In this lab, you use the flowchart and pseudocode found in the figure below to add code to a partially created Java program. When completed, college admissions officers should be able to use the Java program to determine whether to accept or reject a student, based on his or her test score and class rank. start input testScore, classRank if testScore >= 90 then if classRank >= 25 then output "Accept" else output "Reject" end if else if testScore >= 80 then if classRank >= 50 then output "Accept" else output "Reject " endif else if testScore >= 70 then if classRank >= 75 then output "Accept" else output "Reject" end if else out put "Reject" end if end if end if stop Instructions Study the pseudocode in picture above. Declare the variables testScoreString and classRankString. Write the interactive input statements to retrieve: A student’s test score (testScoreString) A student's class rank (classRankString) Write the statements to convert the string representation of a student’s test score and class rank to the integer data type (testScore and classRank, respectively). The rest of the program is written for you. Execute the program by clicking "Run Code." Enter 87 for the test score and 60 for the class rank. Execute the program by entering 60 for the test score and 87 for the class rank. * Program Name: CollegeAdmission.java Function: This program determines if a student will be admitted or rejected. Input: Interactive Output: Accept or Reject */ import java.util.Scanner; public class CollegeAdmission { public static void main(String args[]) { Scanner s = new Scanner(System.in); // Declare variables // Get input and convert to correct data type // Test using admission requirements and print Accept or Reject if( testScore >= 90 ) { if( classRank >= 25) { System.out.println("Accept"); } else System.out.println("Reject"); } else { if( testScore >= 80 ) { if( classRank >= 50 ) System.out.println("Accept"); else System.out.println("Reject"); } else { if( testScore >= 70 ) { if( classRank >=75 ) System.out.println("Accept"); else System.out.println("Reject"); } else System.out.println("Reject"); } } } // End of main() method } // End of CollegeAdmission class
In this lab, you use the flowchart and pseudocode found in the figure below to add code to a partially created Java program. When completed, college admissions officers should be able to use the Java program to determine whether to accept or reject a student, based on his or her test score and class rank. start input testScore, classRank if testScore >= 90 then if classRank >= 25 then output "Accept" else output "Reject" end if else if testScore >= 80 then if classRank >= 50 then output "Accept" else output "Reject " endif else if testScore >= 70 then if classRank >= 75 then output "Accept" else output "Reject" end if else out put "Reject" end if end if end if stop Instructions Study the pseudocode in picture above. Declare the variables testScoreString and classRankString. Write the interactive input statements to retrieve: A student’s test score (testScoreString) A student's class rank (classRankString) Write the statements to convert the string representation of a student’s test score and class rank to the integer data type (testScore and classRank, respectively). The rest of the program is written for you. Execute the program by clicking "Run Code." Enter 87 for the test score and 60 for the class rank. Execute the program by entering 60 for the test score and 87 for the class rank. * Program Name: CollegeAdmission.java Function: This program determines if a student will be admitted or rejected. Input: Interactive Output: Accept or Reject */ import java.util.Scanner; public class CollegeAdmission { public static void main(String args[]) { Scanner s = new Scanner(System.in); // Declare variables // Get input and convert to correct data type // Test using admission requirements and print Accept or Reject if( testScore >= 90 ) { if( classRank >= 25) { System.out.println("Accept"); } else System.out.println("Reject"); } else { if( testScore >= 80 ) { if( classRank >= 50 ) System.out.println("Accept"); else System.out.println("Reject"); } else { if( testScore >= 70 ) { if( classRank >=75 ) System.out.println("Accept"); else System.out.println("Reject"); } else System.out.println("Reject"); } } } // End of main() method } // End of CollegeAdmission class
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In this lab, you use the flowchart and pseudocode found in the figure below to add code to a partially created Java program. When completed, college admissions officers should be able to use the Java program to determine whether to accept or reject a student, based on his or her test score and class rank.
start input testScore,
classRank
if testScore >= 90
then if classRank >= 25
then output "Accept"
else output "Reject"
end
if else if testScore >= 80
then if classRank >= 50
then output "Accept"
else output "Reject
" endif else if testScore >= 70
then if classRank >= 75
then output "Accept"
else output "Reject"
end if else out put "Reject" end if end if end if stop
Instructions
- Study the pseudocode in picture above.
- Declare the variables testScoreString and classRankString.
- Write the interactive input statements to retrieve:
- A student’s test score (testScoreString)
- A student's class rank (classRankString)
- Write the statements to convert the string representation of a student’s test score and class rank to the integer data type (testScore and classRank, respectively).
- The rest of the program is written for you.
- Execute the program by clicking "Run Code." Enter 87 for the test score and 60 for the class rank.
- Execute the program by entering 60 for the test score and 87 for the class rank.
* Program Name: CollegeAdmission.java
Function: This program determines if a student will be admitted or rejected.
Input: Interactive
Output: Accept or Reject
*/
import java.util.Scanner;
public class CollegeAdmission
{
public static void main(String args[])
{
Scanner s = new Scanner(System.in);
// Declare variables
// Get input and convert to correct data type
// Test using admission requirements and print Accept or Reject
if( testScore >= 90 )
{
if( classRank >= 25)
{
System.out.println("Accept");
}
else
System.out.println("Reject");
}
else
{
if( testScore >= 80 )
{
if( classRank >= 50 )
System.out.println("Accept");
else
System.out.println("Reject");
}
else
{
if( testScore >= 70 )
{
if( classRank >=75 )
System.out.println("Accept");
else
System.out.println("Reject");
}
else
System.out.println("Reject");
}
}
} // End of main() method
} // End of CollegeAdmission class
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
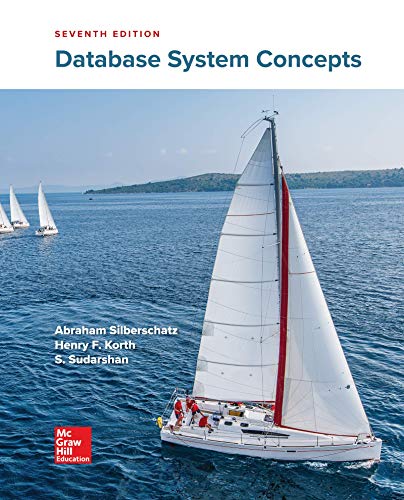
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
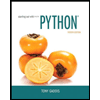
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
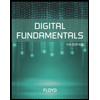
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
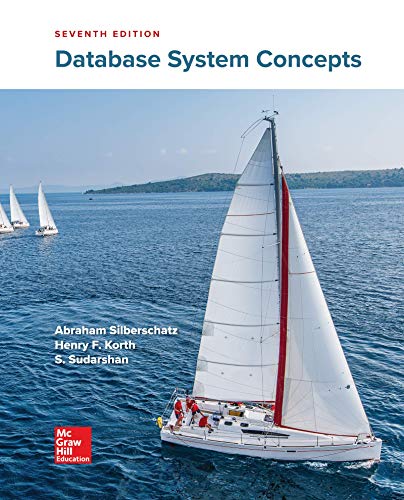
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
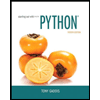
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
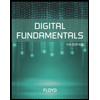
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
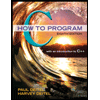
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
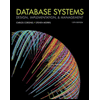
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
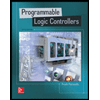
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education