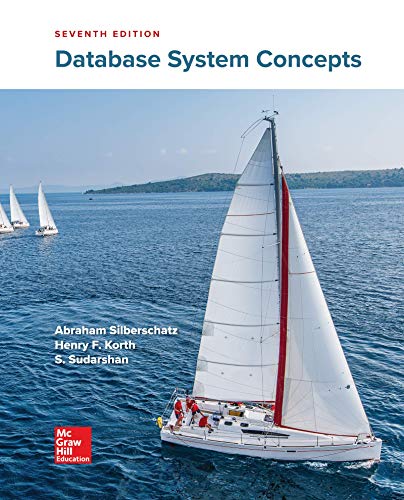
(IN JAVA)
Run the following
public class FinalProgramTwo {
public static void main(String args[]) {
int n;
Scanner sc =new Scanner(System.in);
System.out.println("Enter the Size of 1st array:");
n=sc.nextInt();// size of 1st array
String a[] = new String[n];
System.out.println("Enter the element of 1st array:");
for(int i=0;i<n;i++){
a[i]=sc.next();
}
System.out.println("Enter the size of 2nd array");
int m =sc.nextInt(); // size of 2nd array
System.out.println("Enter the element of 2nd array:");
String b[] = new String[m];
for(int i=0;i<m;i++){
b[i]=sc.next();
}
String d[] = new String[m+n];
d= mergeUniqueValues(a,b);
int size =m+n;
for( int i=0;i<d.length;i++){
System.out.println(d[i]);
}
}
private static String[] mergeUniqueValues(String[] a, String[] b) {
int size=a.length+b.length;
String c[] = new String[size];
int i;
int j;
int pos=0;
for(i=0;i<a.length;i++){
c[pos++]=a[i];
}
for( j=0;j<b.length;j++){
c[pos++]=b[j];
}
Arrays.sort(c);
LinkedHashSet<String> remove_dublicate =
new LinkedHashSet<String>(Arrays.asList(c)); // converting array to list
String[] newArray = remove_dublicate.toArray(new String[ remove_dublicate.size() ]); // converting list to array
return newArray;
}
}

Step by stepSolved in 2 steps with 2 images

- (Java) Q3 explain the answers to the below questions using step-by-step explanation. Fill in the missing line of code for the insert method for an array: public static void insert(String array[], int numElements, int indexToInsert, String newValue) {if (array.length == numElements) {System.out.println("Array is full. No room to insert.");return;}for (int i = numElements; i > indexToInsert; i--) {//fill in the missing line of code here}array[indexToInsert] = newValue;}arrow_forward**JAVA DEBUGGING** The file provided in the code editor to the right contains syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. // Displays five random numbers between // (and including) user-specified values import java.util.Scanner; public class DebugSix4 { public static void main(String[] args) { int high, low, count = 0; final int NUM = 5; Scanner input = new Scanner(System.in); System.out.print("This application displays " + NUM + " random numbers" + "\nbetween the low and high values you enter" + "\nEnter low value now... "); low = input.nextInt() System.out.print("Enter high value... "); high = inputnextInt(); while(low < high) { System.out.println("The number you entered for a high number, " + high + ", is not more than " + low);…arrow_forward1) Complete the assignment in Joodle/Repl.it or an IDE of your choice. 2) A) asks how many grades are to be entered and then creates an array of that size. Recall when getting user input from the keyboard to: import java.util.Scanner; Also, declare a scanner object. For instance: Scanner scanner = new Scanner(System.in); B) The program should then ask the user to enter the grades and assigns it into the array. C) Next, transverse the array, calculate and print the average. Round the average to the nearest tenth. System.out.format("Array Avg: %.1f", average); D) It should then counts the number of passing grades, the number of failing grades, and the number of Bogus grades. A bogus grade is considered: (>100 or < 0) and print the results. E) Write a method cleanArray() that takes the array as a parameter and removes any negative numbers or numbers greater than 100. (Bogus grades) F) Finally, display the average of all the valid grades, and all of the counters. Round the average to the…arrow_forward
- (Plot the cube function) Write a program that draws a diagram for the functionf(x) = x3 (see Figure ).Hint: Add points to a polyline using the following code:Polyline polyline = new Polyline();ObservableList<Double> list = polyline.getPoints();double scaleFactor = 0.0125;for (int x = –100; x <= 100; x++) {list.add(x + 200.0);list.add(scaleFactor * x * x * x);}arrow_forwardJAVA Language: Transpose Rotate. Question: Modify Transpose.encode() so that it uses a rotation instead of a reversal. That is, a word like “hello” should be encoded as “ohell” with a rotation of one character. (Hint: use a loop to append the letters into a new string) import java.util.*; public class TestTranspose { public static void main(String[] args) { String plain = "this is the secret message"; // Here's the message Transpose transpose = new Transpose(); String secret = transpose.encrypt(plain); System.out.println("\n ********* Transpose Cipher Encryption *********"); System.out.println("PlainText: " + plain); // Display the results System.out.println("Encrypted: " + secret); System.out.println("Decrypted: " + transpose.decrypt(secret));// Decrypt } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new…arrow_forwardIdentify the errors in the following code segment * provide the correction for each error. Import Java.util.scanner; public class Readable { public Static void main(String[] args) { Scanner KB ; int shares; double averagePrice = 14.67; Shares = KB.nextDouble(); System.out.println("There were " , shares + " shares sold at $" + averagePrice + " per share."); } }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
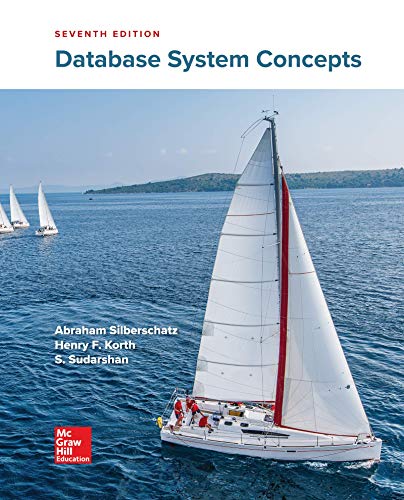
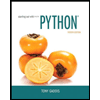
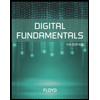
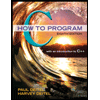
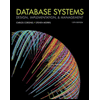
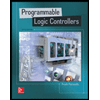