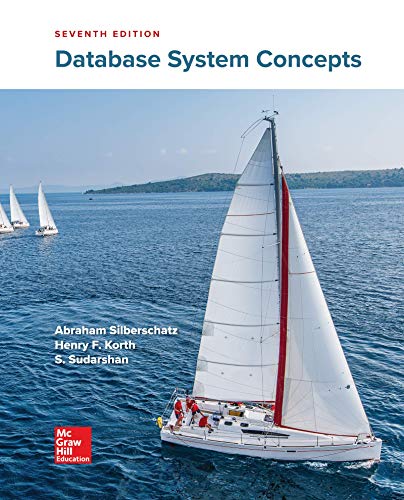
I need help with this Java program. I got some minor error that I couldn't fix.
Checker Classes
You will have to implement specific checks to highlight errors found in the source files. We provided an interface Check.java that defines a single method public Optional<Error> lint(String line, int lineNumber) . All the checkers you write should implement this interface and hence, you need to implement the lint method.
All of these should return an an Error when one is present with a custom message of your choosing to describe what the error means to the user. If the condition a Check is looking for is not present for a line, should return Optional.empty().
Other class
Error.java
public Error(int code, int lineNumber, String message)
Constructs an Error given the error code, line number and a message.
public String toString()
Returns a String representation of the error with the line number, error code and message. The representation should be formatted as (replace curly braces with the actual values)
public int getLineNumber()
Returns the line number of this error
public int getCode()
Returns the error code of this error
public String getMessage()
Returns the message for this error
Linter.java
public Linter(List<Check> checks)
Constructs a Linter given a list of checks to run through.
public List<Error> lint(String fileName) throws FileNotFoundException
Returns a list of all errors found.
All of these should return an an Error when one is present with a custom message of your choosing to describe what the error means to the user. If the condition a Check is looking for is not present for a line, should return Optional.empty().
BreakCheck class
import java.util.*;
public class BreakCheck implements Check {
public Optional<Error> lint(String line, int lineNumber) {
// Match "break" outside of comments or strings
if (line.matches("^(?!\\s*//|\\s*/\\*|\\s*\\*).*\\bbreak\\b.*")) {
return Optional.of(new Error(2, lineNumber, "Line contains 'break' keyword outside of a comment"));
}
return Optional.empty();
}
}
Error Class:
public class Error {
private final int code;
private final int lineNumber;
private final String message;
public Error(int code, int lineNumber, String message) {
this.code = code;
this.lineNumber = lineNumber;
this.message = message;
}
public String toString() {
return String.format("(Line: %d) has error code %d\n%s", lineNumber, code, message);
}
public int getLineNumber() {
return lineNumber;
}
public int getCode() {
return code;
}
public String getMessage() {
return message;
}
}
TestFile:
public class TestFile {
public static void main(String[] args) {
System.out.println("This is a really really really long line that should fail the long line check");
while (true) {
System.out.println("");
break;
}
}
}
Linter Class
import java.util.*;
import java.io.*;
public class Linter {
private final List<Check> checks;
public Linter(List<Check> checks) {
this.checks = checks;
}
public List<Error> lint(String fileName) throws FileNotFoundException {
List<Error> errors = new ArrayList<>();
File file = new File(fileName);
Scanner scanner = new Scanner(file);
int lineNumber = 0;
while (scanner.hasNextLine()) {
lineNumber++;
String line = scanner.nextLine();
for (Check check : checks) {
Optional<Error> result = check.lint(line, lineNumber);
if (result.isPresent()) {
errors.add(result.get());
}
}
}
scanner.close();
return errors;
}
}
LinterMain :
import java.util.*;
import java.io.*;
public class LinterMain {
public static final String FILE_NAME = "TestFile.java";
public static void main(String[] args) throws FileNotFoundException{
List<Check> checks = new ArrayList<>();
checks.add(new LongLineCheck());
checks.add(new BlankPrintlnCheck());
checks.add(new BreakCheck());
Linter linter = new Linter(checks);
List<Error> errors = linter.lint(FILE_NAME);
for (Error e : errors) {
System.out.println(e);
}
}
}
Check.java
import java.util.*;public interface Check {
public Optional<Error> lint(String line, int lineNumber);
}
I can't pass this check :
BreakCheck correctly tests for break statements:
Failed: Make sure that breakCheck does not only check if a line starts with a comment for the case when 'break' is in a comment. ==> expected: <true> but was: <false>
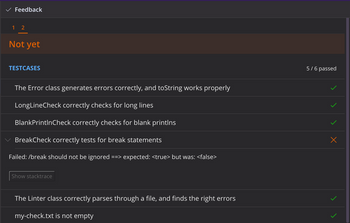
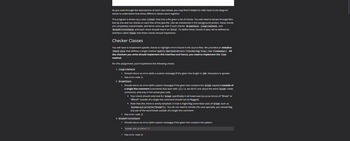

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- please write code in java Create a right triangle class with instance variables(double) for sideA, sideB and the hypo. write three constructors -the 2-param version assigns to sides A and B respectively. the 1-param version assigns the same value to both sides. the 0-param version assigns 10.0 to each side. the constructor also computes the hypotenuse based on the other two sides and saves that in the hypotenuse variable. Create accessors for all three variables. Write a toString() that will print/label the three sides. write some main code to create three instances of your class, one to test each of your constructors. you can input values or use fixed values. Display each instance using an implicit toString() call.arrow_forwardWrite a java code for this. Strings Read the paragraph and encrypt the content using the following rules; "The String, StringBuffer, and StringBuilder classes are defined in java.lang. Thus, they are available to all programs automatically. All are declared final, which means that none of these classes may be subclassed. This allows certain optimizations that increase performance to take place on common string operations. All three implement the CharSequence interface." RULES The words ‘the’, ‘and’, and ‘for’ should be replaced by their reverse strings in upper case letters (use predefined functions reverse() and toupper()) The first occurrence of the word ‘are’ should be replaced by ‘AREERA’ and the last occurrence of the word ‘of’ to be replaced by ‘123’.arrow_forwardplease answer with outputarrow_forward
- Below for each class you find a UML and description of the public interface. Implementing the public interface as described is madatory. There's freedom on how to implement these classes.The private properties and private methods are under your control.. There are multiple ways of implementing all these classes. Feel free to add private properties and methods. For each object, it's mandatory to create a header file (.h), implementation file (.cpp) and a driver. Blank files are included. The header should have the class definition in it. The implementation file should contain the implementations of the methods laid out in the header fine. And finally the Driver should test/demonstrate all the features of the class. It's best to develop the driver as the class is being written. Check each section to see if there are added additional requirements for the driver. Two test suites are included so that work can be checked. It's important to implement the drivers to test and demonstrate…arrow_forwardBelow for each class you find a UML and description of the public interface. Implementing the public interface as described is madatory. There's freedom on how to implement these classes.The private properties and private methods are under your control.. There are multiple ways of implementing all these classes. Feel free to add private properties and methods. For each object, it's mandatory to create a header file (.h), implementation file (.cpp) and a driver. Blank files are included. The header should have the class definition in it. The implementation file should contain the implementations of the methods laid out in the header fine. And finally the Driver should test/demonstrate all the features of the class. It's best to develop the driver as the class is being written. Check each section to see if there are added additional requirements for the driver. Two test suites are included so that work can be checked. It's important to implement the drivers to test and demonstrate…arrow_forwardWrite a java class method named capitalizeWords that takes one parameter of type String. The method should print its parameter, but with the first letter of each word capitalized. For example, the call capitalizeWords ("abstract window toolkit"); will produce the following output: Abstract Window Toolkitarrow_forward
- Can I get a help with this in Java please? Introduce a new class, called Borrower to the project. Its purpose is to represent the borrower of the CD. It should have two fields surname and libraryId; where the latter is a mix of letters and numbers, and a suitable constructor with parameters for only these two fields in the order specified above as well as appropriate accessor methods.arrow_forwardJava. Please use the template in the picture.arrow_forwardIn Java, please. Complete the Course class by implementing the findStudentHighestGpa() method, which returns the Student object with the highest GPA in the course. Assume that no two students have the same highest GPA. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. (Hint: getGPA() returns a student's GPA.) Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Nguyen 3.5 Brenda Stern 2.0 Lynda Robison 3.2 Sonya King 3.9 the output is: Top student: Sonya King (GPA: 3.9) LabProgram.java import java.util.Scanner; public class LabProgram { public static void main(String args[]) { Scanner scnr = new Scanner(System.in); Course course = new Course(); int…arrow_forward
- Implement the following in the .NET Console App. Write the Bus class. A Bus has a length, a color, and a number of wheels. a. Implement data fields using auto-implemented Properies b. Include 3 constructors: default, the one that receives the Bus length, color and a number of wheels as input (utilize the Properties) and the Constructor that takes the Bus number (an integer) as input.arrow_forwardUsing the Card.java class file, write a program to simulate a Deck of Cards. See Programming Project 8.7 (PP 8.7) from page 403 of your textbook (or view the attached image) for a description of what your program needs to do. Note that although the book description of the problem states that you should write the Card class, I do not want you to do that. You must use the Card file exactly as it is provided (NO modifications) and only write the DeckOfCards and Driver classes. public class Card{public final static int ACE = 1;public final static int TWO = 2;public final static int THREE = 3;public final static int FOUR = 4;public final static int FIVE = 5;public final static int SIX = 6;public final static int SEVEN = 7;public final static int EIGHT = 8;public final static int NINE = 9;public final static int TEN = 10;public final static int JACK = 11;public final static int QUEEN = 12;public final static int KING = 13; public final static int CLUBS = 1;public final static int DIAMONDS =…arrow_forwardUse Java program to add a method printReceipt to the CashRegister class. The method should print the prices of all purchased items and the total amount due. Hint: You will need to form a string of all prices. Use the concat method of the String class to add additional items to that string. To turn a price into a string, use the call String.valueOf(price). Also, the payment information will need to be the same as the price information. Here is the CashRegister class: /** A cash register totals up sales and computes change due.*/public class CashRegister{ private double purchase; private double payment; /** Constructs a cash register with no money in it. */ public CashRegister() { purchase = 0; payment = 0; } /** Records the sale of an item. @param amount the price of the item */ public void recordPurchase(double amount) { purchase = purchase + amount; } /** Processes a payment received from the customer. @param…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
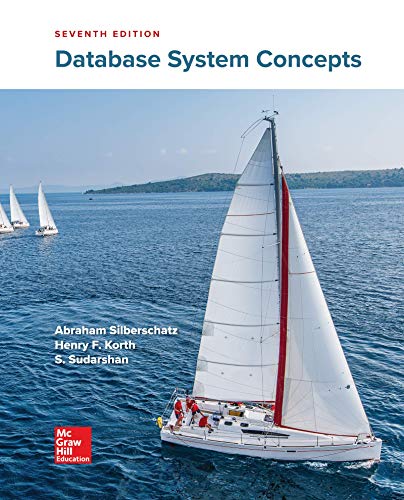
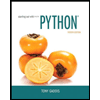
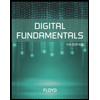
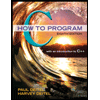
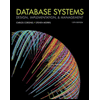
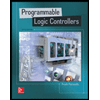