif(student1.getRollNum() > student2.getRollNum())
return 1;
else
return 0;
}
}
![X
Sorted Students
Student [roll number =2, name=Allan, address = 620 pioneer st]
Student [roll number = 9, name =Billy, address =700 ash st]
Student [roll number =4, name =Chris, address =801 cheney dr]
Student [roll number =5, name=Diana, address =830 washington st]
Student [roll number =6, name Eliza, address = 200 main st]
Student [roll number =1, name =Fedrick, address =504 plymouth ave]
Student [roll number =7, name =George, address =910 oak st]
Student [roll number =3, name=Hannah, address =300 maple ave]
Student [roll number =8, name Julia, address = 100 pine st]
Student [roll number 10, name=Kevin, address =500 elm st]
Student [roll number=1, name =Fedrick, address =504 plymouth ave]
Student [roll number =2, name=Allan, address =620 pioneer st]
Student [roll number =3, name=Hannah, address =300 maple ave]
Student [roll number =4, name =Chris, address =801 cheney dr]
Student [roll number = 5, name =Diana, address =830 washington st]
Student [roll number =6, name Eliza, address=200 main st]
Student [roll number =7, name=George, address 910 oak st]
Student [roll number =8, name=Julia, address = 100 pine st]
Student [roll number =9, name=Billy, address =700 ash st]
Student [roll number = 10, name =Kevin, address =500 elm st]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6f16074c-c1d6-41d5-8a99-10feddcfb656%2Fa47776c8-a2be-4a1a-bdb3-6f1988443678%2Fv98alxl_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 1 images

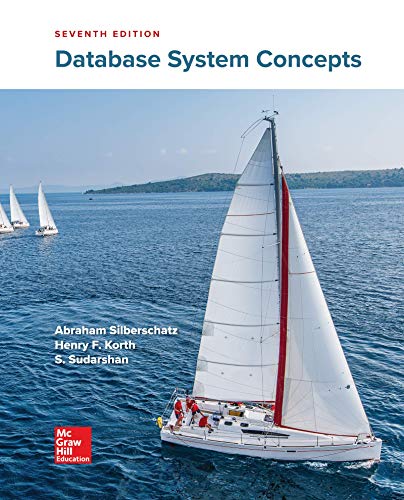
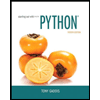
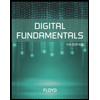
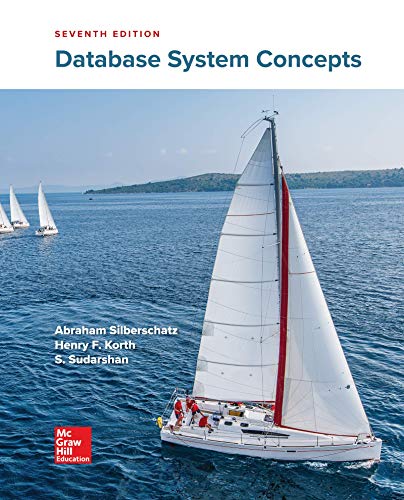
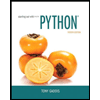
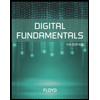
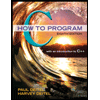
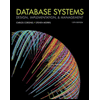
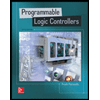