Add a toString() method to Fraction class that returns the fraction as a String in the form "x / y", where x and y are numerator and denominator respectively. As the method does not do any display itself, the output can be done by a client program that calls the method in an output statement. Use client program to test this functionality; i.e. provide an output statement to display a fraction as its String representation. class Fraction2 { private int n, d; public Fraction() { this.n = this.d = 0; //Initialize the values } public Fraction(int n, int d) { this.n = n; //Initialize the variables this.d = d; } //Define the getter function getNum() that returns the numerator public int getNum() { //Returns numerator return n; } //Define the getter function getDen() that returns the denominator public int getDen() { //Returns denominator return d; } //Define the boolean function isZero() that returns 0 if numerator is 0 and denominator is not equals to zero public boolean isZero() { return(getNum() == 0 && getDen() != 0); } public boolean isequals(Object c) { Fraction f = (Fraction) c; return(Integer.compare(n,f.n))==0 && (Integer.compare(d,f.d)==0); } //Define the function getSimplifiedFraction() that returns the simplified fraction public String getSimplifiedFraction() { //Decalre the string variable result to store the result String result = ""; //if the numerator and denominator both are zero if(getNum() == 0 && getDen() == 0) //result is zero result = "0"; //if the numerator is zero and denominator is non-zero else if(isZero()) //result is zero result = "0"; //if the numerator is non-zero and denominator is zero else if(getNum() != 0 && getDen() == 0) //result is Undefined result = "Undefined"; //for a defined and non-zero fraction else { //if the remainder is zero if(getNum() % getDen() == 0) result = (getNum() / getDen()) + ""; //if the numerator and denominator both are greater than zero else if(getNum() < 0 && getDen() < 0) result = (getNum() * -1) + "/" + (getDen() * -1); //if any of them is zero else if(getNum() < 0 || getDen() < 0) { //if the numerator is greater than zero if(getNum() < 0) result = "-" + (getNum() * -1) + "/" + getDen(); // appending a sign. //if the denominator is zero else result = "-" + getNum() + "/" + (getDen() * -1); // appending a sign. } //both are non-zero else result = (getNum() + "/" + getDen()); } //return the result return result; } //Define the method display() that displays the resultant fraction public void display() { //Call and display the fraction System.out.println(getSimplifiedFraction()); } public Fraction add(Fraction rhs) { Fraction sum = new Fraction(); sum.d = d * rhs.d; sum.n = n * rhs.d + d * rhs.n; sum.simplify(); return sum; } private void simplify() { int a,b; if(Math.abs(n) > Math.abs(d)) { a = Math.abs(n); b = Math.abs(d); } else { a = Math.abs(d); b = Math.abs(n); } int r = a%b; while(r != 0) { a = b; b = r; r = a%b; } //divide numerator and denominator by b n /= b; d /= b; }
Add a toString() method to Fraction class that returns the fraction as a
String in the form "x / y", where x and y are numerator and denominator
respectively. As the method does not do any display itself, the output can be done by a client program that calls the method in an output statement. Use client program to test this functionality; i.e. provide an output statement to display a fraction as its String representation.
class Fraction2
{
private int n, d;
public Fraction()
{
this.n = this.d = 0; //Initialize the values
}
public Fraction(int n, int d)
{
this.n = n; //Initialize the variables
this.d = d;
}
//Define the getter function getNum() that returns the numerator
public int getNum()
{
//Returns numerator
return n;
}
//Define the getter function getDen() that returns the denominator
public int getDen()
{
//Returns denominator
return d;
}
//Define the boolean function isZero() that returns 0 if numerator is 0 and denominator is not equals to zero
public boolean isZero()
{
return(getNum() == 0 && getDen() != 0);
}
public boolean isequals(Object c)
{
Fraction f = (Fraction) c;
return(Integer.compare(n,f.n))==0 && (Integer.compare(d,f.d)==0);
}
//Define the function getSimplifiedFraction() that returns the simplified fraction
public String getSimplifiedFraction()
{
//Decalre the string variable result to store the result
String result = "";
//if the numerator and denominator both are zero
if(getNum() == 0 && getDen() == 0)
//result is zero
result = "0";
//if the numerator is zero and denominator is non-zero
else if(isZero())
//result is zero
result = "0";
//if the numerator is non-zero and denominator is zero
else if(getNum() != 0 && getDen() == 0)
//result is Undefined
result = "Undefined";
//for a defined and non-zero fraction
else
{
//if the remainder is zero
if(getNum() % getDen() == 0)
result = (getNum() / getDen()) + "";
//if the numerator and denominator both are greater than zero
else if(getNum() < 0 && getDen() < 0)
result = (getNum() * -1) + "/" + (getDen() * -1);
//if any of them is zero
else if(getNum() < 0 || getDen() < 0)
{
//if the numerator is greater than zero
if(getNum() < 0)
result = "-" + (getNum() * -1) + "/" + getDen(); // appending a sign.
//if the denominator is zero
else
result = "-" + getNum() + "/" + (getDen() * -1); // appending a sign.
}
//both are non-zero
else
result = (getNum() + "/" + getDen());
}
//return the result
return result;
}
//Define the method display() that displays the resultant fraction
public void display()
{
//Call and display the fraction
System.out.println(getSimplifiedFraction());
}
public Fraction add(Fraction rhs) {
Fraction sum = new Fraction();
sum.d = d * rhs.d;
sum.n = n * rhs.d + d * rhs.n;
sum.simplify();
return sum;
}
private void simplify()
{
int a,b;
if(Math.abs(n) > Math.abs(d))
{
a = Math.abs(n);
b = Math.abs(d);
}
else
{
a = Math.abs(d);
b = Math.abs(n);
}
int r = a%b;
while(r != 0)
{
a = b;
b = r;
r = a%b;
}
//divide numerator and denominator by b
n /= b;
d /= b;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

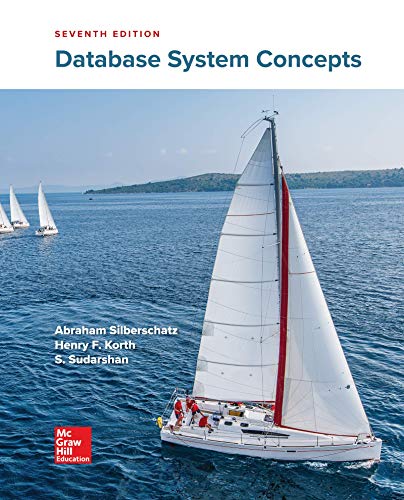
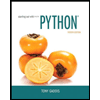
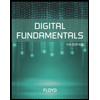
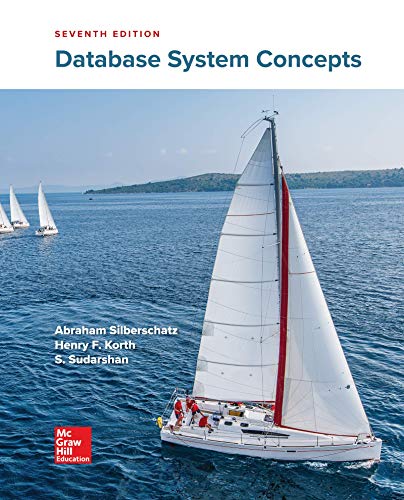
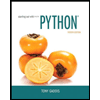
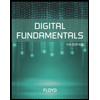
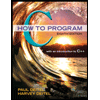
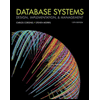
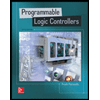