IntSet unionWith(const IntSet& otherIntSet) const // Pre: size() + (otherIntSet.subtract(*this)).size() <= MAX_SIZE // Post: An IntSet representing the union of the invoking IntSet // and otherIntSet is returned. // Note: Equivalently (see postcondition of add), the IntSet // returned is one that initially is an exact copy of the // invoking IntSet but subsequently has all elements of // otherIntSet added. // IntSet intersect(const IntSet& otherIntSet) const // Pre: (none) // Post: An IntSet representing the intersection of the invoking // IntSet and otherIntSet is returned. // Note: Equivalently (see postcondition of remove), the IntSet // returned is one that initially is an exact copy of the // invoking IntSet but subsequently has all of its elements // that are not also elements of otherIntSet removed. // IntSet subtract(const IntSet& otherIntSet) const // Pre: (none) // Post: An IntSet representing the difference between the invoking // IntSet and otherIntSet is returned. // Note: Equivalently (see postcondition of remove), the IntSet // returned is one that initially is an exact copy of the // invoking IntSet but subsequently has all elements of // otherIntSet removed. IntSet unionWith(const IntSet& otherIntSet) const; IntSet intersect(const IntSet& otherIntSet) const; IntSet subtract(const IntSet& otherIntSet) const;
IntSet unionWith(const IntSet& otherIntSet) const
// Pre: size() + (otherIntSet.subtract(*this)).size() <= MAX_SIZE
// Post: An IntSet representing the union of the invoking IntSet
// and otherIntSet is returned.
// Note: Equivalently (see postcondition of add), the IntSet
// returned is one that initially is an exact copy of the
// invoking IntSet but subsequently has all elements of
// otherIntSet added.
// IntSet intersect(const IntSet& otherIntSet) const
// Pre: (none)
// Post: An IntSet representing the intersection of the invoking
// IntSet and otherIntSet is returned.
// Note: Equivalently (see postcondition of remove), the IntSet
// returned is one that initially is an exact copy of the
// invoking IntSet but subsequently has all of its elements
// that are not also elements of otherIntSet removed.
// IntSet subtract(const IntSet& otherIntSet) const
// Pre: (none)
// Post: An IntSet representing the difference between the invoking
// IntSet and otherIntSet is returned.
// Note: Equivalently (see postcondition of remove), the IntSet
// returned is one that initially is an exact copy of the
// invoking IntSet but subsequently has all elements of
// otherIntSet removed.
IntSet unionWith(const IntSet& otherIntSet) const;
IntSet intersect(const IntSet& otherIntSet) const;
IntSet subtract(const IntSet& otherIntSet) const;

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

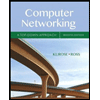
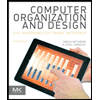
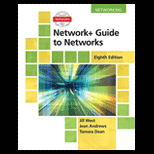
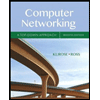
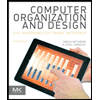
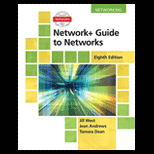
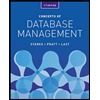
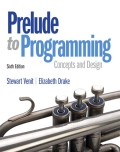
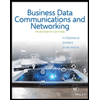