(In java please) ( NO TOY CLASS PLEASE READ INSTRUCTION OR DOWN VOTE PLEASE LOOK AT TWO IMAGES BELOW FIRST ONE IS CURRENCY CLASS NEED THE SECOND IS INSTRUCTIONS ON HOW TO MAKE TEST CLASS. PLEASE NO TOY CLASS OR DOWN VOTE USE CURRENCY CLASS INSTEAD AND UPDATE IT) THIS A LinkNode structure or class which will have two attributes - a data attribute, and a pointer attribute to the next node. The data attribute of the LinkNode should be a reference/pointer of the Currency class of Lab 2. Do not make it an inner class or member structure to the SinglyLinkedList class of #2 below. A SinglyLinkedList class which will be composed of three attributes - a count attribute, a LinkNode pointer/reference attribute named as and pointing to the start of the list and a LinkNode pointer/reference attribute named as and pointing to the end of the list. Since this is a class, make sure
(In java please) ( NO TOY CLASS PLEASE READ INSTRUCTION OR DOWN VOTE PLEASE LOOK AT TWO IMAGES BELOW FIRST ONE IS CURRENCY CLASS NEED THE SECOND IS INSTRUCTIONS ON HOW TO MAKE TEST CLASS. PLEASE NO TOY CLASS OR DOWN VOTE USE CURRENCY CLASS INSTEAD AND UPDATE IT) THIS A LinkNode structure or class which will have two attributes - a data attribute, and a pointer attribute to the next node. The data attribute of the LinkNode should be a reference/pointer of the Currency class of Lab 2. Do not make it an inner class or member structure to the SinglyLinkedList class of #2 below. A SinglyLinkedList class which will be composed of three attributes - a count attribute, a LinkNode pointer/reference attribute named as and pointing to the start of the list and a LinkNode pointer/reference attribute named as and pointing to the end of the list. Since this is a class, make sure all these attributes are private. The class and attribute names for the node and linked list are the words in bold in #1 and #2. For the Linked List, implement the following linked-list behaviors as explained in class - getters/setters/constructors/destructors, as needed, for the attributes of the class. createList method in addition to the constructor - this is optional for overloading purposes. destroyList method in place of or in addition to the destructor - this is optional for overloading purposes, addCurrency method which takes a Currency object and a node index value as parameters to add the Currency to the list at that index. removeCurrency method which takes a Currency object as parameter and removes that Currency object from the list and may return a copy of the Currency. removeCurrency overload method which takes a node index as parameter and removes the Currency object at that index and may return a copy of the Currency. findCurrency method which takes a Currency object as parameter and returns the node index at which the Currency is found in the list. getCurrency method which takes an index values as a parameter and returns the Currency object. printList method which returns a string of all the Currency objects in the list in the order of index, tab spaced. isListEmpty method which returns if a list is empty or not. countCurrency method which returns a count of Currency nodes in the list. Any other methods you think would be useful in your program. A Stack class derived from the SinglyLinkedList but with no additional attributes and the usual stack methods - constructor and createStack (optional) methods, push which takes a Currency object as parameter and adds it to the top of the stack. pop which takes no parameter and removes and returns the Currency object from the top of the stack. peek which takes no parameter and returns a copy of the Currency object at the top of the stack. printStack method which returns a string signifying the contents of the stack from the top to bottom, tab spaced. destructor and/or destroyStack (optional) methods. Ensure that the Stack objects do not allow Linked List functions to be used on them. A Queue class derived from the SinglyLinkedList but with no additional attributes and the usual queue methods - constructor and createQueue (optional) methods, enqueue which takes a Currency object as parameter and adds it to the end of the queue. dequeue which takes no parameter and removes and returns the Currency object from the front of the queue. peekFront which takes no parameter and returns a copy of the Currency object at the front of the queue. peekRear which takes no parameter and returns a copy of the Currency object at the end of the queue. printQueue method which returns a string signifying the contents of the queue from front to end, tab spaced. destructor and/or destroyQueue (optional) methods. Ensure that the Queue objects do not allow Linked List functions to be used on them. Ensure that all your classes are mimimal and cohesive with adequate walls around them. Make sure to reuse and not duplicate any code. Then a main driver program that will demonstrate all the capabilities of your ADTs as follows - First, print a Welcome message for the demonstration of your ADTs - you can decide what the message says but it should include your full name(s). Second, create the following twenty (20) Dollar objects in a Currency array to be used in the program. $57.12 $23.44 $87.43 $68.99 $111.22 $44.55 $77.77 $18.36 $543.21 $20.21 $345.67 $36.18 $48.48 $101.00 $11.00 $21.00 $51.00 $1.00 $251.00 $151.00



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

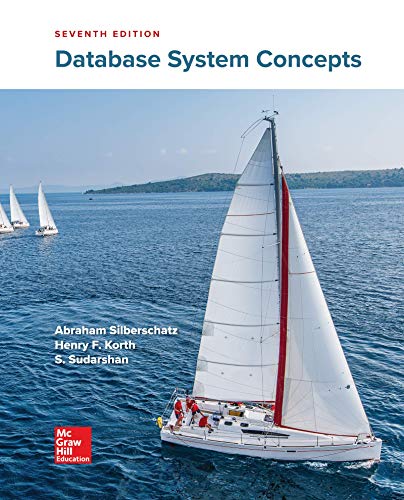
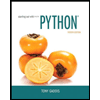
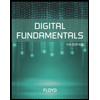
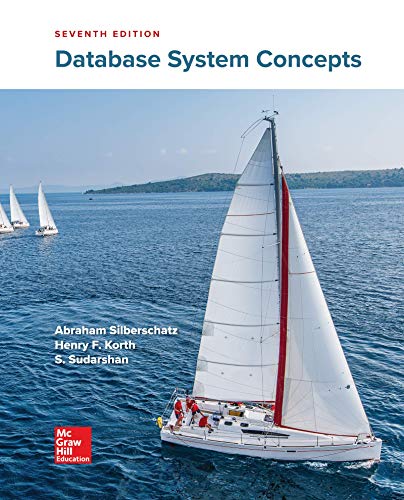
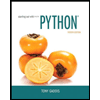
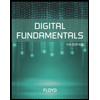
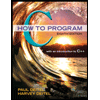
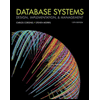
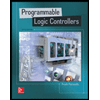